最近学着使用Mybatis和SpringBoot进行整合,涉及到多种数据类型时,要分别写入每个映射类,觉得过于繁琐。寻思着想了个简单的方式,利用map完成,具体如何实现呢。
下面给大家分享个简单的例子,我自认为复杂的可以借鉴。
<select id="getDeptMap" parameterType="pd" resultType="pd">
select d.deptno,d.dname from dept d
<where>
1 = 1
<if test="deptno != null and deptno != '' ">
and d.deptno = #{deptno}
</if>
</where>
</select>
mybatis的配置项
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!-- 进行Mybatis的相应的环境的属性定义 -->
<settings> <!-- 在本项目之中开启二级缓存 -->
<setting name="cacheEnabled" value="true"/>
</settings>
<!-- 实体映射 -->
<typeAliases>
<typeAlias type="cn.linkpower.util.PageData" alias="pd"/>
</typeAliases>
</configuration>
springboot数据库连接池以及配置项导入的配置 application.yml
server:
port: 80
mybatis:
config-location: classpath:mybatis/mybatis.cfg.xml # mybatis配置文件所在路径
type-aliases-package: cn.linkpower.vo # 定义所有操作类的别名所在包
mapper-locations: # 所有的mapper映射文件
- classpath:mybatis/mapper/**/*.xml
spring:
datasource:
type: com.alibaba.druid.pool.DruidDataSource # 配置当前要使用的数据源的操作类型
driver-class-name: org.gjt.mm.mysql.Driver # 配置MySQL的驱动程序类
url: jdbc:mysql://127.0.0.1:3306/javademo # 数据库连接地址
username: root # 数据库用户名
password: root # 数据库连接密码
filters: stat,wall,log4j # 开启druid监控还需要进行一项过滤配置
dbcp2: # 进行数据库连接池的配置
min-idle: 5 # 数据库连接池的最小维持连接数
initial-size: 5 # 初始化提供的连接数
max-total: 10 # 最大的连接数
max-wait-millis: 200 # 等待连接获取的最大超时时间
下面的这个工具类才是重点
package cn.linkpower.util;
import java.io.BufferedReader;
import java.io.Reader;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
import javax.servlet.http.HttpServletRequest;
import com.alibaba.druid.proxy.jdbc.ClobProxyImpl;
/**
* 参数封装Map
* @author 香蕉不拿拿先生
*
*/
@SuppressWarnings("rawtypes")
public class PageData extends HashMap implements Map{
private static final long serialVersionUID = 1L;
Map map = null;
HttpServletRequest request;
@SuppressWarnings("unchecked")
public PageData(HttpServletRequest request){
this.request = request;
Map properties = request.getParameterMap();
Map returnMap = new HashMap();
//将map的key和value组成的数据包装成一个Map.Entry对象
Iterator entries = properties.entrySet().iterator();
Map.Entry entry;
String name = "";
String value = "";
while (entries.hasNext()) {
//获取单个对象
entry = (Map.Entry) entries.next();
name = (String) entry.getKey();
Object valueObj = entry.getValue();
if(null == valueObj){
value = "";
}else if(valueObj instanceof String[]){
//值的类型是String类型的数组
String[] values = (String[])valueObj;
for(int i=0;i<values.length;i++){
value = values[i] + ",";
}
//value.length()-1 去掉最后一个 ","
value = value.substring(0, value.length()-1);
}else{
value = valueObj.toString();
}
//将每个解析出来的数据保存在map集合中
returnMap.put(name, value);
}
map = returnMap;
}
public PageData() {
map = new HashMap();
}
@Override
public Object get(Object key) {
Object obj = null;
if(map.get(key) instanceof Object[]) {
Object[] arr = (Object[])map.get(key);
obj = request == null ? arr:(request.getParameter((String)key) == null ? arr:arr[0]);
} else {
obj = map.get(key);
}
return obj;
}
public String getString(Object key) {
return (String)get(key);
}
@SuppressWarnings("unchecked")
@Override
public Object put(Object key, Object value) {
if(value instanceof ClobProxyImpl){ //读取oracle Clob类型数据
try {
ClobProxyImpl cpi = (ClobProxyImpl)value;
Reader is = cpi.getCharacterStream(); //获取流
BufferedReader br = new BufferedReader(is);
String str = br.readLine();
StringBuffer sb = new StringBuffer();
while(str != null){ //循环读取数据拼接到字符串
sb.append(str);
sb.append("\n");
str = br.readLine();
}
value = sb.toString();
} catch (Exception e) {
e.printStackTrace();
}
}
return map.put(key, value);
}
@Override
public Object remove(Object key) {
return map.remove(key);
}
public void clear() {
map.clear();
}
public boolean containsKey(Object key) {
return map.containsKey(key);
}
public boolean containsValue(Object value) {
return map.containsValue(value);
}
public Set entrySet() {
return map.entrySet();
}
public boolean isEmpty() {
return map.isEmpty();
}
public Set keySet() {
return map.keySet();
}
@SuppressWarnings("unchecked")
public void putAll(Map t) {
map.putAll(t);
}
public int size() {
return map.size();
}
public Collection values() {
return map.values();
}
}
三层架构的代码
dao层
package cn.linkpower.dao;
import java.util.List;
import org.apache.ibatis.annotations.Mapper;
import cn.linkpower.util.PageData;
import cn.linkpower.vo.Dept;
@Mapper //必须添加此项注解 不然接口和xml文件无法整合
public interface IDeptDAO {
//测试map传入传出
public List<Dept> getDeptMap(PageData pd)throws Exception;
}
service层
package cn.linkpower.service;
import java.util.List;
import java.util.Map;
import org.springframework.transaction.annotation.Propagation;
import org.springframework.transaction.annotation.Transactional;
import cn.linkpower.util.PageData;
import cn.linkpower.vo.Dept;
public interface IDeptService {
//采用传入map类型进行数据的添加操作
@Transactional(readOnly = true)
public List<Dept> getDeptMap(PageData pd) throws Exception;
}
实现层
package cn.linkpower.service.impl;
import java.util.List;
import javax.annotation.Resource;
import org.springframework.stereotype.Service;
import cn.linkpower.dao.IDeptDAO;
import cn.linkpower.service.IDeptService;
import cn.linkpower.util.PageData;
import cn.linkpower.vo.Dept;
@Service
public class DeptService implements IDeptService {
@Resource
private IDeptDAO deptDao;
//测试map方式传入和传出
@Override
public List<Dept> getDeptMap(PageData pd) throws Exception {
return this.deptDao.getDeptMap(pd);
}
}
基础控制层
package cn.linkpower.util;
import javax.servlet.http.HttpServletRequest;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import org.springframework.web.servlet.ModelAndView;
public class BaseController {
protected Logger logger = Logger.getLogger(this.getClass());
private static final long serialVersionUID = 6357869213649815390L;
/**
* new PageData对象
*
* @return
*/
public PageData getPageData() {
return new PageData(this.getRequest());
}
/**
* 得到ModelAndView
*
* @return
*/
public ModelAndView getModelAndView() {
return new ModelAndView();
}
/**
* 得到request对象
*
* @return
*/
public HttpServletRequest getRequest() {
HttpServletRequest request = ((ServletRequestAttributes) RequestContextHolder.getRequestAttributes())
.getRequest();
return request;
}
/**
* 得到32位的uuid
*
* @return
*/
public String get32UUID() {
return UuidUtil.get32UUID();
}
public static void logBefore(Logger logger, String interfaceName) {
logger.info("");
logger.info("start");
logger.info(interfaceName);
}
public static void logAfter(Logger logger) {
logger.info("end");
logger.info("");
}
}
实现代码的控制层
扫描二维码关注公众号,回复:
5499232 查看本文章
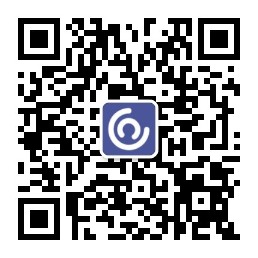
package cn.linkpower.controller;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletRequest;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import cn.linkpower.service.IDeptService;
import cn.linkpower.util.BaseController;
import cn.linkpower.util.JsonUtils;
import cn.linkpower.util.PageData;
import cn.linkpower.vo.Dept;
/**
* 测试改进的控制器效果(验证mybatis传入map类型)
*
* @author 香蕉不拿拿先生
*
*/
@Controller
public class TestController extends BaseController {
Logger log = LoggerFactory.getLogger(this.getClass());
@Resource
private IDeptService deptService;
@RequestMapping(value = "/getDeptMap", method = RequestMethod.GET)
@ResponseBody
public Object getDeptMap() throws Exception {
log.info("进入控制器");
// 创建map集合对象 保存参数信息
PageData pd = new PageData();
// 调用父类的定义方法 通过请求创建指定的PageData对象
pd = this.getPageData();
// 和request.getParameter一样 deptnum
String deptno = pd.getString("deptnum");
log.info("获取到的数据:" + deptno);
// 保存数据
pd.put("deptno", deptno);
List<Dept> deptList = this.deptService.getDeptMap(pd);
JsonUtils jsonUtils = new JsonUtils();
jsonUtils.setCode("200");
jsonUtils.setMsg("ok");
jsonUtils.setData(deptList);
return jsonUtils;
// http://localhost/getDeptMap?deptnum=1
}
}
json工具类
package cn.linkpower.util;
public class JsonUtils {
private String code;
private String msg;
private Object data;
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
public Object getData() {
return data;
}
public void setData(Object data) {
this.data = data;
}
@Override
public String toString() {
return "JsonUtils [code=" + code + ", msg=" + msg + ", data=" + data + "]";
}
}
运行springboot,访问http://localhost/getDeptMap?deptnum=3
数据库的结构
drop database if exists javademo;
create database mldn character set utf8;
use javademo;
create table dept(
deptno bigint Auto_increment,
dname varchar(50),
constraint pk_deptno primary key(deptno)
);
insert into dept(dname) values("开发部");
insert into dept(dname) values("财务部");
insert into dept(dname) values("市场部");
insert into dept(dname) values("后勤部");
insert into dept(dname) values("公关部");
我的代码的主要思想是使用map进行数据的保存操作,在mapper.xml文件中写的sql语句,是根据PageData类中保存的键值对进行参数的获取判断,然后进行查询数据库操作,如果数据参数很多,是不是也可以使用这个方式进行参数的保存pagadata和传递解析呢?
复杂的我暂未实现,如果大家有心得和更好的方式,还望大家能够分享下,小白不胜感激。
说到这里我在这追加说明一个上述的好的地方,application.yml中,我没有使用c3p0连接池,而是用的alibaba的?大家可以看下我写的配置项
package cn.linkpower.config;
import javax.sql.DataSource;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.boot.web.servlet.FilterRegistrationBean;
import org.springframework.boot.web.servlet.ServletRegistrationBean;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import com.alibaba.druid.pool.DruidDataSource;
import com.alibaba.druid.support.http.StatViewServlet;
import com.alibaba.druid.support.http.WebStatFilter;
/**
* 拓展技能:进行druid数据库连接池的性能的监控操作 需要做一些配置(例如:你访问的ip地址是否是白名单、数据库sql监控等)
* 访问方式: http://localhost/druid/
* @author 76519
*
*/
@Configuration
public class DruidConfig {
@Bean
public ServletRegistrationBean druidServlet() { // 主要实现WEB监控的配置处理
System.out.println("WEB监控的配置处理");
//想用使用 Servlet 功能,就必须要借用 Spring Boot 提供的 ServletRegistrationBean 接口
//访问方式http://localhost/druid/getAll 启动alibaba的druid数据库连接池自带的servlet
ServletRegistrationBean servletRegistrationBean = new ServletRegistrationBean(
new StatViewServlet(), "/druid/*"); // 现在要进行druid监控的配置处理操作
servletRegistrationBean.addInitParameter("allow",
"127.0.0.1,192.168.28.159"); // 白名单
servletRegistrationBean.addInitParameter("deny", "192.168.28.200"); // 黑名单
servletRegistrationBean.addInitParameter("loginUsername", "admin"); // 用户名
servletRegistrationBean.addInitParameter("loginPassword", "hello"); // 密码
servletRegistrationBean.addInitParameter("resetEnable", "false"); // 是否可以重置数据源
return servletRegistrationBean ;
}
@Bean
public FilterRegistrationBean filterRegistrationBean() {
FilterRegistrationBean filterRegistrationBean = new FilterRegistrationBean() ;
filterRegistrationBean.setFilter(new WebStatFilter());
filterRegistrationBean.addUrlPatterns("/*"); // 所有请求进行监控处理
filterRegistrationBean.addInitParameter("exclusions", "*.js,*.gif,*.jpg,*.css,/druid/*");
return filterRegistrationBean ;
}
@Bean
@ConfigurationProperties(prefix = "spring.datasource")
public DataSource druidDataSource() {
return new DruidDataSource();
}
}
然后启动Springboot项目,浏览器访问http://localhost/druid/login.html输入你配置中的写的用户名和密码。这个作为一个惊喜的发现分享下吧。