环境配置:windows 64,VS,SQLite(点击下载),System.Data.SQLite.DLL(点击下载)。
一、新建项目,添加引用
1.在VS中新建一个控制台应用程序,如下图
2.添加引用
将下载的System.Data.SQLite.DLL复制到新建项目的路径下
在VS中找到项目,右键选择添加引用
浏览到dll路径下,添加进来。
代码中添加
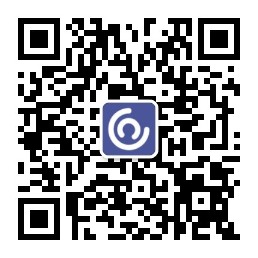
using System.Data.SQLite;
添加类库CSQLiteHelper,用于存放SQLite操作方法(此代码https://blog.csdn.net/qq_30725967/article/details/88169182)
具体代码
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Data.SQLite;
using System.Data;
using System.Xml;
using System.Text.RegularExpressions;
using System.IO;
namespace CSharp_SQLite
{
public class CSQLiteHelper
{
private string _dbName = "";
private SQLiteConnection _SQLiteConn = null; //连接对象
private SQLiteTransaction _SQLiteTrans = null; //事务对象
private bool _IsRunTrans = false; //事务运行标识
private string _SQLiteConnString = null; //连接字符串
private bool _AutoCommit = false; //事务自动提交标识
public string SQLiteConnString
{
set { this._SQLiteConnString = value; }
get { return this._SQLiteConnString; }
}
public CSQLiteHelper(string dbPath)
{
this._dbName = dbPath;
this._SQLiteConnString = "Data Source=" + dbPath;
}
/// <summary>
/// 新建数据库文件
/// </summary>
/// <param name="dbPath">数据库文件路径及名称</param>
/// <returns>新建成功,返回true,否则返回false</returns>
static public Boolean NewDbFile(string dbPath)
{
try
{
SQLiteConnection.CreateFile(dbPath);
return true;
}
catch (Exception ex)
{
throw new Exception("新建数据库文件" + dbPath + "失败:" + ex.Message);
}
}
/// <summary>
/// 创建表
/// </summary>
/// <param name="dbPath">指定数据库文件</param>
/// <param name="tableName">表名称</param>
static public void NewTable(string dbPath, string tableName)
{
SQLiteConnection sqliteConn = new SQLiteConnection("data source=" + dbPath);
if (sqliteConn.State != System.Data.ConnectionState.Open)
{
sqliteConn.Open();
SQLiteCommand cmd = new SQLiteCommand();
cmd.Connection = sqliteConn;
cmd.CommandText = "CREATE TABLE " + tableName + "(Name varchar,Team varchar, Number varchar)";
cmd.ExecuteNonQuery();
}
sqliteConn.Close();
}
/// <summary>
/// 打开当前数据库的连接
/// </summary>
/// <returns></returns>
public Boolean OpenDb()
{
try
{
this._SQLiteConn = new SQLiteConnection(this._SQLiteConnString);
this._SQLiteConn.Open();
return true;
}
catch (Exception ex)
{
throw new Exception("打开数据库:" + _dbName + "的连接失败:" + ex.Message);
}
}
/// <summary>
/// 打开指定数据库的连接
/// </summary>
/// <param name="dbPath">数据库路径</param>
/// <returns></returns>
public Boolean OpenDb(string dbPath)
{
try
{
string sqliteConnString = "Data Source=" + dbPath;
this._SQLiteConn = new SQLiteConnection(sqliteConnString);
this._dbName = dbPath;
this._SQLiteConnString = sqliteConnString;
this._SQLiteConn.Open();
return true;
}
catch (Exception ex)
{
throw new Exception("打开数据库:" + dbPath + "的连接失败:" + ex.Message);
}
}
/// <summary>
/// 关闭数据库连接
/// </summary>
public void CloseDb()
{
if (this._SQLiteConn != null && this._SQLiteConn.State != ConnectionState.Closed)
{
if (this._IsRunTrans && this._AutoCommit)
{
this.Commit();
}
this._SQLiteConn.Close();
this._SQLiteConn = null;
}
}
/// <summary>
/// 开始数据库事务
/// </summary>
public void BeginTransaction()
{
this._SQLiteConn.BeginTransaction();
this._IsRunTrans = true;
}
/// <summary>
/// 开始数据库事务
/// </summary>
/// <param name="isoLevel">事务锁级别</param>
public void BeginTransaction(IsolationLevel isoLevel)
{
this._SQLiteConn.BeginTransaction(isoLevel);
this._IsRunTrans = true;
}
/// <summary>
/// 提交当前挂起的事务
/// </summary>
public void Commit()
{
if (this._IsRunTrans)
{
this._SQLiteTrans.Commit();
this._IsRunTrans = false;
}
}
}
}
此时运行会报错,
警告 所生成项目的处理器架构“MSIL”与引用“System.Data.SQLite”的处理器架构“x86”不匹配。这种不匹配可能会导致运行时失败。请考虑通过配置管理器更改您的项目的目标处理器架构,以使您的项目与引用间的处理器架构保持一致,或者为引用关联一个与您的项目的目标处理器架构相符的处理器架构。
修改项目属性:x86。
再次运行,无误。
二、创建数据库
SQLite 是文件型的数据库,后缀名可以是".db3"、".db"或者“.sqlite”,甚至可以由你决定它的后缀。其中前3个类型是SQLite默认类型。
新建一个数据库文件,代码如下
1 /// <summary>
2 /// 新建数据库文件
3 /// </summary>
4 /// <param name="dbPath">数据库文件路径及名称</param>
5 /// <returns>新建成功,返回true,否则返回false</returns>
6 static public Boolean NewDbFile(string dbPath)
7 {
8 try
9 {
10 SQLiteConnection.CreateFile(dbPath);
11 return true;
12 }
13 catch (Exception ex)
14 {
15 throw new Exception("新建数据库文件" + dbPath + "失败:" + ex.Message);
16 }
17 }
三、创建表
1 /// <summary>
2 /// 创建表
3 /// </summary>
4 /// <param name="dbPath">指定数据库文件</param>
5 /// <param name="tableName">表名称</param>
6 static public void NewTable(string dbPath, string tableName)
7 {
8
9 SQLiteConnection sqliteConn = new SQLiteConnection("data source=" + dbPath);
10 if (sqliteConn.State != System.Data.ConnectionState.Open)
11 {
12 sqliteConn.Open();
13 SQLiteCommand cmd = new SQLiteCommand();
14 cmd.Connection = sqliteConn;
15 cmd.CommandText = "CREATE TABLE " + tableName + "(Name varchar,Team varchar, Number varchar)";
16 cmd.ExecuteNonQuery();
17 }
18 sqliteConn.Close();
19 }
例子:创建一个数据库文件 NBA.db3
然后创建表Stars,再创建表的列,Name,Team,Number。
1 class Program
2 {
3 private static string dbPath = @"d:\NBA.db3";
4 static void Main(string[] args)
5 {
6 //创建一个数据库db文件
7 CSQLiteHelper.NewDbFile(dbPath);
8 //创建一个表
9 string tableName = "Stars";
10 CSQLiteHelper.NewTable(dbPath, tableName);
11 }
12 }
看下效果,数据库文件NBA的表Stars中有Name,Team和Number字段
四、插入数据
接下来,使用SQLite 的 INSERT INTO 语句用于向数据库的某个表中添加新的数据行。
先在Main()方法中创建一些数据。
1 List<object[]> starsDatas = new List<object[]>();
2 starsDatas.Add(new object[] { "Garnett", "Timberwolves", "21" });
3 starsDatas.Add(new object[] { "Jordan", "Bulls", "23" });
4 starsDatas.Add(new object[] { "Kobe", "Lakers", "24" });
5 starsDatas.Add(new object[] { "James", "Cavaliers", "23" });
6 starsDatas.Add(new object[] { "Tracy", "Rockets", "1" });
7 starsDatas.Add(new object[] { "Carter", "Nets", "15" });
插入到表单中
1 private static void AddStars(List<object[]> stars)
2 {
3 CSQLiteHelper sqlHelper = new CSQLiteHelper(dbPath);
4 sqlHelper.OpenDbConn();
5 string commandStr = "insert into Stars(Name,Team,Number) values(@name,@team,@number)";
6 foreach (var item in stars)
7 {
8 if (isExist("Name", item[0].ToString()))
9 {
10 Console.WriteLine(item[0] + "的数据已存在!");
11 continue;
12 }
13 else
14 {
15 sqlHelper.ExecuteNonQuery(commandStr, item);
16 Console.WriteLine(item[0] + "的数据已保存!");
17 Console.ReadKey();
18 }
19 }
20 sqlHelper.CloseDbConn();
21 Console.ReadKey();
22
23 }
效果如下:
五、查询数据
SQLite 的 SELECT 语句用于从 SQLite 数据库表中获取数据,以结果表的形式返回数据。这些结果表也被称为结果集。
一个简单的例子:查询一个球星所在的球队。
输入球星的名字,输出球队。
1 private static void Team(string name)
2 {
3 CSQLiteHelper sqliteHelper = new CSQLiteHelper(dbPath);
4 sqliteHelper.OpenDbConn();
5 string commandText = @"select * from Stars where Name ='" + name+"'";
6 DataTable dt = sqliteHelper.Query(commandText).Tables[0];
7 if (dt.Rows.Count == 0)
8 {
9 Console.WriteLine("查无此人!");
10 Console.ReadLine();
11 sqliteHelper.CloseDbConn();
12 return;
13 }
14 string team = dt.Rows[0]["Team"].ToString();
15 sqliteHelper.CloseDbConn();
16 Console.WriteLine(name + "--" + team);
17 Console.ReadKey();
18 }
1 static void Main(string[] args)
2 {
3 Console.WriteLine("请输入一个Star:");
4 string name = Console.ReadLine();
5 Team(name);
6 }
效果如下
六、删除数据
SQLite 的 DELETE 语句用于删除表中已有的记录。可以使用带有 WHERE 子句的 DELETE 查询来删除选定行,否则所有的记录都会被删除。
1 private static void Delete(string name)
2 {
3 CSQLiteHelper sqliteHelper = new CSQLiteHelper(dbPath);
4 sqliteHelper.OpenDbConn();
5 string commandtext = "delete from Stars where Name = '" + name + "'";
6 sqliteHelper.ExecuteNonQuery(commandtext);
7 sqliteHelper.CloseDbConn();
8 Console.WriteLine(name + "被删除!");
9 }
注意:delete语句与select语句不同,delete后直接跟from,不能写成:
"delete * from Stars where [condition];
调用一下
Console.WriteLine("删除球星:");
string starName = Console.ReadLine();
Delete(starName);
删除前:
删除后:
七、运算符
运算符是一个保留字或字符,主要用于 SQLite 语句的 WHERE 子句中执行操作,如比较和算术运算。
运算符用于指定 SQLite 语句中的条件,并在语句中连接多个条件。
可以直接在SQLite语句中加入运算符,= - * / % > < = >= <= 等等
例:
下面代码是首先插入一些NBA球员的信息,然后筛选出球衣号码大于10的球员:
1 using System;
2 using System.Collections.Generic;
3 using System.Data.SQLite;
4 using System.Data;
5 using System.IO;
6 using System.Data.Common;
7 using System.Diagnostics;
8
9
10 namespace CSharp_SQLite
11 {
12 class Program
13 {
14
15 static void Main(string[] args)
16 {
17 string dbPath = "NBAStars.nba";
18 Stopwatch timer = new Stopwatch();
19 timer.Start();
20 CSQLiteHelper sqlhelper = new CSQLiteHelper(dbPath);
21 sqlhelper.OpenDbConn();
22 sqlhelper.BeginTransaction();
23 sqlhelper.ExecuteNonQuery("delete from Stars");
24 #region 球员信息
25 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Michael Jordan','Bulls','23')");
26 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('James Harden','Rockets','13')");
27 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Lebron James','Cavaliers','23')");
28 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Tracy Mcgrady','Rockets','1')");
29 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Yao Ming','Rockets','11')");
30 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Kevin Garnett','Timberwolves','21')");
31 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Tim Duncan','Supers','21')");
32 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Vince Carter','Nets','15')");
33 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Michael Carter Williams','76ers','10')");
34 #endregion
35 sqlhelper.Commit();
36 sqlhelper.CloseDbConn();
37 timer.Stop();
38 Console.WriteLine("初始化数据库表单用时:" + timer.Elapsed);
39
40
41 CSQLiteHelper sqlHelper = new CSQLiteHelper(dbPath);
42 sqlHelper.OpenDbConn();
43 sqlHelper.BeginTransaction();
44 DataTable dt = sqlHelper.Query("select Name, Number from Stars where Number > 10").Tables[0];
45 sqlHelper.Commit();
46 sqlHelper.CloseDbConn();
47 Console.WriteLine("球衣号码大于10的球员有:");
48 for (int i = 0; i < dt.Rows.Count; i++)
49 {
50 Console.WriteLine(dt.Rows[i]["Name"] + "\t" + dt.Rows[i]["Number"]);
51 }
52 Console.ReadLine();
53 }
54
55 }
56
57 }
结果如下:
若想输出这些球员的球衣号码,则应该在select语句修改为
DataTable dt = sqlHelper.Query("select Name, Number from Stars where Number > 10").Tables[0];
将输出修改为
Console.WriteLine(dt.Rows[i]["Name"] + "\t" + dt.Rows[i]["Number"]);
结果如下:
注:select语句中尽量不要使用select * from,那样会影响效率。
八、like语句
SQLite 的 LIKE 运算符是用来匹配通配符指定模式的文本值。如果搜索表达式与模式表达式匹配,LIKE 运算符将返回真(true),也就是 1。这里有两个通配符与 LIKE 运算符一起使用:
- 百分号 %
- 下划线 _
百分号(%)代表零个、一个或多个数字或字符。下划线(_)代表一个单一的数字或字符。这些符号可以被组合使用。
语法
% 和 _ 的基本语法如下:
SELECT column_list
FROM table_name
WHERE column LIKE 'XXXX%'
or
SELECT column_list
FROM table_name
WHERE column LIKE '%XXXX%'
or
SELECT column_list
FROM table_name
WHERE column LIKE 'XXXX_'
or
SELECT column_list
FROM table_name
WHERE column LIKE '_XXXX'
or
SELECT column_list
FROM table_name
WHERE column LIKE '_XXXX_'
您可以使用 AND 或 OR 运算符来结合 N 个数量的条件。在这里,XXXX 可以是任何数字或字符串值。
例
我们在球员中筛选出名字中包含James的球员
1 using System;
2 using System.Collections.Generic;
3 using System.Data.SQLite;
4 using System.Data;
5 using System.IO;
6 using System.Data.Common;
7 using System.Diagnostics;
8
9
10 namespace CSharp_SQLite
11 {
12 class Program
13 {
14
15 static void Main(string[] args)
16 {
17 string dbPath = "NBAStars.nba";
18 Stopwatch timer = new Stopwatch();
19 timer.Start();
20 CSQLiteHelper sqlhelper = new CSQLiteHelper(dbPath);
21 sqlhelper.OpenDbConn();
22 sqlhelper.BeginTransaction();
23 sqlhelper.ExecuteNonQuery("delete from Stars");
24 #region 球员信息
25 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Michael Jordan','Bulls','23')");
26 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('James Harden','Rockets','13')");
27 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Lebron James','Cavaliers','23')");
28 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Tracy Mcgrady','Rockets','1')");
29 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Yao Ming','Rockets','11')");
30 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Kevin Garnett','Timberwolves','21')");
31 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Tim Duncan','Supers','21')");
32 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Vince Carter','Nets','15')");
33 sqlhelper.ExecuteNonQuery("insert into Stars (Name,Team,Number) values('Michael Carter Williams','76ers','10')");
34 #endregion
35 sqlhelper.Commit();
36 sqlhelper.CloseDbConn();
37 timer.Stop();
38 Console.WriteLine("初始化数据库表单用时:" + timer.Elapsed);
39
40
41 CSQLiteHelper sqlHelper = new CSQLiteHelper(dbPath);
42 sqlHelper.OpenDbConn();
43 sqlHelper.BeginTransaction();
44 DataTable dt = sqlHelper.Query("select Name, Number from Stars where Name like '%James%'").Tables[0];
45 sqlHelper.Commit();
46 sqlHelper.CloseDbConn();
47 Console.WriteLine("名字中包含James的球员有:");
48 for (int i = 0; i < dt.Rows.Count; i++)
49 {
50 Console.WriteLine(dt.Rows[i]["Name"] + "\t" + dt.Rows[i]["Number"]);
51 }
52 Console.ReadLine();
53 }
54
55 }
56
57 }
其中第44行代码 DataTable dt = sqlHelper.Query("select Name, Number from Stars where Name like '%James%'").Tables[0];
%James%表示前面和后面包含零个、一个或多个数字或字符,也就是包含名字内James
运行结果如下
注:SQLite 是一个软件库,实现了自给自足的、无服务器的、零配置的、事务性的 SQL 数据库引擎。SQLite 源代码不受版权限制。SQLite 是在世界上最广泛部署的 SQL 数据库引擎。SQLite是世界上应用最广泛的数据库,拥有着不计其数的应用