摘要如下:
JUNIT做数据驱动的测试比较麻烦,所谓数据驱动的测试,就是比如同样的一个方法,能用EXCEL,CSV等格式提供大量的不同测试数据给测试去验证,这里可以使用
easytest这个不错的开源工具,地址在:https://github.com/EaseTech/easytest
下面例子讲解:
1) 比如一个加法的:
public class Calculator { public Double add(Double a, Double b){ return a+b; } }
2) MAVEN引入EASYTEST
<dependency> <groupId>org.easetech</groupId> <artifactId>easytest-core</artifactId> <version>1.4.0</version> </dependency>
3 然后可以编制CSV或者EXCEL,记得第1列是要测试的方法名,内容留空,
然后是每列的参数
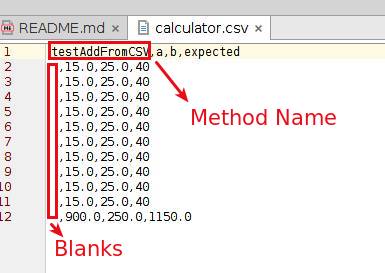
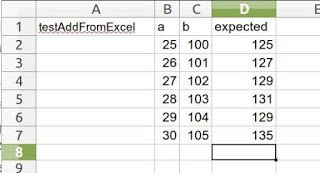
4 CSV测试的:
@RunWith(DataDrivenTestRunner.class) public class CSVLoaderExample extends DataDrivenTest { @Test @DataLoader(filePaths = "calculator.csv", loaderType = LoaderType.CSV) public String testAddFromCSV(@Param(name = "a") Double a, @Param(name = "b") Double b, @Param(name = "expected") Double expected) { Assert.assertEquals(expected, calculator.add(a, b), 0.1); return "success"; } @Test @DataLoader(filePaths = "calculator2.csv") public void testAdd(@Param(name = "a") Double a, @Param(name = "b")Double b, @Param(name = "expected")Double expected){ Assert.assertEquals(expected, calculator.add(a,b),0.1); } }
首先继承DataDrivenTest ,然后用注解 @DataLoader(filePaths = "calculator.csv", loaderType = LoaderType.CSV) 标识是用CSV,XML还是EXCEL
然后@Param(name = "a")中的NAME就是对应EXCEL或CSV文件中的列的标题了
EXCEL也是一样:
@RunWith(DataDrivenTestRunner.class) public class ExcelLoaderExample extends DataDrivenTest { @Test @DataLoader(filePaths = "calculator.xls", loaderType = LoaderType.EXCEL) public void testAddFromExcel(@Param(name = "a") Double a, @Param(name = "b") Double b, @Param(name = "expected") Double expected) { Assert.assertEquals(expected, calculator.add(a, b), 0.1); } @Test @DataLoader(filePaths = {"calculator2.xls"}) public void testAdd(@Param(name = "a") Double a, @Param(name = "b")Double b, @Param(name = "expected")Double expected){ Assert.assertEquals(expected, calculator.add(a,b),0.1); } }
4 还有不错的报表功能,可以输出:
@RunWith(DataDrivenTestRunner.class) @Report(outputLocation = "classpath:TestReports") public class ClassPathExampleReport extends DataDrivenTest{ @Test @DataLoader(filePaths = "calculator.xls") public void testAddFromExcel(@Param(name = "a") Double a, @Param(name = "b") Double b, @Param(name = "expected") Double expected) { Assert.assertEquals(expected, calculator.add(a, b), 0.1); } @Test @DataLoader(filePaths = "calculator2.xls") public void testAdd(@Param(name = "a") Double a, @Param(name = "b") Double b, @Param(name = "expected") Double expected) { Assert.assertEquals(expected, calculator.add(a, b), 0.1); } }
这样就在TARGET目录下有个目录是TestReports,
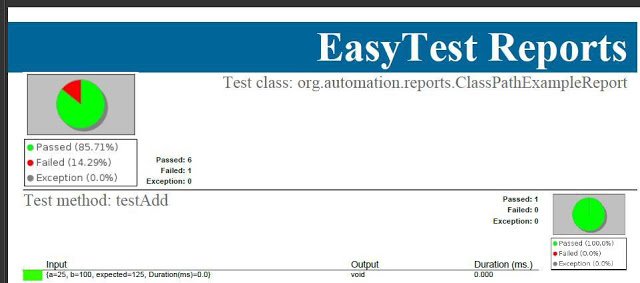