相关代码下载链接:
http://download.csdn.net/detail/stevenhu_223/4884357
在有些时候,我们需要对我们自己编写的代码进行单元测试(好处是,减少后期维护的精力和费用),这是一些最基本的模块测试。当然,在进行单元测试的同时也必然得清楚我们测试的代码的内部逻辑实现,这样在测试的时候才能清楚地将我们希望代码逻辑实现得到的结果和测试实际得到的结果进行验证对比。
废话少说,上代码:
首先创建一个java工程,在工程中创建一个被单元测试的Student数据类,如下:
package com.phicomme.hu;
public class Student
{
private String name;
private String sex;
private int high;
private int age;
private String school;
public Student(String name, String sex ,int high, int age, String school)
{
this.name = name;
this.sex = sex;
this.high = high;
this.age = age;
this.school = school;
}
public String getName()
{
return name;
}
public void setName(String name)
{
this.name = name;
}
public String getSex()
{
return sex;
}
public void setSex(String sex)
{
this.sex = sex;
}
public int getHigh()
{
return high;
}
public void setHigh(int high)
{
this.high = high;
}
public int getAge()
{
return age;
}
public boolean setAge(int age)
{
if (age >25)
{
return false;
}
else
{
this.age = age;
return true;
}
}
public String getSchool()
{
return school;
}
public void setSchool(String school)
{
this.school = school;
}
}
在eclipse下单元测试这个类:
首先导入Junit包:选中java工程,点击鼠标右键--->选择properties---->在窗口中选Java Build Path---->在右侧点击Add Library---->在弹出的窗口列表中选中Junit---->下一步----->Junit 4(我用的是Junit 4)---->finish
这样Junit 4包就导完了,接下来就是创建测试类:
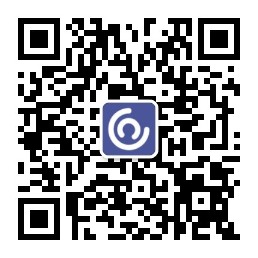
将测试类和被测试类放在不同的包中(也可以放在同一个包中,此处只是为了区别),代码如下:
测试类1:
package com.phicomme.test;
import com.phicomme.hu.Student;
import junit.framework.TestCase;
public class StudentTest01 extends TestCase
{
Student testStudent;
//此方法在执行每一个测试方法之前(测试用例)之前调用
@Override
protected void setUp() throws Exception
{
// TODO Auto-generated method stub
super.setUp();
testStudent = new Student("djm", "boy", 178, 24, "华东政法");
System.out.println("setUp()");
}
//此方法在执行每一个测试方法之后调用
@Override
protected void tearDown() throws Exception
{
// TODO Auto-generated method stub
super.tearDown();
System.out.println("tearDown()");
}
//测试用例,测试Person对象的getSex()方法
public void testGetSex()
{
assertEquals("boy", testStudent.getSex());
System.out.println("testGetSex()");
}
//测试Person对象的getAge()方法
public void testGetAge()
{
assertEquals(24, testStudent.getAge());
System.out.println("testGetAge()");
}
}
测试类2:
package com.phicomme.test;
import junit.framework.TestCase;
import com.phicomme.hu.Student;
public class StudentTest extends TestCase
{
private Student testStudent;
@Override
protected void setUp() throws Exception
{
// TODO Auto-generated method stub
super.setUp();
testStudent = new Student("steven_hu", "boy", 170 , 23, "上海理工");
}
@Override
protected void tearDown() throws Exception
{
// TODO Auto-generated method stub
super.tearDown();
}
public void testSetage()
{
assertTrue(testStudent.setAge(21));
}
public void testGetSchool()
{
//预期值和实际值不一样,测试时出现失败(Failure)
assertEquals("南昌大学", testStudent.getSchool());
}
public void testGetName()
{
assertEquals("hdy", testStudent.getName());
}
}
当然,如果同时需要一起测试以上这两个测试类,可以通过TestSuite类实现,它相当于是一个套件,可以把所有测试类添进来一起运行测试;
代码如下:
package com.phicomme.test;
import com.phicomme.hu.StudentTest02;
import junit.framework.Test;
import junit.framework.TestSuite;
public class AllTest
{
//static PersonTest p = new PersonTest();
//static PersonTest p1 = new PersonTest();
public static Test suite()
{
TestSuite suite = new TestSuite("Test for com.phicomme.test");
//suite.addTest(p);
//suite.addTest(p1);
suite.addTestSuite(StudentTest.class);
suite.addTestSuite(StudentTest01.class);
return suite;
}
}
最后,分别测试以上三个类(选中需要测试的类---->鼠标右键---->Run As---->Junit Test):
StudentTest类的测试结果图:
StudentTest01类的测试结果图:
AllTest类的测试结果图:
有关java的测试就讲到这里,希望对大家有帮助,有时间也会接着讲讲有关android的单元测试,和在手机上实现编写一个UI界面替代eclipse如上图中的测试界面;
转载请注明本文地址: java单元测试(Junit)