题目
给定一个排序链表,删除所有重复的元素,使得每个元素只出现一次。
示例 1:
输入: 1->1->2
输出: 1->2
示例 2:
输入: 1->1->2->3->3
输出: 1->2->3
方法一,将无重复值的结点添加到一个新链表
思路:
原链表不变,新建一个链表,遍历原链表,将原链表第一个结点值赋值为flag,若当前结点的值不等于flag,则将该结点插入新链表,若等于则原链表向下继续遍历
head:原链表的头结点 h:新链表的头结点 current:遍历新链表的结点 flag:原链表当前结点的值
python3代码:
# 定义单链表
class ListNode:
def __init__(self, x):
self.val = x
self.next = None
class Solution:
def deleteDuplicates(self, head):
"""
:type head: ListNode
:rtype: ListNode
"""
if head == None:
return None
h = ListNode(head.val)
current = h
flag = head.val
while head != None:
if flag == head.val:
head = head.next
else:
current.next = ListNode(head.val)
current = current.next
flag = head.val
head = head.next
return h
运行时间:
方法二,在原链表上删除有重复值的结点
思路:
在原链表上修改。判断头元素与头元素下一节点的值是否相等,若相等则cur.next = cur.next.next,若不等则继续向下遍历
扫描二维码关注公众号,回复:
5312572 查看本文章
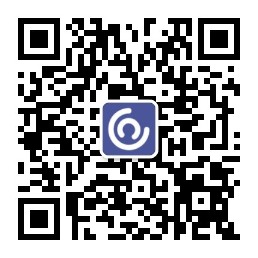
python3代码:
class Solution:
def deleteDuplicates(self, head):
"""
:type head: ListNode
:rtype: ListNode
"""
if head is None:
return head
cur = head
while cur.next:
if cur.val == cur.next.val:
cur.next = cur.next.next
else:
cur = cur.next
return head
运行时间: