The count-and-say sequence is the sequence of integers with the first five terms as following:
1. 1
2. 11
3. 21
4. 1211
5. 111221
1
is read off as "one 1"
or 11
.11
is read off as "two 1s"
or 21
.21
is read off as "one 2
, then one 1"
or 1211
.
Given an integer n, generate the nth term of the count-and-say sequence.
Note: Each term of the sequence of integers will be represented as a string.
Example 1:
Input: 1
Output: "1"
Example 2:
Input: 4
Output: "1211"
首先说一下题意:
n=1时输出字符串1;n=2时,数上次字符串中的数值个数,因为上次字符串有1个1,所以输出11;n=3时,由于上次字符是11,有2个1,所以输出21;n=4时,由于上次字符串是21,有1个2和1个1,所以输出1211。
自己写的好像理解错题意了所以删掉了。。
以下是参考的c语言代码示例:
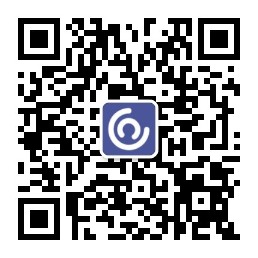
char* countAndSay(int n) {
char *result = NULL;//指针没有指向确定的内存空间
if (n == 1) {
result = malloc(2);//用malloc开辟一个2字节的内存空间,用来放result数组
result[0] = '1';
result[1] = '\0';
return result;//在c 语言中遇到return语句就结束,所以不用把后面的过程都放在else里
}
char *str = countAndSay(n - 1);//递归
int len = strlen(str);//strlen输出数组长度
result = malloc(2 * len * (sizeof *result));//函数的用法是malloc(内存大小);新的数组最长为之前数组的两倍长度,所以开辟一个两倍空间;sizeof返回*result所占的内存,是内存的单位值
int resultPos = 0;//result数组的下标
char curChar = str[0];//第一个字符
int curCnt = 1;//计数为1
for (int i = 1; i < len; i++) {
if (curChar == str[i]) {
curCnt++;//如果相同字符则计数+1
continue;
} else {
result[resultPos] = curCnt + '0';//如果不同字符,则'0'+计数,存在result[resultPos]中
resultPos++;//下标加一
result[resultPos] = curChar;//把字符存在下一个result[resultPos]中
resultPos++;//下标再加一
curChar = str[i]; //这时重新置字符为不同的字符
curCnt = 1;//计数回到1
}
}
if (curCnt != 0) {
result[resultPos] = curCnt + '0';
resultPos++;
result[resultPos] = curChar;
resultPos++;
}//最后一次的字符和计数没有经过不同字符出现时候的存储,所以单独存起来一遍
result[resultPos] = '\0';//字符串末尾为'\0'
result = realloc(result, resultPos + 1);//realloc函数用法为:(数据类型*)realloc(要改变内存大小的指针名,新的大小)
free(str);//释放str,str是中间过程的数组
return result;
}
以下是java版本示例:
class Solution {
public String countAndSay(int n) {
if (n <= 1) {
return "1";
}//初始化
String prev = countAndSay(n-1);//现在的字符串与之前的字符串是递归的关系,要注意,在递归之前要将初始字符串设置好,然后后面直接用递归结果就可以了,并不复杂,String需要大写
StringBuilder curr = new StringBuilder();//java中新建不确定长度的字符串用StringBuilder,后面用append添加元素即可
for (int i = 0; i < prev.length(); ) {
char c = prev.charAt(i);//string型的字符串用charAt(i)可以获得
int count = 1;//两层循环结构,第一层遍历前字符串的每一个字符,第二层以j计数,从i开始看后面有几个数跟i相同,遇到不同的会终止第二层循环。也就是2112读作122112,2并不合并。
while (i+count < prev.length() && prev.charAt(i+count) == c) {
count++;//以i为基准,向后扫描count位,扫描后跳转到i+count位置
}
curr.append(count).append(c);
i += count;//读了一段数之后,添加元素,把i指向这段后面的内容
}
return curr.toString();//用.toString()来返回字符串,不用会报错:StringBuilder cannot be converted to String
}
}