最近在写项目的时候遇到要求使用tablayout和fragment,遇到了这里记录一下大致思路。
tablayout是头部可以左右切换的头部控制栏控件,配合viewpager使用,fragment是碎片,可以放在viewpager里面,实现类似网易云音乐首页切换的效果。效果图如下:
首先添在build.gradle里面添加依赖:
1 implementation 'com.android.support:support-v4:28.0.0' 2 implementation 'com.android.support:design:28.0.0'
然后在布局文件里写好tablayout和viewpager
1 <?xml version="1.0" encoding="utf-8"?> 2 <android.support.v4.widget.DrawerLayout 3 xmlns:android="http://schemas.android.com/apk/res/android" 4 xmlns:app="http://schemas.android.com/apk/res-auto" 5 xmlns:tools="http://schemas.android.com/tools" 6 android:id="@+id/drawer_layout" 7 android:layout_width="match_parent" 8 android:layout_height="match_parent" 9 android:fitsSystemWindows="true" 10 tools:openDrawer="start"> 11 12 13 <LinearLayout 14 android:layout_width="match_parent" 15 android:layout_height="match_parent" 16 android:orientation="vertical" 17 app:layout_behavior="@string/appbar_scrolling_view_behavior"> 18 19 <android.support.v7.widget.Toolbar 20 android:id="@+id/toolbar" 21 android:layout_width="match_parent" 22 android:layout_height="?attr/actionBarSize" 23 android:background="#06a5fa" 24 app:popupTheme="@style/AppTheme.PopupOverlay" /> 25 26 27 <android.support.design.widget.TabLayout 28 android:id="@+id/Tablayout" 29 app:tabBackground="@android:color/white" 30 app:tabIndicatorColor="@color/colorAccent" 31 app:tabTextColor="@android:color/black" 32 android:layout_width="match_parent" 33 android:layout_height="wrap_content"/> 34 35 <android.support.v4.view.ViewPager 36 android:id="@+id/Viewpager" 37 android:layout_width="match_parent" 38 android:layout_height="match_parent"> 39 </android.support.v4.view.ViewPager> 40 </LinearLayout> 41 42 <include 43 app:layout_behavior="@string/appbar_scrolling_view_behavior" 44 layout="@layout/app_bar_main" 45 android:layout_width="match_parent" 46 android:layout_height="match_parent" /> 47 48 <android.support.design.widget.NavigationView 49 android:id="@+id/nav_view" 50 android:layout_width="wrap_content" 51 android:layout_height="match_parent" 52 android:layout_gravity="start" 53 android:fitsSystemWindows="true" /> 54 55 </android.support.v4.widget.DrawerLayout>
由于tablayout只是项目要求中的一个,所以代码中有一些其他的布局文件,重点看tablayout和viewpager
然后首先在activity里面初始化控件和两个list还有要用到的fragment:
1 private TabLayout tabLayout; 2 private ViewPager viewPager;
1 private ArrayList<String> TitleList = new ArrayList<>(); //页卡标题集合 2 private ArrayList<Fragment> ViewList = new ArrayList<>(); //页卡视图集合 3 private Fragment all_Fragment,recent_Fragment,like_Fragment; //页卡视图
titlelist用来储存tab的标题,viewlist用来储存视图,剩下的三个fragment就是我们要呈现的视图,当然上面代码中的三个fragment是我继承fragment后自己重写的,代码如下:
1 public class All_Fragment extends Fragment { 2 3 4 private View rootView; 5 private RecyclerView recyclerView; 6 private SwipeRefreshLayout swipeRefreshLayout; 7 private StringBuilder response; 8 List<Map<String,Object>> list=new ArrayList<>(); 9 10 11 12 private int flag; 13 public static final int GET_DATA_SUCCESS = 1; 14 private String id; 15 private String thumbnail; 16 private String description; 17 private String name; 18 19 20 @Override 21 public void onAttach(Context context){ 22 super.onAttach(context); 23 } 24 25 26 @Override 27 public View onCreateView(@Nullable LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState){ 28 rootView = inflater.inflate(R.layout.activity_all_column_,container,false); 29 32 initUi(); 33 return rootView; 34 } 35 36 private void initUi(){ 37 //这里写加载布局的代码 38 } 39 40 @Override 41 public void onActivityCreated(Bundle savedInstanceState){ 42 super.onActivityCreated(savedInstanceState); 43 //这里写逻辑代码 44 } 45 }
篇幅原因,这里只贴一个fragment的代码,另外两个类似.
扫描二维码关注公众号,回复:
5301207 查看本文章
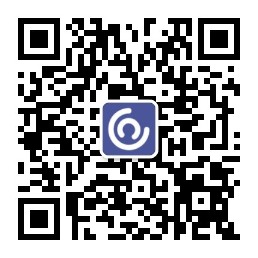
上述工作完成之后,在activity的oncreate方法中找到我们在布局文件xml中定义的控件:
1 //首先找到tablayout控件和view pager控件 2 tabLayout = findViewById(R.id.Tablayout); 3 viewPager = findViewById(R.id.Viewpager);
然后将fragment在后面new出来:
1 all_Fragment = new All_Fragment(); 2 recent_Fragment = new Recent_Fragment(); 3 like_Fragment = new Like_Fragment();
然后将fragment添加到页卡视图的集合里面去:
1 //添加页卡视图 2 ViewList.add(all_Fragment); 3 ViewList.add(recent_Fragment); 4 ViewList.add(like_Fragment);
将想要设置的tab标题添加到titlelist:
1 //添加页卡标题 2 TitleList.add("栏目总览"); 3 TitleList.add("热门消息"); 4 TitleList.add("我的收藏");
设置tab的显示模式并添加tab选项卡:
1 //设置tab模式 2 tabLayout.setTabMode(TabLayout.MODE_FIXED); 3 //添加tab选项卡 4 tabLayout.addTab(tabLayout.newTab().setText(TitleList.get(0))); 5 tabLayout.addTab(tabLayout.newTab().setText(TitleList.get(1))); 6 tabLayout.addTab(tabLayout.newTab().setText(TitleList.get(2)));
设置viewpager的adapter并与tab绑定:
1 //设置adapter 2 viewPager.setAdapter(new FragmentPagerAdapter(getSupportFragmentManager()){ 3 4 //获取每个页卡 5 @Override 6 public android.support.v4.app.Fragment getItem(int position){ 7 return ViewList.get(position); 8 } 9 10 //获取页卡数 11 @Override 12 public int getCount(){ 13 return TitleList.size(); 14 } 15 16 //获取页卡标题 17 @Override 18 public CharSequence getPageTitle(int position){ 19 return TitleList.get(position); 20 } 21 }); 22 23 //tab与viewpager绑定 24 tabLayout.setupWithViewPager(viewPager);
这样大致框架就搭建好了,想要实现的具体内容可以写在fragment里面,下面是我简单加载图片后的效果:
菜鸟一枚,有什么错误的地方请多指正,感激不尽!