一.Ice是什么
ICE是ZEROC的开源通信协议产品,是一个面向对象的中间件,使我们能够以最小的代价构建分布式应用程序。ICE使我们专注于应用逻辑的开发,它来处理所有底层的网络接口编程,这样我们就不用去考虑这样的细节:打开网络连接、网络数据传输的序列化与反序列化、连接失败的尝试次数等。
二.在Qt中嵌入Ice框架
在Qt中调用Ice通信框架时,是先启动Ice框架,再在Ice中run()函数启动Qt界面。
main.cpp
#include "ice/microwaveclient.h"
//#include <QApplication>
int main(int argc, char *argv[])
{
//先启动ice,再启动主界面
// QApplication a(argc, argv);
MicrowaveClient *app = MicrowaveClient::getInstance();
return app->main(argc,argv,"Config.Client");
}
类MicrowaveClient继承QObject和Ice::Application,并在该类中实现run函数,并将此类设计为单例类:
class MicrowaveClient : public QObject,public Ice::Application
MicrowaveClient.cpp:
static MicrowaveClient* m_client = NULL;
//单例模式
MicrowaveClient* MicrowaveClient::getInstance()
{
if(m_client == NULL)
{
m_client = new MicrowaveClient();
}
return m_client;
}
//回调类
class LogCallbackReceiverI : public LogCallbackReceiver
{
public:
LogCallbackReceiverI():LogCallbackReceiver()
{
}
virtual void WaiteCaptureEnd(LogEnum lotType, const string& msg, const Ice::Current& = ::Ice::Current())
{
//处理服务端返回的信息
}
};
//run函数
int MicrowaveClient::run(int argc, char *argv[])
{
if(argc > 1)
{
cerr << appName() << ": too many arguments" << endl;
return EXIT_FAILURE;
}
//----------------创建qt线程--------------------
QApplication a(argc, argv);
QFile qss(":/res/style.qss");
if(qss.open(QFile::ReadOnly)){
qApp->setStyleSheet(qss.readAll());
} else {
qDebug()<<qss.error();
qDebug()<<qss.errorString();
}
m_mainWindow = new MainWindow();
m_mainWindow->setStyleSheet("MainWindow#w{border: 1px solid rgb(9,69,117)");
m_mainWindow->showMaximized();
//-----------------------------------------------
//-----------------初始化ice-------------------------
//绑定代理(需要处理服务器未打开时的异常)
try{
m_server = ServerPrx::checkedCast(communicator()->propertyToProxy("Hello.Proxy"));
//设置回调适配器
Ice::ObjectAdapterPtr adapter = communicator()->createObjectAdapter("Callback.Client");
LogCallbackReceiverPtr cr = new LogCallbackReceiverI;
adapter->add(cr, communicator()->stringToIdentity("LogCallbackReceiver"));
adapter->activate();
m_receiver = LogCallbackReceiverPrx::uncheckedCast(
adapter->createProxy(communicator()->stringToIdentity("LogCallbackReceiver")));
}
catch(const Ice::Exception& ex)
{
cerr << ex << endl;
}
return a.exec();
}
在run函数中创建QtGui线程并创建主窗口,并最后返回Qt的循环机制。函数中还绑定了回调函数,以便服务端返回状态。以下是配置文件:
Config.Client:
Hello.Proxy=hello:tcp -h 127.0.0.1 -p 10001
Callback.Client.Endpoints=default
Ice.IPv6=0
Ice.Override.Timeout=10000
Ice.ThreadPool.Client.Size=5
Ice.ThreadPool.Client.SizeMax=10
该配置文件是设置通信的IP地址、端口和连接数等参数。
需要使用Ice框架,需要引入对应的lib,在pro文件中增加如下几行:
CONFIG(debug, debug | release){
LIBS += -L../lib -liced -liceutild
}else{
LIBS += -L../lib -lice -liceutil
}
INCLUDEPATH +=
../include/ice
读者可以根据头文件和lib存放路径自行修改对应引入路径,并将相应的dll文件拷贝到相应目录下。笔者在实际验证的过程中,此lib、dll库需要跟编译该Qt项目编译器一致,不然会导致不可预料的错误,崩在ice里。笔者验证的在Qt creator中使用mvc12_64位的编译器,引用的是自己通过mvc12编译的ice库,下面给出下载地址:
扫描二维码关注公众号,回复:
5289641 查看本文章
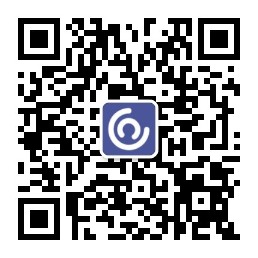
接口文件中定义了回调函数和其它需要用到的接口,如下:
#pragma once
module DeviceServer
{
//回调类
interface LogCallbackReceiver
{
//回调方法
//lotType 日志类型
//msg 日志内容
void WaiteCaptureEnd(LogEnum lotType, string msg);
};
interface Server
{
//接口xx
void test(string testName,LogCallbackReceiver* cReceiver, out string idn);
//接口xxx
........
};
};
完成接口文件后,需要使用slice2cpp.exe转换为C++文件,并在MicrowaveClient.cpp或者MicrowaveClient.h中引用。