题目内容:
给定一个整数数组 nums
和一个目标值 target
,请你在该数组中找出和为目标值的那 两个 整数,并返回他们的数组下标。
你可以假设每种输入只会对应一个答案。但是,你不能重复利用这个数组中同样的元素。
示例:
- Input:
nums = [2, 7, 11, 15], target = 9
- Output:
[0, 1]
实现算法:
(一)、暴力解决:
时间复杂度:O(n^2) 空间复杂度:O(1)
直接嵌套两层循环实现:
class TowSumSolution{
public int[] twoSum(int[] nums, int target) {
for (int i = 0; i < nums.length; i++) {
for (int j = i + 1; j < nums.length; j++) {
if (nums[i] + nums[j] == target) {
return new int[] { i, j };
}
}
}
throw new IllegalArgumentException("No two sum solution");
}
}
(二)、两遍Hashmap:
时间复杂度:O(n) 空间复杂度:O(n)
扫描二维码关注公众号,回复:
5288114 查看本文章
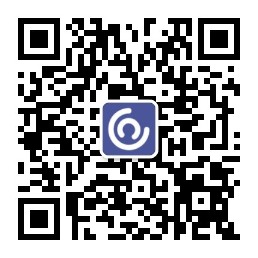
先进行第一次循环,将所有数组元素放入哈希表中;
第二次循环在哈希表中进行查找出能与第 i 个数组元素相加等于target目标值数值的非自身数组元素。
public int[] twoSumSolution(int[] nums, int target) {
Map<Integer, Integer> map = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
map.put(nums[i], i);
}
for (int i = 0; i < nums.length; i++) {
int complement = target - nums[i];
if (map.containsKey(complement) && map.get(complement) != i) {
return new int[] { i, map.get(complement) };
}
}
throw new IllegalArgumentException("No two sum solution");
}
(三)、一遍Hashmap:
时间复杂度:O(n) 空间复杂度:O(n)
在取第 i 个数组元素出来时,求相加等于target数值的另一个数,之后在已知的哈希表中查询,若有查到则返回另个元素的位置,否则将该元素放入哈希表中,进行下一步第i+1个数组元素的查找,依此类推。
class TowSumSolution {
public int[] twoSum(int[] nums, int target) {
Map<Integer, Integer> map = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
int complement = target - nums[i];
if (map.containsKey(complement)) {
return new int[] { map.get(complement), i };
}
map.put(nums[i], i);
}
throw new IllegalArgumentException("No two sum solution");
}
}