threading 常见用法:
(1) 在 thread 中,我们是通过 thread.start_new_thread(function, args) 来开启一个线程,接收一个函数和该函数的参数,函数的参数必须是元组的形式
(2) 在 threading 中,我们是通过 threading.Thread(target=function, args=(....)) 来创建一个对象,然后再通过对象的 start() 方法来开启一个线程,多个线程可以并发执行
#!/usr/bin/env python #-*- coding:utf-8 -*- import time import threading def fun(): print time.ctime() time.sleep(1) for i in range(3): t = threading.Thread(target=fun, args=()) # 创建一个 Thread 对象,接收一个函数和该函数的参数 t.start() # 通过对象来开启一个新的线程,通过线程来执行函数
[root@localhost ~]$ python 1.py # 多个线程并发执行,只需要执行一秒 Mon Jan 28 21:15:35 2019 Mon Jan 28 21:15:35 2019 Mon Jan 28 21:15:35 2019
t.join()方法用于阻塞下一个线程的运行,等待当前线程执行完再执行下一个线程,例子如下:
#!/usr/bin/env python #-*- coding:utf-8 -*- import time import threading def fun(): print time.ctime() time.sleep(1) for i in range(3): t = threading.Thread(target=fun, args=()) t.start() t.join()
[root@localhost ~]$ python 1.py # 由于线程被阻塞,需要执行3秒 Mon Jan 28 21:33:35 2019 Mon Jan 28 21:33:36 2019 Mon Jan 28 21:33:37 2019
t.setDaemon()方法用于是否设置当前线程随着主线程的退出而退出,例子如下:
#!/usr/bin/env python #-*- coding:utf-8 -*- import time import threading def fun(): # 函数的功能是打印三次当前时间 for i in range(3):
print time.ctime() time.sleep(1) t = threading.Thread(target=fun, args=()) t.setDaemon(True) t.start()
print 'End...' # 执行 print 之后,主线程就退出了,最终结果只会打印一次
[root@localhost ~]$ python 1.py Mon Jan 28 21:54:48 2019
End...
扫描二维码关注公众号,回复:
5152132 查看本文章
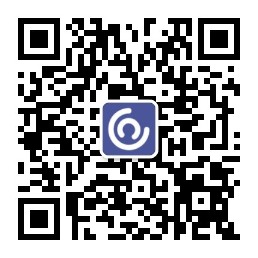