版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/qq_36533951/article/details/79144590
如何整合的?
其实就是Spring中提供了一个可以操作数据库的对象,对象封装了jdbc技术
JDBCTemplate jdbc模板对象
具体用法其实和DBUtils一样
直接放案例
bean
public class User {
private Integer id ;
private String name ;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
接口
*/
public interface UserDao {
//增
void save ( User u ) ;
//删除
void delete (Integer id ) ;
//改
void update ( User u ) ;
//查
User getById ( Integer id ) ;
//查
int getTotalCount () ;
//查
List <User> getAll () ;
}
实现
基本包含dao常用操作
public class UserDaoImpl implements UserDao {
private JdbcTemplate jt ;
public JdbcTemplate getJt() {
return jt;
}
public void setJt(JdbcTemplate jt) {
this.jt = jt;
}
/*
* @see com.study.spring.UserDao#save(com.study.spring.User)
*/
@Override
public void save(User u) {
String sql = "insert into user values (null , ?)" ;
jt.update(sql, u.getName()) ;
}
/*
* @see com.study.spring.UserDao#delete(java.lang.Integer)
*/
@Override
public void delete(Integer id) {
String sql = "delete from user where id = ?" ;
jt.update(sql, id) ;
}
/*
* @see com.study.spring.UserDao#update(com.study.spring.User)
*/
@Override
public void update(User u) {
String sql = "update user set name = ? where id = ?" ;
jt.update(sql, u.getName() , u.getId()) ;
}
/*
* @see com.study.spring.UserDao#getById(java.lang.Integer)
*/
@Override
public User getById(Integer id) {
String sql = "select * from User where id = ?" ;
return jt.queryForObject(sql, new RowMapper <User> ()
{
@Override //result 里存放每次遍历的结果 args 存放索引
public User mapRow(ResultSet result , int args ) throws SQLException {
User user = new User () ;
user.setId( result.getInt("id") );
user.setName( result.getString("name") );
return user ;
}
} , id ) ;
}
/*
* @see com.study.spring.UserDao#getTotalCount()
*/
@Override
public int getTotalCount() {
String sql = "select count(*) from user" ;
//当查询结果是值类型可以使用此方法,要手动指定值类型
return jt.queryForObject( sql , Integer.class ) ;
}
/*
* @see com.study.spring.UserDao#getAll()
*/
@Override
public List<User> getAll() {
String sql = "select * from user " ;
List <User> list = jt.query( sql , new RowMapper <User> ()
{
@Override
public User mapRow(ResultSet result, int args) throws SQLException {
User user = new User () ;
user.setId( result.getInt("id") );
user.setName( result.getString("name") );
return user ;
}
}) ;
return list ;
}
}
xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<!-- 将数据库连接池交由Spring管理 -->
<bean name="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource" >
<property name="jdbcUrl" value="jdbc:mysql:///Demo"></property>
<property name="driverClass" value="com.mysql.jdbc.Driver"></property>
<property name="user" value="root"></property>
<property name="password" value="zh123456"></property>
</bean>
<!-- jdbcTemplate -->
<bean name="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="dataSource"></property>
</bean>
<!-- dao -->
<bean name="userDao" class="com.study.spring.UserDaoImpl">
<property name="jt" ref="jdbcTemplate"></property>
</bean>
</beans>
接下来介绍一个扩招 JDBCDaoSupport
继承此类后,一个类可以从其父类获得JDBCTemplate对象(父类根据数据库连接池类型创建的)
public class UserDaoImpl extends JdbcDaoSupport implements UserDao {
/*
* @see com.study.spring.UserDao#save(com.study.spring.User)
*/
@Override
public void save(User u) {
String sql = "insert into user values (null , ?)" ;
super.getJdbcTemplate().update(sql, u.getName()) ;
}
/*
* @see com.study.spring.UserDao#delete(java.lang.Integer)
*/
@Override
public void delete(Integer id) {
String sql = "delete from user where id = ?" ;
super.getJdbcTemplate().update(sql, id) ;
}
/*
* @see com.study.spring.UserDao#update(com.study.spring.User)
*/
@Override
public void update(User u) {
String sql = "update user set name = ? where id = ?" ;
super.getJdbcTemplate().update(sql, u.getName() , u.getId()) ;
}
/*
* @see com.study.spring.UserDao#getById(java.lang.Integer)
*/
@Override
public User getById(Integer id) {
String sql = "select * from User where id = ?" ;
return super.getJdbcTemplate().queryForObject(sql, new RowMapper <User> ()
{
@Override //result 里存放每次遍历的结果 args 存放索引
public User mapRow(ResultSet result , int args ) throws SQLException {
User user = new User () ;
user.setId( result.getInt("id") );
user.setName( result.getString("name") );
return user ;
}
} , id ) ;
}
/*
* @see com.study.spring.UserDao#getTotalCount()
*/
@Override
public int getTotalCount() {
String sql = "select count(*) from user" ;
//当查询结果是值类型可以使用此方法,要手动指定值类型
return super.getJdbcTemplate().queryForObject( sql , Integer.class ) ;
}
/*
* @see com.study.spring.UserDao#getAll()
*/
@Override
public List<User> getAll() {
String sql = "select * from user " ;
List <User> list = super.getJdbcTemplate().query( sql , new RowMapper <User> ()
{
@Override
public User mapRow(ResultSet result, int args) throws SQLException {
User user = new User () ;
user.setId( result.getInt("id") );
user.setName( result.getString("name") );
return user ;
}
}) ;
return list ;
}
}
xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<!-- 将数据库连接池交由Spring管理 -->
<bean name="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource" >
<property name="jdbcUrl" value="jdbc:mysql:///Demo"></property>
<property name="driverClass" value="com.mysql.jdbc.Driver"></property>
<property name="user" value="root"></property>
<property name="password" value="zh123456"></property>
</bean>
<!-- dao -->
<bean name="userDao" class="com.study.spring.UserDaoImpl">
<property name="dataSource" ref="dataSource"></property>
</bean>
</beans>
读取properties配置
有时候数据库链接地址 用户 密码经常来回变动,一变动就要从Spring配置文件中找到此配置在修改,比较麻烦,所以为了方便修改可以将这些信息放到properties文件中
写properties文件时建议在key前面加上前缀,以免和Spring中其他关键字冲突(不想写也行)
注意:properties中不要有空格,否则运行慢+报错!!!
properties
扫描二维码关注公众号,回复:
5150669 查看本文章
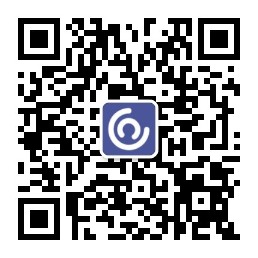
jdbcUrl=jdbc:mysql:///Demo
driverClass=com.mysql.jdbc.Driver
user=root
password=xxxxxxxx
用${key}取值
bean.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<!-- 指定Spring读取DB.properties配置 -->
<context:property-placeholder location="classpath:DB.properties"/>
<!-- 将数据库连接池交由Spring管理 -->
<bean name="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource" >
<property name="jdbcUrl" value="${jdbcUrl}"></property>
<property name="driverClass" value="${driverClass}"></property>
<property name="user" value="${user}"></property>
<property name="password" value="${password}"></property>
</bean>
<!-- dao -->
<bean name="userDao" class="com.study.spring.UserDaoImpl">
<property name="dataSource" ref="dataSource"></property>
</bean>
</beans>