我这次创建的是一个叫做student的表,里面存有id、name和age字段,我将搭建一个hibernate环境,利用框架的核心API对student进行数据插入。数据库用的是Mysql。
1.首先在工程中添加hibernate的jar包:
将解压路径中hibernate3.jar和lib路径下的required、jpa子目录下所有JAR包添加到应用的类加载路径中。
hibernate的帮助文档在
hibernate-distribution-3.6.0.Final\documentation\manual\zh-CN\html_single下的index.html
2..编写实体类的JavaBean(cn.edu.hpu.model包下)
package cn.edu.hpu.model;
public class Student {
private int id;
private String name;
private int age;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
3.配置实体类的hbm映射文件:
Student.hbm.xml:
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<hibernate-mapping package="cn.edu.hpu.model">
<class name="Student" table="stu">
<!-- 主键 这里的主键名字刚好也是'id'-->
<id name="id" column="id"></id>
<property name="name"></property>
<property name="age"></property>
</class>
</hibernate-mapping>
4.在src目录下hibernate的配置配置文件,即数据库连接池hibernate.cfg.xml:
<?xml version='1.0' encoding='utf-8'?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!-- Database connection settings -->
<property name="connection.driver_class">org.gjt.mm.mysql.Driver</property>
<property name="connection.url">jdbc:mysql://localhost:3306/students</property>
<property name="connection.username">root</property>
<property name="connection.password">1234</property>
<!-- JDBC connection pool (use the built-in) JDBC连接池-->
<!-- <property name="connection.pool_size">1</property> -->
<!-- SQL dialect SQL方言-->
<property name="dialect">org.hibernate.dialect.MySQLDialect</property>
<!-- Enable Hibernate's automatic session context management -->
<!-- <property name="current_session_context_class">thread</property> -->
<!-- Disable the second-level cache 去掉二级缓存-->
<property name="cache.provider_class">org.hibernate.cache.NoCacheProvider</property>
<!-- Echo all executed SQL to stdout 是否打印sql语句-->
<property name="show_sql">true</property>
<!-- Drop and re-create the database schema on startup 是否自动生成建表语句语句-->
<property name="hbm2ddl.auto">update</property>
<mapping resource="cn/edu/hpu/model/Student.hbm.xml"/>
</session-factory>
</hibernate-configuration>
5.编写测试类:
package cn.edu.hpu.test;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
import cn.edu.hpu.model.Student;
public class StudentTest {
public static void main(String[] args) {
Student s=new Student();
s.setId(1);
s.setName("s1");
s.setAge(1);
//Configuration是用来读配置文件的
Configuration cfg=new Configuration();
//指定要读哪个文件,不写参数默认读hibernate配置
//文件下的 <mapping resource=.....
SessionFactory sf=cfg.configure().buildSessionFactory();
//buildSessionFactory()可以理解为能得到conn的类
//暂时把Session理解成数据库的Connection
Session session=sf.openSession();//得到session
session.beginTransaction();
session.save(s);
session.getTransaction().commit();
session.close();
sf.close();
}
}
查看MySql数据库,发现已经存在一张新的表stu,并且里面拥有一个id为1,name为s1,age为1的记录。
扫描二维码关注公众号,回复:
5113405 查看本文章
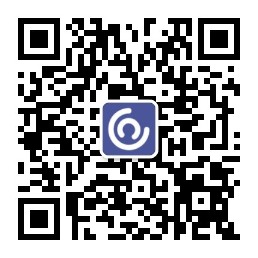
这个小例子向大家叙述了如何搭建一个hibernate的环境,以及利用session来对实体数据进行简单的操作。