功能
- 目标文件存在时给出“是否覆盖”,“是否合并”等提示信息,用户可以选择覆盖目标文件或者将已经存在的目标文件和源文件实现合并(在目标文件的尾部实现追加写入)
- 源文件不存在时给出错误提示信息
- 源文件是目录时给出错误提示信息
源码
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <string.h>
#include <errno.h>
#include <sys/stat.h>
#define BUF_SIZE 1024
void copy (int srcFd, int tarFd) {
ssize_t ret;
char buf[BUF_SIZE];
while ((ret = read (srcFd, buf, BUF_SIZE)) > 0) {
if ((ret = write (tarFd, buf, ret)) == -1)
break;
}
if (ret == -1) {
printf ("exception on copy file: %s\n", strerror (errno));
}
}
int main (int argc, char **argv) {
if (argc != 3) {
printf ("usage: source-path target-path\n");
exit (0);
}
char *srcPath = argv[1], *tarPath = argv[2];
int flag = O_WRONLY | O_CREAT;
struct stat s_buf;
// 判断源路径是否存在且可读
if (access (srcPath, F_OK | R_OK) == -1) {
printf ("%s: %s\n", strerror (errno), srcPath);
exit (-1);
}
else {
stat (srcPath, &s_buf);
// 判断源路径是否是目录
if (S_ISDIR (s_buf.st_mode)) {
printf ("source is not a file: %s\n", srcPath);
exit (-1);
}
}
// 判断目标路径是否存在且可写
if (access (tarPath, F_OK | W_OK) != -1) {
// 目标路径已存在且可写
char c;
while (1) {
printf ("target file exists: %s\n", tarPath);
printf ("1 - overwrite\n");
printf ("2 - merge\n");
printf ("3 - quit\n");
c = getchar ();
if (c == '1') {
flag |= O_TRUNC;
break;
}
else if (c == '2') {
flag |= O_APPEND;
break;
}
else if (c == '3')
exit (0);
}
}
else if (errno != ENOENT) {
printf ("%s: %s\n", strerror (errno), tarPath);
exit (-1);
}
// 打开文件描述符,进行copy
int srcFd = open (srcPath, O_RDONLY);
int tarFd = open (tarPath, flag, 0666);
copy (srcFd, tarFd);
close (srcFd);
close (tarFd);
}
运行示例
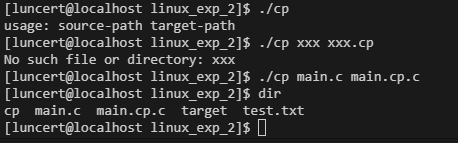