生产者–消费者(–仓库)模式
-
此模式脱离仓库没有意义
-
(1)仓库用来存储数据
(2)仓库不满,生产
(3)仓库满足消费者要求时,消费
/**
* 仓库类型
*/
public class Depot{
private static final int MAX_NUM = 100;
private int num;
public Depot(int num) {
this.num = num;
}
/**消费方法*/
public synchronized void consume(int need){
while(need>num){
try {
this.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("满足需求,可以购买"+need);
num-=need;
System.out.println("购买成功,仓库剩余:"+num);
this.notifyAll();
}
/**生产方法*/
public synchronized void product(int pro){
while(pro+num>MAX_NUM){
try {
System.out.println("仓库空余位置不足,不能生产");
this.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("生产数量:"+pro);
num+=pro;
System.out.println("生产完毕,仓库剩余"+num);
this.notifyAll();
}
public static void main(String[] args) {
Depot depot = new Depot(30);
Consumtor c1 = new Consumtor(depot, "小A", 40);
Consumtor c2 = new Consumtor(depot, "小B", 50);
Consumtor c3 = new Consumtor(depot, "小C", 60);
Consumtor c4 = new Consumtor(depot, "小D", 45);
Productor p1 = new Productor(depot, "x1", 30);
Productor p2 = new Productor(depot, "x2", 50);
Productor p3 = new Productor(depot, "x3", 70);
Productor p4 = new Productor(depot, "x4", 40);
Productor p5 = new Productor(depot, "x5", 60);
c1.start();
c2.start();
c3.start();
c4.start();
p1.start();
p2.start();
p3.start();
p4.start();
p5.start();
}
}
/**
* 生产者
* */
class Productor extends Thread{
private Depot depot;
private String name;
private int pro;
public Productor(Depot depot, String name, int pro) {
super();
this.depot = depot;
this.name = name;
this.pro = pro;
}
public void run(){
depot.product(pro);
}
}
/**
* 消费者
* */
class Consumtor extends Thread{
private Depot depot;
private String name;
private int need;
public Consumtor(Depot depot, String name, int need) {
super();
this.depot = depot;
this.name = name;
this.need = need;
}
public void run(){
depot.consume(need);
}
}
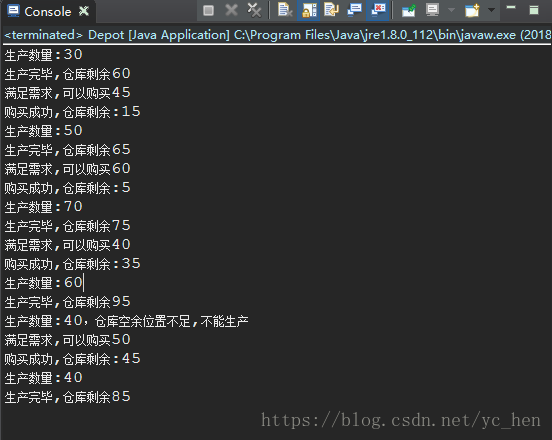