线程的生命周期及五种基本状态
线程中断处理
1.while(!interrupted())
2.td.interrupt()
创建线程的几种方式
1.继承Thread类
public class Demo1 extends Thread {
public Demo1(String name) {
super(name);
}
@Override
public void run() {
System.out.println(getName() + "线程执行了 .. ");//打印线程名称
}
public static void main(String[] args) {
Demo1 d1 = new Demo1("first-thread");
d1.start();
}
}
2.实现Runnable接口
public class Demo2 implements Runnable {
@Override
public void run() {
while(true) {
System.out.println("thread running ...");
}
}
public static void main(String[] args) {
Thread thread = new Thread(new Demo2());
thread.start();
}
}
3.匿名内部类的方式
public class Demo3 {
public static void main(String[] args) {
new Thread() {
public void run() {
System.out.println("thread start ..");
};
}.start();
new Thread(new Runnable() {
@Override
public void run() {
System.out.println("thread start ..");
}
}).start();
//最终打印出sub,因为之类重写了父类的方法
new Thread(new Runnable() {
@Override
public void run() {
System.out.println("runnable");
}
}) {
public void run() {
System.out.println("sub");
};
}.start();
}
}
4.通过 Callable 和 Future 创建线程(带返回值的线程)
import java.util.concurrent.Callable;
import java.util.concurrent.FutureTask;
public class Demo4 implements Callable<Integer> {
public static void main(String[] args) throws Exception {
Demo4 d = new Demo4();
//预先执行
FutureTask<Integer> task = new FutureTask<>(d);
Thread t = new Thread(task);
t.start();
System.out.println("我先干点别的。。。");
Integer result = task.get();
System.out.println("线程执行的结果为:" + result);
}
@Override
public Integer call() throws Exception {
System.out.println("正在进行紧张的计算....");
Thread.sleep(3000);
return 1;
}
}
5.定时器
import java.util.Timer;
import java.util.TimerTask;
public class Demo5 {
public static void main(String[] args) {
Timer timer = new Timer();
timer.schedule(new TimerTask() {
@Override
public void run() {
// 实现定时任务
System.out.println("timertask is run");
}
}, 0, 1000);
}
}
6.线程池的方式
public class Demo6 {
public static void main(String[] args) {
ExecutorService threadPool = Executors.newCachedThreadPool();
for (int i = 0; i < 1000; i++) {
threadPool.execute(new Runnable() {
@Override
public void run() {
System.out.println(Thread.currentThread().getName());
}
});
}
threadPool.shutdown();
}
}
7.Lambda表达式实现
import java.util.Arrays;
import java.util.List;
public class Demo7 {
public static void main(String[] args) {
List<Integer> values = Arrays.asList(10,20,30,40);
int res = new Demo7().add(values);
System.out.println("计算的结果为:" + res);
}
//从集合中读取数据并进行计算
public int add (List<Integer> values) {
//parallelStream 是一个并行的流,stream是一个串行的流
//values.parallelStream().forEach(System.out :: println);
//values.stream().forEach(System.out :: println);
//mapToInt把并行的流转换成一个整型的流
//i -> i * 2名称和值
return values.parallelStream().mapToInt( i -> i * 2).sum();
}
}
8.Spring多线程实现
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
@Service
public class ThreadTest {
@Async
public void a() {
while (true) {
System.out.println("a");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
@Async
public void b() {
while (true) {
System.out.println("b");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
扫描二维码关注公众号,回复:
5022100 查看本文章
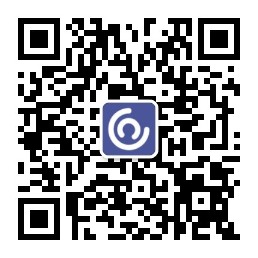