1. EventBus 简单使用
EventBus的使用实践已经在上一篇的博客中讲述了,可查阅EventBus—使用实践。
在此只简单列举EventBus的使用:
1.1. 定义事件
public class TextEvent {
private String mText;
public TextEvent(String text) {
this.mText = text;
}
public String getText() {
return mText;
}
public void setText(String text) {
this.mText = text;
}
}
1.2. 注册与解注册
@Override
protected void onStart() {
super.onStart();
EventBus.getDefault().register(this);
}
@Override
protected void onStop() {
super.onStop();
EventBus.getDefault().unregister(this);
}
1.3. 发布事件
EventBus.getDefault().post(new TextEvent("From MainActivity text event"));
1.4. 接收事件
@Subscribe
public void onHandleTextEvent(TextEvent event){
mTvResult.setText(event.getText());
}
2. 源码解析
在解析EventBus源码之前,想想几个问题:
- 通过简单的几行代码,EventBus是如何找到订阅者的?
- 粘性事件是怎么实现的?
- EventBus是如何实现切换线程的?
EventBus所有的秘密都藏在EventBus.getDefault().register(this)方法里面,先看看Event类关系图:
其中有几个非常重要的数据结构需要注意,EventBus事件分发与处理都是围绕着它们进行处理:
- subscriptionByEventType:事件与订阅信息的映射关系表,一般为一对多的关系;
- typesBySubscriber:订阅者与事件的映射关系表,
- stickyEvents:粘性事件存储表,key为事件class对象,value为粘性事件对象;
- subscriberMethodFinder:算法核心类,找寻与解析订阅者的所有订阅方法并封装成SubscriberMethod返回;
- Poster子类:切换不同线程执行订阅方法的辅助类;
2.1. 注册(register)
EventBus用了典型的单例模式,先看看它的register方法做了些什么事情:
EventBus:
public void register(Object subscriber) {
Class<?> subscriberClass = subscriber.getClass();
// 获取订阅者的订阅方法并封装成SubscriberMethod返回
List<SubscriberMethod> subscriberMethods = subscriberMethodFinder.findSubscriberMethods(subscriberClass);
synchronized (this) {
for (SubscriberMethod subscriberMethod : subscriberMethods) {
// 将订阅者以及订阅方法添加到相应的映射表中
subscribe(subscriber, subscriberMethod);
}
}
}
SubscriberMethodFinder.findSubscriberMethods()方法中有两个方法可以获取subscriberMethods,分别通过反射方法或索引加速方法获取,可通过ignoreGeneratedIndex配置修改相应的策略,默认为false。其中索引加速在第三节讲述,通过开启索引加速,极大地提升了EventBus的运行效率。反射获取订阅方法就不讲述了,较简单。
SubscriberMethodFinder:
List<SubscriberMethod> findSubscriberMethods(Class<?> subscriberClass) {
List<SubscriberMethod> subscriberMethods = METHOD_CACHE.get(subscriberClass);
if (subscriberMethods != null) {
return subscriberMethods;
}
if (ignoreGeneratedIndex) {
// 反射策略方法获取订阅方法
subscriberMethods = findUsingReflection(subscriberClass);
} else {
// 索引加速方法策略,若启用索引加速,则用索引加速策略,否则最终
// 也会使用反射方法获取
subscriberMethods = findUsingInfo(subscriberClass);
}
if (subscriberMethods.isEmpty()) {
// 如果订阅者没有订阅方法,即@Subscribe注解标注的方法,则会抛异常
throw new EventBusException("Subscriber " + subscriberClass
+ " and its super classes have no public methods with the @Subscribe annotation");
} else {
METHOD_CACHE.put(subscriberClass, subscriberMethods);
return subscriberMethods;
}
}
private List<SubscriberMethod> findUsingInfo(Class<?> subscriberClass) {
FindState findState = prepareFindState();
findState.initForSubscriber(subscriberClass);
while (findState.clazz != null) {
// 通过索引加速策略获取subscriberInfo
findState.subscriberInfo = getSubscriberInfo(findState);
if (findState.subscriberInfo != null) {
SubscriberMethod[] array = findState.subscriberInfo.getSubscriberMethods();
for (SubscriberMethod subscriberMethod : array) {
if (findState.checkAdd(subscriberMethod.method, subscriberMethod.eventType)) {
findState.subscriberMethods.add(subscriberMethod);
}
}
} else {
// 若未启用索引加速。则使用反射方法获取
findUsingReflectionInSingleClass(findState);
}
findState.moveToSuperclass();
}
return getMethodsAndRelease(findState);
}
private SubscriberInfo getSubscriberInfo(FindState findState) {
if (findState.subscriberInfo != null && findState.subscriberInfo.getSuperSubscriberInfo() != null) {
SubscriberInfo superclassInfo = findState.subscriberInfo.getSuperSubscriberInfo();
if (findState.clazz == superclassInfo.getSubscriberClass()) {
return superclassInfo;
}
}
// 启动索引加速后,subscriberInfoIndexes不为null,并使用其直接获取SubscriberInfo
if (subscriberInfoIndexes != null) {
for (SubscriberInfoIndex index : subscriberInfoIndexes) {
SubscriberInfo info = index.getSubscriberInfo(findState.clazz);
if (info != null) {
return info;
}
}
}
return null;
}
再回到EventBus的register方法中调用的subscribe(subscriber, subscriberMethod)方法:
EventBus:
private void subscribe(Object subscriber, SubscriberMethod subscriberMethod) {
Class<?> eventType = subscriberMethod.eventType;
// 封装订阅信息Subscription,订阅者以及其其中一个订阅方法
Subscription newSubscription = new Subscription(subscriber, subscriberMethod);
CopyOnWriteArrayList<Subscription> subscriptions = subscriptionsByEventType.get(eventType);
// 获取事件与订阅的映射关系表中的订阅信息列表,若无,则重新创建一个列表
// 事件与订阅者是一对多的关系
if (subscriptions == null) {
subscriptions = new CopyOnWriteArrayList<>();
subscriptionsByEventType.put(eventType, subscriptions);
} else {
if (subscriptions.contains(newSubscription)) {
throw new EventBusException("Subscriber " + subscriber.getClass() + " already registered to event "
+ eventType);
}
}
// 将封装的订阅信息根据优先级添加到关系映射表中
int size = subscriptions.size();
for (int i = 0; i <= size; i++) {
if (i == size || subscriberMethod.priority > subscriptions.get(i).subscriberMethod.priority) {
subscriptions.add(i, newSubscription);
break;
}
}
// 构建与添加 订阅者与事件的关系并添加到subscribedEvents映射表中
List<Class<?>> subscribedEvents = typesBySubscriber.get(subscriber);
if (subscribedEvents == null) {
subscribedEvents = new ArrayList<>();
typesBySubscriber.put(subscriber, subscribedEvents);
}
subscribedEvents.add(eventType);
// 若监听了粘性事,则执行粘性事件
if (subscriberMethod.sticky) {
if (eventInheritance) {
// Existing sticky events of all subclasses of eventType have to be considered.
// Note: Iterating over all events may be inefficient with lots of sticky events,
// thus data structure should be changed to allow a more efficient lookup
// (e.g. an additional map storing sub classes of super
classes: Class -> List<Class>).
// 遍历粘性事件的列表,并执行其中匹配的粘性事件
Set<Map.Entry<Class<?>, Object>> entries = stickyEvents.entrySet();
for (Map.Entry<Class<?>, Object> entry : entries) {
Class<?> candidateEventType = entry.getKey();
if (eventType.isAssignableFrom(candidateEventType)) {
Object stickyEvent = entry.getValue();
checkPostStickyEventToSubscription(newSubscription, stickyEvent);
}
}
} else {
Object stickyEvent = stickyEvents.get(eventType);
checkPostStickyEventToSubscription(newSubscription, stickyEvent);
}
}
}
小结:
EventBus注册主要做了一下几件事:
- 通过SubscriberMethodFinder根据订阅者class查找相应的订阅方法;
- 遍历每个订阅方法,把订阅者和订阅方法构建成Subscription;
- 根据优先级将Subscription添加到subscriptionsByEventType(即使用EventBus时可通过priority配置事件的处理优先级)
- 把订阅者及其对应订阅方法添加到typesBySubscriber;
- 执行粘性事件(重点)
2.2. 发布事件(Post)
发布事件其实是基于上述的几个映射表,获取订阅者的订阅方法,并利用反射执行订阅方法,并使用了消费者与生产者模式:
EventBus:
/** Posts the given event to the event bus. */
public void post(Object event) {
// 获取当前线程的事件队列,并将新来的事件插入置队列中
PostingThreadState postingState = currentPostingThreadState.get();
List<Object> eventQueue = postingState.eventQueue;
eventQueue.add(event);
if (!postingState.isPosting) {
postingState.isMainThread = isMainThread();
postingState.isPosting = true;
if (postingState.canceled) {
throw new EventBusException("Internal error. Abort state was not reset");
}
try {
// 不断分发事件,直至为空
while (!eventQueue.isEmpty()) {
postSingleEvent(eventQueue.remove(0), postingState);
}
} finally {
postingState.isPosting = false;
postingState.isMainThread = false;
}
}
}
private void postSingleEvent(Object event, PostingThreadState postingState) throws Error {
Class<?> eventClass = event.getClass();
boolean subscriptionFound = false;
if (eventInheritance) {
List<Class<?>> eventTypes = lookupAllEventTypes(eventClass);
int countTypes = eventTypes.size();
for (int h = 0; h < countTypes; h++) {
Class<?> clazz = eventTypes.get(h);
// 发布事件
subscriptionFound |= postSingleEventForEventType(event, postingState, clazz);
}
} else {
subscriptionFound = postSingleEventForEventType(event, postingState, eventClass);
}
// 若无订阅者订阅该事件,则会发布NoSubscriberEvent事件
if (!subscriptionFound) {
if (logNoSubscriberMessages) {
logger.log(Level.FINE, "No subscribers registered for event " + eventClass);
}
if (sendNoSubscriberEvent && eventClass != NoSubscriberEvent.class &&
eventClass != SubscriberExceptionEvent.class) {
post(new NoSubscriberEvent(this, event));
}
}
}
private boolean postSingleEventForEventType(Object event, PostingThreadState postingState, Class<?> eventClass) {
CopyOnWriteArrayList<Subscription> subscriptions;
synchronized (this) {
// 关键点:通过subscriptionsByEventType映射列表,获取订阅者信息
subscriptions = subscriptionsByEventType.get(eventClass);
}
if (subscriptions != null && !subscriptions.isEmpty()) {
// 遍历所有的订阅者并执行该事件的订阅方法
for (Subscription subscription : subscriptions) {
postingState.event = event;
postingState.subscription = subscription;
boolean aborted = false;
try {
// 执行订阅者的方法
postToSubscription(subscription, event, postingState.isMainThread);
aborted = postingState.canceled;
} finally {
postingState.event = null;
postingState.subscription = null;
postingState.canceled = false;
}
if (aborted) {
break;
}
}
return true;
}
return false;
}
private void postToSubscription(Subscription subscription, Object event, boolean isMainThread) {
// 通过订阅方法的线程模式,使用不同的策略执行订阅方法,通过HandlerPoster
// AsyncPoster,BackgroundPoster执行不同的线程策略,比如后台线程、主线程等,AsyncPoster等内部使用了生产者和消费者的模式
switch (subscription.subscriberMethod.threadMode) {
case POSTING:
invokeSubscriber(subscription, event);
break;
case MAIN:
if (isMainThread) {
invokeSubscriber(subscription, event);
} else {
mainThreadPoster.enqueue(subscription, event);
}
break;
case MAIN_ORDERED:
if (mainThreadPoster != null) {
mainThreadPoster.enqueue(subscription, event);
} else {
// temporary: technically not correct as poster not decoupled from subscriber
invokeSubscriber(subscription, event);
}
break;
case BACKGROUND:
if (isMainThread) {
backgroundPoster.enqueue(subscription, event);
} else {
invokeSubscriber(subscription, event);
}
break;
case ASYNC:
asyncPoster.enqueue(subscription, event);
break;
default:
throw new IllegalStateException("Unknown thread mode: " + subscription.subscriberMethod.threadMode);
}
}
小结:
分发事件主要做了以下几件事情:
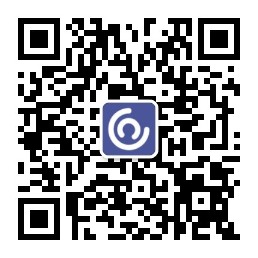
- 把事件插入到当前线程的事件队列中,不断遍历发布事件队列中的事件;
- 通过subscriptionsByEventType获取每个事件的订阅者的方法;
- 根据ThreadMode,并依赖HandlerPoster,AsyncPoster,BackgroundPoster执行不同的线程策略;
通过上述的分析,已经完整地解析上述的三个问题:发布、粘性事件以及线程模式。
2.3. 解注册(unregister)
EventBus:
/** Unregisters the given subscriber from all event classes. */
public synchronized void unregister(Object subscriber) {
//
List<Class<?>> subscribedTypes = typesBySubscriber.get(subscriber);
if (subscribedTypes != null) {
for (Class<?> eventType : subscribedTypes) {
unsubscribeByEventType(subscriber, eventType);
}
typesBySubscriber.remove(subscriber);
} else {
logger.log(Level.WARNING, "Subscriber to unregister was not registered before: " + subscriber.getClass());
}
}
有了上面注册的过程,解注册就非常简单了,主要做了以下几件事件:
- 通过subscribedTypes获取该订阅者所有的订阅事件;(subscribedTypes映射列表的作用就是获取订阅者订阅的所有事件,方便解注册)
- 遍历所有事件,移除subscriptionsByEventType中的订阅者;
- 从subscribedTypes中移除该订阅者;
**注意:**注册和解注册必要成对存在,否则将出现内存泄漏。
3. 索引加速
为了提供性能,EventBus 3.0引入了索引加速的功能,大幅度提高的性能。启动索引加速需要做一些额外的配置,可参考EventBus—使用实践。其实索引加速功能,就是利用APT方式在编译时解析注解生成索引java文件,避免使用反射。
回顾上面的分析,整个流程中在SubscriberMethodFinder.findSubscriberMethods()检索订阅方法时会使用反射进行获取,这里会有性能的问题,那么索引加速在内存中直接加载避免使用反射获取。
使用索引加速时会在build目录下生成一个java文件,例如:
java文件的名称是在模块gradle中配置的
defaultConfig {
.....
javaCompileOptions {
annotationProcessorOptions {
arguments = [ eventBusIndex : 'com.wuzl.eventbus.TestEventBusIndex' ]
}
}
.....
}
看看编译生成的索引类,将所有订阅者及其订阅方法全部缓存至SUBSCRIBER_INDEX中,显然通过SUBSCRIBER_INDEX可以获取订阅者所有信息,而避免使用反射,那么接下来需要将索引文件配置进去。
TestEventBusIndex:
public class TestEventBusIndex implements SubscriberInfoIndex {
private static final Map<Class<?>, SubscriberInfo> SUBSCRIBER_INDEX;
static {
SUBSCRIBER_INDEX = new HashMap<Class<?>, SubscriberInfo>();
putIndex(new SimpleSubscriberInfo(MainActivity.class, true, new SubscriberMethodInfo[]{
new SubscriberMethodInfo("handleTextEvent", com.wuzl.eventbus.event.TextEvent.class),
}));
}
private static void putIndex(SubscriberInfo info) {
SUBSCRIBER_INDEX.put(info.getSubscriberClass(), info);
}
@Override
public SubscriberInfo getSubscriberInfo(Class<?> subscriberClass) {
SubscriberInfo info = SUBSCRIBER_INDEX.get(subscriberClass);
if (info != null) {
return info;
} else {
return null;
}
}
}
在使用索引加速的时候,需要将该索引文件配置到EventBus当中
EventBus.builder().addIndex(new TestEventBusIndex()).installDefaultEventBus();
EventBus:
/** Adds an index generated by EventBus' annotation preprocessor. */
public EventBusBuilder addIndex(SubscriberInfoIndex index) {
if (subscriberInfoIndexes == null) {
subscriberInfoIndexes = new ArrayList<>();
}
// 将索引加速类添加到subscriberInfoIndexes数据结构
subscriberInfoIndexes.add(index);
return this;
}
// 重新构建默认的EventBus
public EventBus installDefaultEventBus() {
synchronized (EventBus.class) {
if (EventBus.defaultInstance != null) {
throw new EventBusException("Default instance already exists." +
" It may be only set once before it's used the first time to ensure consistent behavior.");
}
// 重新new一个EventBus对象并设置为默认EventBus
EventBus.defaultInstance = build();
return EventBus.defaultInstance;
}
}
EventBus(EventBusBuilder builder) {
indexCount = builder.subscriberInfoIndexes != null ? builder.subscriberInfoIndexes.size() : 0;
// 将索引加速传递给SubscriberMethodFinder
subscriberMethodFinder = new SubscriberMethodFinder(builder.subscriberInfoIndexes,
builder.strictMethodVerification, builder.ignoreGeneratedIndex);
}
那么SubscriberMethodFinder在检索订阅者信息时,发现subscriberInfoIndexes不为null,将直接从内存中加载信息,而不走反射流程,极大地提高的性能。
SubscriberMethodFinder:
private SubscriberInfo getSubscriberInfo(FindState findState) {
....
if (subscriberInfoIndexes != null) {
for (SubscriberInfoIndex index : subscriberInfoIndexes) {
SubscriberInfo info = index.getSubscriberInfo(findState.clazz);
if (info != null) {
return info;
}
}
}
return null;
}
小结:
其实索引加速做的事情很简单,就是避免在findSubscriberMethods去调用耗时的反射机制。实现非常巧妙。
4. 总结
EventBus的源码解析中,发现非常多有用的设计思想,比如典型的单例模式、生成者与消费者模式、FindState的缓存机制以及巧妙的APT索引加速等等,非常值得学习与借鉴。