Just assuming that:
- There’s a Pet class and various subtypes of Pet .
- The static Pets.list() method returns an ArrayList filled with randomly selected Pet objects.
// collections/ListFeatures.java
// (c)2017 MindView LLC: see Copyright.txt
// We make no guarantees that this code is fit for any purpose.
// Visit http://OnJava8.com for more book information.
import java.util.*;
import typeinfo.pets.*;
public class ListFeatures {
public static void main(String[] args) {
Random rand = new Random(47);
List<Pet> pets = Pets.list(7);
System.out.println("1: " + pets);
Hamster h = new Hamster();
pets.add(h); // Automatically resizes
System.out.println("2: " + pets);
System.out.println("3: " + pets.contains(h));
pets.remove(h); // Remove by object
Pet p = pets.get(2);
System.out.println("4: " + p + " " + pets.indexOf(p));
Pet cymric = new Cymric();
System.out.println("5: " + pets.indexOf(cymric));
System.out.println("6: " + pets.remove(cymric)); // false
// Must be the exact object:
System.out.println("7: " + pets.remove(p));
System.out.println("8: " + pets);
pets.add(3, new Mouse()); // Insert at an index
System.out.println("9: " + pets);
List<Pet> sub = pets.subList(1, 4);
System.out.println("subList: " + sub);
System.out.println("10: " + pets.containsAll(sub));
Collections.sort(sub); // In-place sort
System.out.println("sorted subList: " + sub); // [1] note here output
System.out.println("sorted subList pets:" + pets); // [1] note here output
// Order is not important in containsAll():
System.out.println("11: " + pets.containsAll(sub));
Collections.shuffle(sub, rand); // Mix it up
System.out.println("shuffled subList: " + sub);
System.out.println("12: " + pets.containsAll(sub));
System.out.println("pets:" + pets); // [2] note here output diff with [1]
List<Pet> copy = new ArrayList<>(pets);
sub = Arrays.asList(pets.get(1), pets.get(4));
System.out.println("sub: " + sub);
copy.retainAll(sub);
System.out.println("13: " + copy);
copy = new ArrayList<>(pets); // Get a fresh copy
System.out.println("get new fresh copy:" + copy);
copy.remove(2); // Remove by index
System.out.println("14: " + copy);
copy.removeAll(sub); // Only removes exact objects // [3] note this code execute
System.out.println("15: " + copy);
copy.set(1, new Mouse()); // Replace an element
System.out.println("16: " + copy);
copy.addAll(2, sub); // Insert a list in the middle
System.out.println("17: " + copy);
System.out.println("18: " + pets.isEmpty());
pets.clear(); // Remove all elements
System.out.println("19: " + pets);
System.out.println("20: " + pets.isEmpty());
pets.addAll(Pets.list(4));
System.out.println("21: " + pets);
Object[] o = pets.toArray();
System.out.println("22: " + o[3]);
Pet[] pa = pets.toArray(new Pet[0]);
System.out.println("23: " + pa[3].id());
}
}
the above code result:
1: [Rat, Manx, Cymric, Mutt, Pug, Cymric, Pug]
2: [Rat, Manx, Cymric, Mutt, Pug, Cymric, Pug, Hamster]
3: true
4: Cymric 2
5: -1
6: false
7: true
8: [Rat, Manx, Mutt, Pug, Cymric, Pug]
9: [Rat, Manx, Mutt, Mouse, Pug, Cymric, Pug]
subList: [Manx, Mutt, Mouse]
10: true
sorted subList: [Manx, Mouse, Mutt] // [1]
sorted subList pets:[Rat, Manx, Mouse, Mutt, Pug, Cymric, Pug] // [1]
11: true
shuffled subList: [Mouse, Manx, Mutt]
12: true
pets:[Rat, Mouse, Manx, Mutt, Pug, Cymric, Pug] // [2]
sub: [Mouse, Pug]
13: [Mouse, Pug]
get new fresh copy:[Rat, Mouse, Manx, Mutt, Pug, Cymric, Pug]
14: [Rat, Mouse, Mutt, Pug, Cymric, Pug] // [3] do not remove the last Pug
15: [Rat, Mutt, Cymric, Pug]
16: [Rat, Mouse, Cymric, Pug]
17: [Rat, Mouse, Mouse, Pug, Cymric, Pug]
18: false
19: []
20: true
21: [Manx, Cymric, Rat, EgyptianMau]
22: EgyptianMau
23: 14
When deciding whether an element is part of a List, discovering the index of an element, and removing an element from a List by reference, the equals() method (part of the root class Object ) is used.
Be aware that List behavior changes depending on equals() behavior.
Output line 14 shows the result of removing an element using its index number, which is more straightforward than removing it by object reference since you don’t worry about equals() behavior when using indexes.
references:
1. On Java 8 - Bruce Eckel
2. https://github.com/wangbingfeng/OnJava8-Examples/blob/master/collections/ListFeatures.java
3. https://github.com/wangbingfeng/OnJava8-Examples/blob/master/typeinfo/pets/Pets.java
4. https://github.com/wangbingfeng/OnJava8-Examples/blob/master/typeinfo/pets/LiteralPetCreator.java
5. https://github.com/wangbingfeng/OnJava8-Examples/blob/master/typeinfo/pets/PetCreator.java
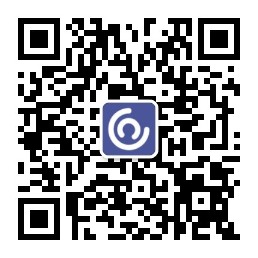