Implement strStr().
Return the index of the first occurrence of needle in haystack, or -1 if needle is not part of haystack.
Example 1:
Input: haystack = "hello", needle = "ll"
Output: 2
Example 2:
Input: haystack = "aaaaa", needle = "bba"
Output: -1
Clarification:
What should we return when needle
is an empty string? This is a great question to ask during an interview.
For the purpose of this problem, we will return 0 when needle
is an empty string. This is consistent to C's strstr() and Java's indexOf().
题目大意:寻找子字符串,以下是四种方法(重要性依次增加)
方法一:暴力法
class Solution {
public:
int strStr(string haystack, string needle) {
if(needle.length()==0)
return 0;
for(int i=0;i<haystack.size();i++){
for(int j=0;j<needle.length();j++){
if(i+j>=haystack.length()||haystack[i+j]!=needle[j])
break;
if(j==needle.length()-1)
return i;
}
}
return -1;
}
};
方法二:库函数find(偷懒hhhh)
class Solution {
public:
int strStr(string haystack, string needle) {
return haystack.find(needle);
}
};
方法三(经典算法):KMP
扫描二维码关注公众号,回复:
4907773 查看本文章
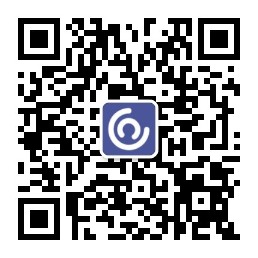
next数组开大一点哎~~
class Solution {
public:
int strStr(string haystack, string needle) {
int n=haystack.length(),m=needle.length();
if(m==0)
return 0;
if(n==0)
return -1;
vector<int>next(100005);
next[0]=-1;
int j=-1,i=0;
while(i<m){
if(j==-1||needle[j]==needle[i]){
++i;++j;
next[i]=j;
}
else
j=next[j];
}
i=0;j=0;
while(i<n&&j<m){
if(j==-1||haystack[i]==needle[j]){
++i;++j;
}
else
j=next[j];
}
if(j==m)
return i-j;
else
return -1;
}
};
KMP算法模板
#include <iostream>
#include <string>
#include <vector>
using namespace std;
string pat,txt;
int N,M;
std::vector<int> next;
int main(){
N=txt.length();M=pat.length();
next.resize(M);
getNext();
retrun KMP();
}
void getNext(){
next[0]=-1;
int j=-1,i=0;
while(i<M){
if(j==-1||pat[j]==pat[i]){
++i;++j;
next[i]=j;
}
else
j=next[j];
}
}
int KMP(){
int i=0,j=0;
while(i<N&&j<M){
if(j==-1||txt[i]==pat[j]){
++i;++j;
}
else
j=next[j];
}
if(j==M)
return i-j;
else
return -1;
}
方法四(经典算法):Byore Moore(比KMP算法快很多)
class Solution {
public:
int strStr(string haystack, string needle) {
int n=haystack.length(),m=needle.length(),skip;
vector<int>Right(256,-1);
for(int i=0;i<m;i++)
Right[needle[i]]=i;
for(int i=0;i<=n-m;i+=skip){
skip=0;
for(int j=m-1;j>=0;--j){
if(needle[j]!=haystack[i+j]){
skip=j-Right[haystack[i+j]];
if(skip<1)
skip=1;
break;
}
}
if(skip==0)
return i;
}
return -1;
}
};
Byore Moore算法模板
#include <iostream>
#include <vector>
#include <string>
using namespace std;
string pat,txt;
int R=256,M,N,skip;
std::vector<int> right(256,-1);
int BoyerMoore (){
M=pat.length();N=txt.length();
for(int i=0;i<M;i++)
right[pat[i]]=i;
for(int i=0;i<=N-M;i+=skip){
skip=0;
for(int j=M-1;j>=0;j--)
if(pat[j]!=txt[i+j]){
skip=j-right[txt[i+j]];
if(skip<1)
skip=1;
break;
}
if(skip==0)
return i;
}
return -1;
}