理论部分参见:http://tpglzu2015.iteye.com/blog/2206134
测试结果,与以上理论基本一致。
测试相关代码
hibernate.cfg.xml
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!-- Database connection settings --> <property name="connection.driver_class">org.postgresql.Driver</property> <property name="connection.url">jdbc:postgresql://127.0.0.1:5432/XXXXXX</property> <property name="connection.username">XXXXXX</property> <property name="connection.password">XXXXXX</property> <!-- JDBC connection pool (use the built-in) --> <property name="connection.pool_size">30</property> <!-- SQL dialect --> <property name="dialect">org.hibernate.dialect.PostgresPlusDialect</property> <!-- Echo all executed SQL to stdout --> <property name="show_sql">true</property> <property name="format_sql">true</property> <property name="hibernate.jdbc.batch_size">100</property> <property name="hibernate.jdbc.fetch_size">50</property> <property name="hibernate.cache.use_second_level_cache">true</property> <property name="hibernate.cache.use_query_cache">true</property> <property name="net.sf.ehcache.configurationResourceName">ehcache.xml</property> <!-- Enable Hibernate's automatic session context management --> <!--<property name="current_session_context_class">thread</property>--> <!-- Drop and re-create the database schema on startup --> <!-- <property name="hbm2ddl.auto">create</property> --> <!-- Disable the second-level cache --> <!-- <property name="cache.provider_class">org.hibernate.cache.NoCacheProvider</property> --> <property name="hibernate.cache.region.factory_class">org.hibernate.cache.ehcache.EhCacheRegionFactory</property> <mapping resource="hibernateDemo/hbms/testtable.hbm.xml"/> </session-factory> </hibernate-configuration>
testtable.hbm.xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd"> <hibernate-mapping package="hibernateDemo.beans"> <class name="TestTable"> <cache usage="read-write"/> <id name="id"></id> <property name="name"></property> </class> </hibernate-mapping>
注意事项,如果cache节点未配置的话,是不会对该对象进行二级缓存的。
所以,需要配置缓存策略的对象需添加cache节点,不需要配置缓存策略的对象需删除cache节点
扫描二维码关注公众号,回复:
484810 查看本文章
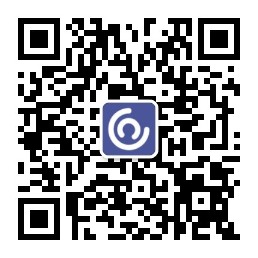
ehcache.xml
<?xml version="1.0" encoding="UTF-8"?> <ehcache> <diskStore path="java.io.tmpdir" /> <cache name="hibernateDemo.beans.TestTable" maxElementsInMemory="10000" eternal="false" timeToIdleSeconds="120" timeToLiveSeconds="120" overflowToDisk="true" maxElementsOnDisk="10000000" diskPersistent="false" diskExpiryThreadIntervalSeconds="120" memoryStoreEvictionPolicy="LRU" /> <defaultCache maxElementsInMemory="0" eternal="false" timeToIdleSeconds="0" timeToLiveSeconds="0" overflowToDisk="false" /> </ehcache>
TestMain.java
package hibernateDemo; import hibernateDemo.beans.TestTable; import java.util.List; import org.hibernate.Query; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.cfg.Configuration; public class TestMain { private Configuration cfg; private SessionFactory sf; Session session; public void setup() { cfg = new Configuration(); sf = cfg.configure().buildSessionFactory(); session = sf.openSession(); } public void close() { session.close(); sf.close(); } public void insert(Long id, String name) { TestTable s = new TestTable(); s.setId(id); s.setName(name); session.beginTransaction(); session.save(s); session.getTransaction().commit(); } public void delete(Long id) { TestTable s = new TestTable(); s.setId(id); session.beginTransaction(); session.delete(s); session.getTransaction().commit(); } public void update(Long id, String name) { TestTable s = new TestTable(); s.setId(id); s.setName(name); session.beginTransaction(); session.update(s); session.getTransaction().commit(); } public void search(Long id) { session.beginTransaction(); String queryStr = "from TestTable "; if (id != null) { queryStr += " where id = " + id; } Query query = session.createQuery(queryStr); List<TestTable> testTables = query.list(); for (TestTable testTable : testTables) { System.out.println(testTable.getId() + "\t" + testTable.getName()); } session.getTransaction().commit(); } public void getTest_SessionCache() { System.err.println("getTest_SessionCache ... do"); setup(); // get method test TestTable testTable = (TestTable) session.get(TestTable.class, 2L); System.out.println("id = " + testTable.getId()); System.out.println("name = " + testTable.getName()); System.out.println("--------------------------"); testTable = (TestTable) session.get(TestTable.class, 2L); System.out.println("id = " + testTable.getId()); System.out.println("name = " + testTable.getName()); close(); System.err.println("getTest_SessionCache ... end"); } public void getTest_FactoryCache() throws InterruptedException { setup(); System.err.println("getTest_FactoryCache ... do"); // get method test TestTable testTable = (TestTable) session.get(TestTable.class, 2L); System.out.println("id = " + testTable.getId()); System.out.println("name = " + testTable.getName()); session.close(); System.out.println("--------------------------"); Thread.sleep(10000); session = sf.openSession(); testTable = (TestTable) session.get(TestTable.class, 2L); System.out.println("id = " + testTable.getId()); System.out.println("name = " + testTable.getName()); System.err.println("getTest_FactoryCache ... end"); close(); } public void loadTest_SessionCache() { setup(); System.err.println("loadTest_SessionCache ... do"); // get method test TestTable testTable = (TestTable) session.load(TestTable.class, 2L); System.out.println("id = " + testTable.getId()); System.out.println("name = " + testTable.getName()); System.out.println("--------------------------"); testTable = (TestTable) session.load(TestTable.class, 2L); System.out.println("id = " + testTable.getId()); System.out.println("name = " + testTable.getName()); System.err.println("loadTest_SessionCache ... end"); close(); } public static void main(String[] args) throws InterruptedException { TestMain testMain = new TestMain(); // testMain.insert(3L, "s3"); // testMain.search(null); // testMain.update(id, name); // testMain.delete(1L); // testMain.getTest_SessionCache(); // testMain.loadTest_SessionCache(); testMain.getTest_FactoryCache(); } }
Log4J.xml
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE log4j:configuration SYSTEM "log4j.dtd"> <log4j:configuration xmlns:log4j='http://jakarta.apache.org/log4j/'> <appender name="rootAppender" class="org.apache.log4j.varia.NullAppender"/> <appender name="TEST" class="org.apache.log4j.RollingFileAppender"> <param name="MaxFileSize" value="5MB" /> <param name="MaxBackupIndex" value="65535" /> <param name="file" value="D:/log/test.log" /> <param name="append" value="true" /> <layout class="org.apache.log4j.PatternLayout"> <param name="ConversionPattern" value="%d{yyyy/MM/dd HH:mm:ss.SSS} %x LOCATION=%C{1}.%M(Line:%L) %m%n" /> </layout> <filter class="org.apache.log4j.varia.LevelRangeFilter"> <param name="LevelMin" value="DEBUG" /> </filter> </appender> <appender name="CONSOLE" class="org.apache.log4j.ConsoleAppender"> <layout class="org.apache.log4j.PatternLayout"> <param name="ConversionPattern" value="%d{yyyy/MM/dd HH:mm:ss.SSS} %p %c %x %m%n" /> </layout> <filter class="org.apache.log4j.varia.LevelRangeFilter"> <param name="LevelMin" value="DEBUG" /> </filter> </appender> <logger name="net.sf.ehcache" class="org.apache.log4j.Logger" additivity="false"> <level value="DEBUG" /> <appender-ref ref="TEST" /> <appender-ref ref="CONSOLE"/> </logger> <!-- <logger name="org.hibernate.SQL" class="org.apache.log4j.Logger" additivity="false"> <level value="DEBUG" /> <appender-ref ref="TEST" /> <appender-ref ref="CONSOLE"/> </logger> --> <logger name="org.hibernate.cache" class="org.apache.log4j.Logger" additivity="false"> <level value="DEBUG" /> <appender-ref ref="TEST" /> <appender-ref ref="CONSOLE"/> </logger> <root> <level value="WARN"/> <appender-ref ref="APP"/> <appender-ref ref="CONSOLE"/> </root> </log4j:configuration>