列表是一组有序的数据。每个列表中的数据项称为元素。在 JavaScript 中,列表中的元素可以是任意数据类型。列表中可以保存多少元素并没有事先限定并可以不断壮大,实际使用时元素的数量受到程序内存的限制。
(1)列表的抽象数据类型定义:
listSize(属性) 列表的元素个数
pos(属性) 列表的当前位置
length(属性) 返回列表中元素的个数
clear(方法) 清空列表中的所有元素
toString(方法) 返回列表的字符串形式
getElement(方法) 返回当前位置的元素
insert(方法) 在现有元素后插入新元素
append(方法) 在列表的末尾添加新元素
remove(方法) 从列表中删除元素
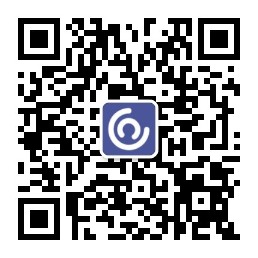
front(方法) 将列表的当前位置设移动到第一个元素
end(方法) 将列表的当前位置移动到最后一个元素
prev(方法) 将当前位置后移一位
next(方法) 将当前位置前移一位
currPos(方法) 返回列表的当前位置
moveTo(方法) 将当前位置移动到指定位置
(2)实现列表类
function List() { this.listSize = 0; this.pos = 0; this.dataStore = []; // 初始化一个空数组来保存列表元素 this.clear = clear; this.find = find; this.toString = toString; this.insert = insert; this.append = append; this.remove = remove; this.front = front; this.end = end; this.prev = prev; this.next = next; this.length = length; this.currPos = currPos; this.moveTo = moveTo; this.getElement = getElement; this.length = length; this.contains = contains; }
append()方法给列表的下一个位置增加一个新的元素,这 个位置刚好等于变量 listSize 的值
function append(element) { this.dataStore[this.listSize++] = element; }
find()方法在列表中查找某一元素,如果找到,就返回该 元素在列表中的位置,否则返回 -1
function find(element) { for (var i = 0; i < this.dataStore.length; ++i) { if (this.dataStore[i] == element) { return i; } } return -1; }
remove()方法从列表中删除元素。首先,需要在列表中找到该元素,然后删除它
function remove(element) { var foundAt = this.find(element); if (foundAt > -1) { this.dataStore.splice(foundAt,1); --this.listSize; return true; } return false; }
length()方法返回列表中有多少个元素
function length() { return this.listSize; }
toString():显示列表中的元素
function toString() { return this.dataStore; }
insert():向列表中插入一个元素,这里的插入是指插入到指定的列表中的元素之后
function insert(element, after) { //element:要插入的元素,after:列表中指定的元素(element插入到该元素之后) var insertPos = this.find(after); //find() 方法寻找传入的 after 参数在列表中的位置 if (insertPos > -1) { this.dataStore.splice(insertPos+1, 0, element); ++this.listSize; return true; } return false; }
clear():清空列表中所有的元素。clear() 方法使用 delete 操作符删除数组 dataStore,接着在下一行创建一个空数组。最后一行将 listSize 和 pos 的值设为 1,表明这是一个新的空列表。
function clear() { delete this.dataStore; this.dataStore = []; this.listSize = this.pos = 0; }
contains():判断给定值是否在列表中
function contains(element) { for (var i = 0; i < this.dataStore.length; ++i) { if (this.dataStore[i] == element) { return true; } } return false; }
遍历列表
function front() { this.pos = 0; } function end() { this.pos = this.listSize-1; } function prev() { if (this.pos > 0) { --this.pos; } } function next() { if (this.pos < this.listSize-1) { ++this.pos; } } function currPos() { return this.pos; } function moveTo(position) { this.pos = position; } function getElement() { return this.dataStore[this.pos]; }
使用迭代器遍历列表。使用迭代器,可以不必关心数据的内部存储方式,以实现对列表的遍历
for(names.front(); names.currPos() < names.length();names.next()) { print(names.getElement()); }
列表数据结构可解决问题举例:影碟租赁程序:使用列表管理影碟,将数据读入列表,当有人借影碟时将元素移出。