邻接表表示图
#include <iostream>
#include <malloc.h>
#include <queue>
using namespace std;
#define VertexType char
#define MaxVertexNum 20
queue<int>Q;
enum GraphType{DG, UDG};
typedef struct ArcNode{
int adjvex;
struct ArcNode *nextarc;
}ArcNode;
typedef struct VNode{
VertexType data;
ArcNode *firstarc;
}VNode, AdjList[MaxVertexNum];
typedef struct{
AdjList vertices;
int vexnum,arcnum;
GraphType kind;
}ALGraph;
void CreateDGGraph(ALGraph &G)
{
int m, n;
ArcNode *vm, *vn;
cout << "请输入顶点数和边数:";
cin >> G.vexnum >> G.arcnum;
cout << "请输入顶点的信息:" << endl;
for(int i=0; i<G.vexnum; i++)
{
cin >> G.vertices[i].data;
G.vertices[i].firstarc=NULL;
}
for(int i=0; i<G.arcnum; i++)
{
cout << "请输入<Vi,Vj>的下标:";
cin >> m >> n;
vm=(ArcNode*)malloc(sizeof(ArcNode));
vm->adjvex=n;
vm->nextarc=NULL;
if(G.vertices[m].firstarc==NULL)
{
vn=G.vertices[m].firstarc=vm;
}
else
{
vn=vn->nextarc=vm;
}
}
}
void CreateUDGGraph(ALGraph &G)
{
int m, n;
ArcNode *pe;
cout << "请输入顶点数和边数:";
cin >> G.vexnum >> G.arcnum;
cout << "请输入顶点的信息:" << endl;
for(int i=0; i<G.vexnum; i++)
{
cin >> G.vertices[i].data;
G.vertices[i].firstarc=NULL;
}
for(int i=0; i<G.arcnum; i++)
{
cout << "请输入<Vi,Vj>的下标:";
cin >> m >> n;
pe=(ArcNode*)malloc(sizeof(ArcNode));
pe->adjvex=n;
pe->nextarc=G.vertices[m].firstarc;
G.vertices[m].firstarc=pe;
pe=(ArcNode*)malloc(sizeof(ArcNode));
pe->adjvex=m;
pe->nextarc=G.vertices[n].firstarc;
G.vertices[n].firstarc=pe;
}
}
void PrintALGraph(ALGraph &G)
{
ArcNode *p;
for (int i=0;i<G.vexnum;i++)
{
cout << "顶点" << i << "(" << G.vertices[i].data << ") "": ";
for (p=G.vertices[i].firstarc; p!=NULL;p=p->nextarc)
cout << p->adjvex << "(" << G.vertices[p->adjvex].data << ") ";
if (p==NULL)
cout << endl;
}
}
int FirstNeighbor(ALGraph G, int v)
{
ArcNode *next=G.vertices[v].firstarc;
if(next)
{
return next->adjvex;
}
return -1;
}
int NextNeighbor(ALGraph G, int v, int w)
{
ArcNode *next=G.vertices[v].firstarc;
while(next)
{
if(next->adjvex==w)break;
next=next->nextarc;
}
if(next)
{
ArcNode *nextNode=next->nextarc;
if(nextNode)
{
return nextNode->adjvex;
}
}
return -1;
}
void visit(ALGraph G, int v)
{
cout << G.vertices[v].data << " ";
}
bool visited[MaxVertexNum];
void BFS(ALGraph G, int v)
{
visit(G, v);
visited[v]=true;
Q.push(v);
while(!Q.empty())
{
v=Q.front();
Q.pop();
for(int w=FirstNeighbor(G, v); w>=0; w=NextNeighbor(G, v, w))
{
if(!visited[w])
{
visit(G, w);
visited[w]=true;
Q.push(w);
}
}
}
}
void BFSTraverse(ALGraph G)
{
for(int i=0; i<G.vexnum; i++)
{
visited[i]=false;
}
for(int i=0; i<G.vexnum; i++)
{
if(!visited[i])
{
BFS(G, i);
}
}
}
void DFS(ALGraph G, int v)
{
visit(G, v);
visited[v]=true;
for(int w=FirstNeighbor(G, v); w>=0; w=NextNeighbor(G, v, w))
{
if(!visited[w])
{
DFS(G, w);
}
}
}
void DFSTraverse(ALGraph G)
{
for(int i=0; i<G.vexnum; i++)
{
visited[i]=false;
}
for(int i=0; i<G.vexnum; i++)
{
if(!visited[i])
{
DFS(G, i);
}
}
}
int main()
{
ALGraph G;
int tag;
cout << "请输入1(有向图),2(无向图):";
cin >> tag;
if(tag==1)
{
G.kind=DG;
CreateDGGraph(G);
}
else
{
G.kind=UDG;
CreateUDGGraph(G);
}
cout << "===========================" << endl;
PrintALGraph(G);
cout <<endl;
cout << "广度遍历:";
BFSTraverse(G);
cout << endl;
cout << "深度遍历:";
DFSTraverse(G);
return 0;
}
有向图测试
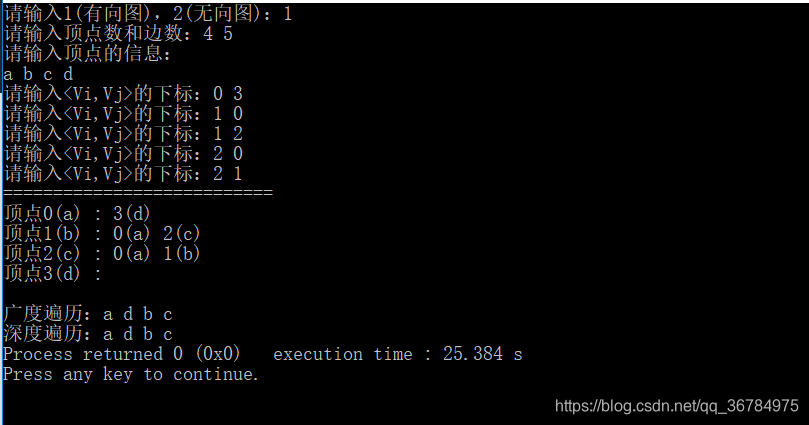
无向图测试
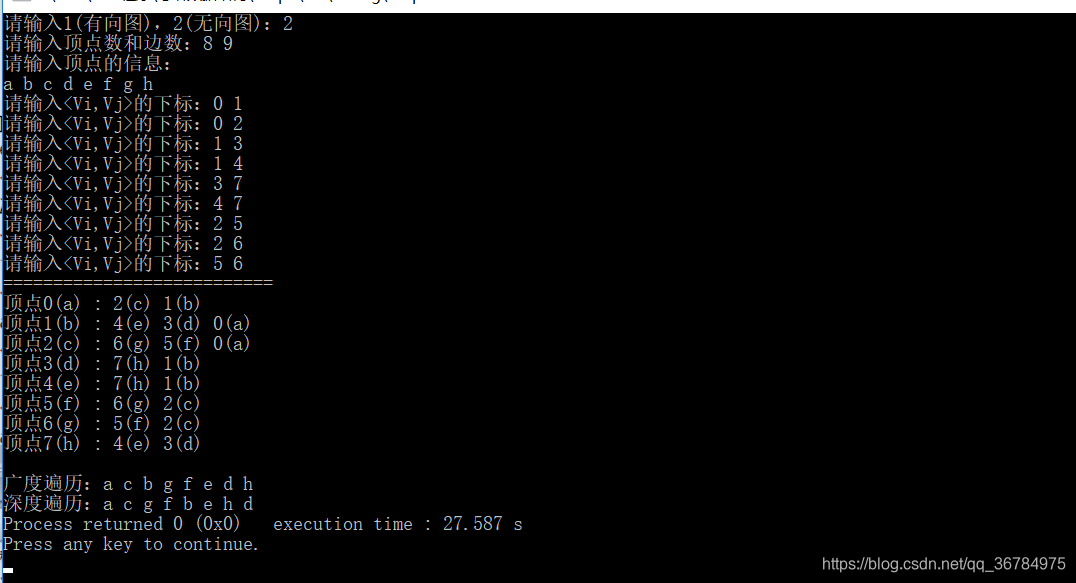