版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/ChiBaoNeLiuLiuNi/article/details/72769778
Given an array of integers, find out whether there are two distinct indices i and j in the array such that the absolute difference between nums[i] and nums[j] is at most t and the absolute difference between i and j is at most k.
--------------------------------------
两种解法, 1, bucket。 2, Treeset
相通之处在于都是维护一个长度为K的sliding window来确保 absolute difference between i and j is at most k.
转换成long避免溢出, 比如 Integer.MIN_VALUE (-2147483648, -2147483647)k = 3, t = 3 这种input
Bucket:O(n)
扫描二维码关注公众号,回复:
4663904 查看本文章
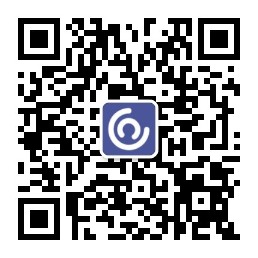
public class Solution {
public boolean containsNearbyAlmostDuplicate(int[] nums, int k, int t) {
if(k < 1 || t < 0) return false;
long valueDiff = (long)t;
long indexDiff = (long)k;
Map<Long, Long> map = new HashMap<>();
for(int i = 0; i < nums.length; i++){
long value = (long)nums[i] - Integer.MIN_VALUE;
long bucket = value / (valueDiff + 1);
if(map.containsKey(bucket) || map.containsKey(bucket - 1) && value - map.get(bucket - 1) <= valueDiff
|| map.containsKey(bucket + 1) && map.get(bucket + 1) - value <= valueDiff)
return true;
map.put(bucket, value);
if(i >= k){
long idx = ((long)nums[i - k] - Integer.MIN_VALUE) / (valueDiff + 1);
map.remove(idx);
}
}
return false;
}
}
TreeSet: O(nlogk)
public class Solution {
public boolean containsNearbyAlmostDuplicate(int[] nums, int k, int t) {
TreeSet<Long> set = new TreeSet<>();
for(int i = 0; i < nums.length; i++){
Long ceil = set.ceiling((long)nums[i] - (long)t);
Long floor = set.floor((long)nums[i] + (long)t);
if(ceil != null && ceil <= nums[i] || floor != null && floor >= nums[i])
return true;
set.add((long)nums[i]);
if(i >= k)
set.remove((long)nums[i - k]);
}
return false;
}
}