1复习
# 迭代器和生成器 # 迭代器 # 可迭代协议 —— 含有iter方法的都是可迭代的 # 迭代器协议 —— 含有next和iter的都是迭代器 # 特点 # 节省内存空间 # 方便逐个取值,一个迭代器只能取一次。 # 生成器 —— 迭代器 # 生成器函数 # 含有yield关键字的函数都是生成器函数 # 生成器函数的特点 #调用之后函数内的代码不执行,返回生成器 #每从生成器中取一个值就会执行一段代码,遇见yield就停止。 #如何从生成器中取值: # for :如果没有break会一直取直到取完 # next :每次只取一个 # send :不能用在第一个,取下一个值的时候给上个位置传一个新的值 # 数据类型强制转换 :会一次性把所有数据都读到内存里 # 生成器表达式 # (条件成立想放在生成器中的值 for i in 可迭代的 if 条件)
2作业
# 3.处理文件,用户指定要查找的文件和内容,将文件中包含要查找内容的每一行都输出到屏幕 def check_file(filename,aim): with open(filename,encoding='utf-8') as f: #句柄 : handler,文件操作符,文件句柄 for i in f: if aim in i: yield i g = check_file('1.复习.py','生成器') for i in g: print(i.strip()) <<< # 迭代器和生成器 # 生成器 —— 迭代器 # 生成器函数 # 含有yield关键字的函数都是生成器函数 # 生成器函数的特点 #调用之后函数内的代码不执行,返回生成器 #每从生成器中取一个值就会执行一段代码,遇见yield就停止。 #如何从生成器中取值: # 生成器表达式 # (条件成立想放在生成器中的值 for i in 可迭代的 if 条件)
def check_file(filename): with open(filename,encoding='utf-8') as f: #句柄 : handler,文件操作符,文件句柄 for i in f: if '迭代器' in i: yield '***'+i for i in check_file('1.复习.py'): print(i.strip()) <<< ***# 迭代器和生成器 ***# 迭代器 *** # 迭代器协议 —— 含有next和iter的都是迭代器 *** # 方便逐个取值,一个迭代器只能取一次。 ***# 生成器 —— 迭代器
3面试题
def demo(): for i in range(4): yield i g=demo() g1=(i for i in g) g2=(i for i in g1) print(list(g)) print(list(g1)) print(list(g2)) <<< [0, 1, 2, 3] [] []
def add(n,i): return n+i def test(): for i in range(4): yield i g=test() for n in [1,10,5]: g=(add(n,i) for i in g) # n = 1 # g=(add(n,i) for i in test()) #g=(1,2,3,4) # n = 10 # g=(add(n,i) for i in (add(n,i) for i in test())) # g=(11, 12,13 ,14) # n = 5 # g=(15,16,17,18) print(list(g)) <<< [15, 16, 17, 18]
4内置函数
# print() # input() # len() # type() # open() # tuple() # list() # int() # bool() # set() # dir() # id() # str() # print(locals()) #返回本地作用域中的所有名字 # print(globals()) #返回全局作用域中的所有名字 # global 变量 # nonlocal 变量 #迭代器.__next__() # next(迭代器) # def next(迭代器): # 迭代器.__next__() # 迭代器 = iter(可迭代的) # 迭代器 = 可迭代的.__iter__()
range
range(10) range(1,11) print('__next__' in dir(range(1,11,2))) <<< False
dir
# dir 查看一个变量拥有的方法 print(dir([])) print(dir(1)) <<< ['__add__', '__class__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort'] ['__abs__', '__add__', '__and__', '__bool__', '__ceil__', '__class__', '__delattr__', '__dir__', '__divmod__', '__doc__', '__eq__', '__float__', '__floor__', '__floordiv__', '__format__', '__ge__', '__getattribute__', '__getnewargs__', '__gt__', '__hash__', '__index__', '__init__', '__init_subclass__', '__int__', '__invert__', '__le__', '__lshift__', '__lt__', '__mod__', '__mul__', '__ne__', '__neg__', '__new__', '__or__', '__pos__', '__pow__', '__radd__', '__rand__', '__rdivmod__', '__reduce__', '__reduce_ex__', '__repr__', '__rfloordiv__', '__rlshift__', '__rmod__', '__rmul__', '__ror__', '__round__', '__rpow__', '__rrshift__', '__rshift__', '__rsub__', '__rtruediv__', '__rxor__', '__setattr__', '__sizeof__', '__str__', '__sub__', '__subclasshook__', '__truediv__', '__trunc__', '__xor__', 'bit_length', 'conjugate', 'denominator', 'from_bytes', 'imag', 'numerator', 'real', 'to_bytes']
help
help(str)
<<<
class str(object)
| str(object='') -> str
| str(bytes_or_buffer[, encoding[, errors]]) -> str
| Create a new string object from the given object. If encoding or | errors is specified, then the object must expose a data buffer | that will be decoded using the given encoding and error handler. | Otherwise, returns the result of object.__str__() (if defined) | or repr(object). | encoding defaults to sys.getdefaultencoding(). | errors defaults to 'strict'. | | Methods defined here: | | __add__(self, value, /) | Return self+value. | | __contains__(self, key, /) | Return key in self.| | __format__(...) | S.__format__(format_spec) -> str | | Return a formatted version of S as described by format_spec.
| __hash__(self, /) | Return hash(self). | | __iter__(self, /) | Implement iter(self).| | __len__(self, /) | Return len(self).
collable
#callable 可调用的 检测是不是一个函数 # 变量 print(callable(print)) a = 1 print(callable(a)) print(callable(globals)) def func():pass print(callable(func)) <<< True False True True
time
import time #( t = __import__('time')) print(time.time()) <<< 1545206579.6000051 #1970年到现在经历的秒数
writable
# 某个方法属于某个数据类型的变量,就用.调用 list.__len__() # 如果某个方法不依赖于任何数据类型,就直接调用 —— 内置函数 和 自定义函数 f = open('1.复习.py') print(f.writable()) print(f.readable()) <<< False True
#输入输出 # ret = input('提示 : ') # print(ret)
def print(self, *args, sep=' ', end='\n', file=None):
print('我们的祖国是花园',end='') #指定输出的结束符
print('我们的祖国是花园 ',end='') print(1,2,3,4,5,sep='|') #指定输出多个值之间的分隔符
f = open('file','w')
print('aaaa',file=f) f.close() <<< 我们的祖国是花园我们的祖国是花园 1|2|3|4|5
hash
#内存相关 #id #hash - 对于相同可hash数据的hash值在一次程序的执行过程中总是不变的ab # - 字典的寻址方式 print(hash(12345)) print(hash('hsgda不想你走,nklgkds')) print(hash('hsgda不想你走,nklgkds')) print(hash(('1','aaa'))) <<< 12345 -2573559241142890582 -2573559241142890582 4497054594156916011 print(hash([])) 会报错 不可哈西
文本进度条
#打印进度条 import time for i in range(0,101,2): time.sleep(0.1) char_num = i//2 per_str = '\r%s%% : %s\n' % (i, '*' * char_num) \ if i == 100 else '\r%s%% : %s' % (i,'*'*char_num) print(per_str,end='', flush=True) #progress Bar <<< 100% : **************************************************
exec和eval
exec('print(123)') eval('print(123)') print(eval('1+2+3+4')) # 有返回值 print(exec('1+2+3+4')) #没有返回值 # exec和eval都可以执行 字符串类型的代码 # eval有返回值 —— 有结果的简单计算 # exec没有返回值 —— 简单流程控制 # eval只能用在你明确知道你要执行的代码是什么 123 123 10 None
code = '''for i in range(1,5): print(i*'*') ''' exec(code) * ** *** ****
compile
code1 = 'for i in range(0,3): print (i)' #流程类 compile1 = compile(code1,'','exec') exec(compile1) code2 = '1 + 2 + 3 + 4' compile2 = compile(code2,'','eval') #计算类 print(eval(compile2)) code3 = 'name = input("please input your name:")' compile3 = compile(code3,'','single') #交互式 exec(compile3) #执行时显示交互命令,提示输入 print(name) # name #执行后name变量有值 <<< 0 1 2 10 please input your name:hhh hhh
complex
# 复数 —— complex #real+img j 实部和虚部都是浮点数 复数不能比较大小 # 5 + 12j === 复合的数 === 复数 aa=123-12j print aa.real # output 实数部分 123.0 print aa.imag # output虚数部分 -12.0
浮点数
# 浮点数(有限循环小数,无限循环小数) != 小数 :有限循环小数,无限循环小数,无限不循环小数 # 浮点数 #354.123 = 3.54123*10**2 = 35.4123 * 10 f = 1.781326913750135970 print(f)
<<<
1.781326913750136
扫描二维码关注公众号,回复:
4566989 查看本文章
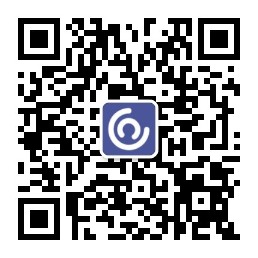
二 、八 、十六进制
print(bin(10)) print(oct(10)) print(hex(10)) <<< 0b1010 0o12 0xa
divmod
print(abs(5)) #绝对值 print(divmod(7,2)) # div除法 mod取余 print(divmod(9,5)) # 除余 <<< 5 (3, 1) (1, 4)
round和pow
print(round(3.14159,3)) print(pow(2,3)) #pow幂运算 == 2**3 print(pow(2,3,3)) #幂运算之后再取余 print(pow(3,2,1)) <<< 3.142 8 2 0
sum
ret = sum([1,2,3,4,5,6]) print(ret) ret = sum([1,2,3,4,5,6,10],) print(ret) <<< 21 31
min和max
print(min([1,2,3,4])) print(min(1,2,3,-4)) print(min(1,2,3,-4,key = abs)) print(max([1,2,3,4])) print(max(1,2,3,-4)) print(max(1,2,3,-4,key = abs)) <<< 1 -4 1 4 3 -4
ord chr
print("对应的ASCII 码为", ord('a')) print(" 对应的字符为", chr(65)) <<< 对应的ASCII 码为 97 对应的字符为 A
5试题讲解
#元祖不能比较大小 字母可以 #while:True 永生语句 # print(2**10) 1024
#若k为整形,下列while循环执行的次数为: k=1000 i=0 while k>1: i=i+1 print (i,k) #python2 中为9? 2**10=1024 k=k/2 <<< 1 1000 2 500.0 3 250.0 4 125.0 5 62.5 6 31.25 7 15.625 8 7.8125 9 3.90625 10 1.953125
def qqxing(k,l={}): # l.append(1) l[k]='v' print(l) qqxing(1) qqxing(2) qqxing(3) qqxing(4,{}) <<< {1: 'v'} {1: 'v', 2: 'v'} {1: 'v', 2: 'v', 3: 'v'} {4: 'v'}
删除重复元素
# 写一段python代码实现删除一个list里面重复元素 list=[1,1,2,2,3] new_list=[] for i in list: if i not in new_list: new_list.append(i) #比set好一点 set无序 print(new_list) <<< [1, 2, 3]