首先打开IDEA,File—>New—>Project创建项目
选择左侧导航栏里的Maven,勾上勾,选择webapp
按如下图进行填写
创建完成后进入项目,右下角弹出的提示点击右边的Enable Auto-Import,自动配置
连接数据库,我用的是Mysql数据库,准备好有数据的数据库表
扫描二维码关注公众号,回复:
4547076 查看本文章
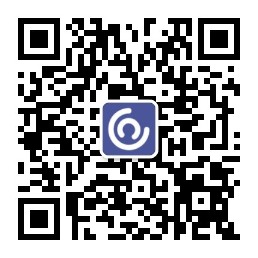
在pom.xml里导入所需jar包:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<packaging>war</packaging>
<name>Student-ssm</name>
<groupId>com.accp</groupId>
<artifactId>Student-ssm</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.8.8</version>
</dependency>
<!-- spring-webmvc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>4.3.12.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>4.3.12.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>4.3.12.RELEASE</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.4.5</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>1.3.1</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.44</version>
</dependency>
<!--数据源-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.2</version>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!-- https://mvnrepository.com/artifact/javax.servlet/javax.servlet-api -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.0.1</version>
<scope>provided</scope>
</dependency>
</dependencies>
</project>
总体结构:
web.xml代码:
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.4"
xmlns="http://java.sun.com/xml/ns/j2ee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd">
<!--乱码解决-->
<filter>
<filter-name>characterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>characterEncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<!--配置springmvc-->
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springmvc.xml</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
springmvc.xml代码:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-3.2.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.2.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-3.2.xsd"
default-autowire="byName">
<context:component-scan base-package="com.accp"/>
<!--数据库连接-->
<bean name="dataSource" class="com.alibaba.druid.pool.DruidDataSource">
<property name="url" value="jdbc:mysql://localhost:3306/student?useUnicode=true&characterEncoding=utf-8"/>
<property name="driverClassName" value="com.mysql.jdbc.Driver"/>
<property name="username" value="root"/>
<property name="password" value="994994"/>
</bean>
<!--映射xml-->
<bean name="sessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean" p:typeAliasesPackage="com.accp.entity">
<property name="dataSource" ref="dataSource"/>
<property name="mapperLocations" value="classpath:xml/*.xml"/>
</bean>
<!--相当于StudentinfoDao studentinfoDao = session.getMapper(StudentinfoDao.class) 获取-->
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="sqlSessionFactoryBeanName" value="sessionFactory"/>
<property name="basePackage" value="com.accp.dao"/>
</bean>
<!--声明式事务-->
<bean name="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager" p:dataSource-ref="dataSource"/>
<tx:advice id="txAdvice" transaction-manager="transactionManager">
<tx:attributes>
<tx:method name="add*" propagation="REQUIRED"/>
<tx:method name="update*"/>
<tx:method name="delete*"/>
<tx:method name="query*" propagation="NOT_SUPPORTED"/>
</tx:attributes>
</tx:advice>
<aop:config>
<aop:pointcut id="pointcut" expression="execution(* com.accp.service..*.*(..))"/>
<aop:advisor advice-ref="txAdvice" pointcut-ref="pointcut"/>
</aop:config>
<!--视图解析-->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/jsp/"/>
<property name="suffix" value=".jsp"/>
</bean>
<mvc:annotation-driven/>
</beans>
entity学生实体类代码:
package com.accp.entity;
public class Studentinfo {
private long sid;
private String sname;
private String sgender;
private long sage;
private String saddress;
private String semail;
public long getSid() {
return sid;
}
public void setSid(long sid) {
this.sid = sid;
}
public String getSname() {
return sname;
}
public void setSname(String sname) {
this.sname = sname;
}
public String getSgender() {
return sgender;
}
public void setSgender(String sgender) {
this.sgender = sgender;
}
public long getSage() {
return sage;
}
public void setSage(long sage) {
this.sage = sage;
}
public String getSaddress() {
return saddress;
}
public void setSaddress(String saddress) {
this.saddress = saddress;
}
public String getSemail() {
return semail;
}
public void setSemail(String semail) {
this.semail = semail;
}
}
dao层StudentinfoDao代码:
package com.accp.dao;
import com.accp.entity.Studentinfo;
import java.util.List;
public interface StudentinfoDao {
List<Studentinfo> queryStudent();
int addStudentinfo(Studentinfo studentinfo);
int deleteStudentinfo(Studentinfo studentinfo);
int updateStudentinfo(Studentinfo studentinfo);
Studentinfo getByStudentId(Studentinfo studentinfo);
}
resources下xml里Studentinfo.xml代码:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.accp.dao.StudentinfoDao">
<select id="queryStudent" resultType="Studentinfo">
select * from studentinfo;
</select>
<select id="getByStudentId" resultType="Studentinfo">
select * from studentinfo where sid = #{sid}
</select>
<insert id="addStudentinfo">
insert into studentinfo value (default ,#{sname},#{sgender},#{sage},#{saddress},#{semail})
</insert>
<delete id="deleteStudentinfo">
delete from studentinfo where sid = #{sid}
</delete>
<update id="updateStudentinfo">
update studentinfo
<trim prefix="set" suffixOverrides="," suffix="where sid=#{sid}">
<if test="sname!=null">sname = #{sname},</if>
<if test="sgender!=null">sgender = #{sgender},</if>
<if test="sage!=null">sage = #{sage},</if>
<if test="saddress!=null">saddress = #{saddress},</if>
<if test="semail!=null">semail = #{semail},</if>
</trim>
</update>
</mapper>
service层StudentinfoService代码:
package com.accp.service;
import com.accp.entity.Studentinfo;
import java.util.List;
public interface StudentinfoService {
List<Studentinfo> queryStudent();
int addStudentinfo(Studentinfo studentinfo);
int deleteStudentinfo(Studentinfo studentinfo);
int updateStudentinfo(Studentinfo studentinfo);
Studentinfo getByStudentId(Studentinfo studentinfo);
}
service层Impl实现类StudentinfoServiceImpl代码:
package com.accp.service.impl;
import com.accp.dao.StudentinfoDao;
import com.accp.entity.Studentinfo;
import com.accp.service.StudentinfoService;
import org.springframework.stereotype.Service;
import javax.annotation.Resource;
import java.util.List;
@Service
public class StudentinfoServiceImpl implements StudentinfoService {
@Resource
private StudentinfoDao studentinfoDao;
public List<Studentinfo> queryStudent() {
return studentinfoDao.queryStudent();
}
public int addStudentinfo(Studentinfo studentinfo) {
return studentinfoDao.addStudentinfo(studentinfo);
}
public int deleteStudentinfo(Studentinfo studentinfo) {
return studentinfoDao.deleteStudentinfo(studentinfo);
}
public int updateStudentinfo(Studentinfo studentinfo) {
return studentinfoDao.updateStudentinfo(studentinfo);
}
public Studentinfo getByStudentId(Studentinfo studentinfo) {
return studentinfoDao.getByStudentId(studentinfo);
}
}
controller控制层StudentinfoController代码:
package com.accp.controller;
import com.accp.entity.Studentinfo;
import com.accp.service.StudentinfoService;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import javax.annotation.Resource;
@Controller
public class StudentinfoController {
@Resource
private StudentinfoService studentinfoService;
@RequestMapping("/showList")
public String showList(Model model){
model.addAttribute("students",studentinfoService.queryStudent());
return "index";
}
@RequestMapping("/JumpAdd")
public String jumpAdd(){
return "add";
}
@RequestMapping("/AddList")
public String addList(Studentinfo studentinfo){
studentinfoService.addStudentinfo(studentinfo);
return "redirect:showList";
}
@RequestMapping("/DeleteS")
public String deleteS(Studentinfo studentinfo){
studentinfoService.deleteStudentinfo(studentinfo);
return "redirect:showList";
}
@RequestMapping("/JumpUpdate")
public String jumpUpdate(Studentinfo studentinfo,Model model){
model.addAttribute("stu",studentinfoService.getByStudentId(studentinfo));
return "update";
}
@RequestMapping("/UpdateS")
public String updateS(Studentinfo studentinfo){
studentinfoService.updateStudentinfo(studentinfo);
return "redirect:showList";
}
}
jsp页面代码:
显示页面(包含删除操作):
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<a href="JumpAdd">增加</a>
<table width="100%" border="1">
<tr>
<td>编号</td>
<td>姓名</td>
<td>性别</td>
<td>年龄</td>
<td>地址</td>
<td>email</td>
<td colspan="2">操作</td>
</tr>
<c:forEach items="${students}" var="stu">
<tr>
<td>${stu.sid}</td>
<td>${stu.sname}</td>
<td>${stu.sgender}</td>
<td>${stu.sage}</td>
<td>${stu.saddress}</td>
<td>${stu.semail}</td>
<td><a href="DeleteS?sid=${stu.sid}">删除</a></td>
<td><a href="JumpUpdate?sid=${stu.sid}">修改</a></td>
</tr>
</c:forEach>
</table>
</body>
</html>
添加页面:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>添加</title>
</head>
<body>
<form action="AddList" method="post">
姓名:<input type="text" name="sname"></br>
性别:<input type="text" name="sgender"></br>
年龄:<input type="text" name="sage"></br>
地址:<input type="text" name="saddress"></br>
邮箱:<input type="text" name="semail"></br>
<input type="submit" value="添加">
</form>
</body>
</html>
修改页面:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>修改</title>
</head>
<body>
<form action="UpdateS?sid=${stu.sid}" method="post">
姓名:<input type="text" name="sname" value="${stu.sname}"></br>
性别:<input type="text" name="sgender" value="${stu.sgender}"></br>
年龄:<input type="text" name="sage" value="${stu.sage}"></br>
地址:<input type="text" name="saddress" value="${stu.saddress}"></br>
邮箱:<input type="text" name="semail" value="${stu.semail}"></br>
<input type="submit" value="修改">
</form>
</body>
</html>
显示效果: