Vue原型对象的包装
在Vue官网直接通过 script 标签导入的 Vue包是 umd模块的形式。在使用前都通过 new Vue({})。记录一下 Vue构造函数的包装。
在 src/core/instance/index.js 这个文件是 Vue构造函数的出生地。
import { initMixin } from './init'
import { stateMixin } from './state'
import { renderMixin } from './render'
import { eventsMixin } from './events'
import { lifecycleMixin } from './lifecycle'
import { warn } from '../util/index'
function Vue (options) {
// 使用安全模式来提醒要使用new操作符来调用Vue
if (process.env.NODE_ENV !== 'production' &&
!(this instanceof Vue)
) {
warn('Vue is a constructor and should be called with the `new` keyword')
}
this._init(options)
}
/**
* 在执行npm run dev构建运行时执行, 包装Vue.prototype。为其添加一些属性和方法
*/
initMixin(Vue)
stateMixin(Vue)
eventsMixin(Vue)
lifecycleMixin(Vue)
renderMixin(Vue)
export default Vue
首先导入了五个方法
- initMixin
- stateMixin
- renderMixin
- eventsMixin
- lifecycleMixin
如果不是在生产环境下,且不通过 new 来调用Vue 会得到警告。
接下来执行 initMixin方法, 到 initMixin来源文件看。
export function initMixin (Vue: Class<Component>) {
Vue.prototype._init = function (){}
}
首先会在 Vue这个构造函数的原型对象上定义一个 _init方法。这个方法是在通过 new Vue({})的时候执行。在 Vue 构造函数中可以看到 this._init(options)。
接着将 Vue构造函数作为参数传递给下一个 stateMixin方法, 到stateMixin来源文件看。
export function stateMixin (Vue: Class<Component>) {
// flow somehow has problems with directly declared definition object
// when using Object.defineProperty, so we have to procedurally build up
// the object here.
const dataDef = {}
dataDef.get = function () { return this._data }
const propsDef = {}
propsDef.get = function () { return this._props }
// 设置两个只读的属性 $data $props
if (process.env.NODE_ENV !== 'production') {
dataDef.set = function () {
warn(
'Avoid replacing instance root $data. ' +
'Use nested data properties instead.',
this
)
}
propsDef.set = function () {
warn(`$props is readonly.`, this)
}
}
Object.defineProperty(Vue.prototype, '$data', dataDef)
Object.defineProperty(Vue.prototype, '$props', propsDef)
Vue.prototype.$set = set
Vue.prototype.$delete = del
Vue.prototype.$watch = function (){}
}
其中
Object.defineProperty(Vue.prototype, '$data', dataDef)
Object.defineProperty(Vue.prototype, '$props', propsDef)
这是在 Vue的原型对象上定义了两个属性 $data
和 $props
。其中分别代理了 _data
和 _props
。看 dataDef 这个对象上定义了一个 get 方法, 最终返回当前实例对象的 _data。props 也是这样子的。
if (process.env.NODE_ENV !== 'production') {
dataDef.set = function () {
warn(
'Avoid replacing instance root $data. ' +
'Use nested data properties instead.',
this
)
}
propsDef.set = function () {
warn(`$props is readonly.`, this)
}
}
当你在非生产环境时, 如果修改 $data 和 $props会得到警告信息。
最后在 Vue.prototype上还定义了 $set、$delete以及 $watch。。
接下来是 eventMixin方法, 进入这个方法的来源文件
export function eventsMixin (Vue: Class<Component>) {
Vue.prototype.$on = function(){};
Vue.prototype.$once = function(){};
Vue.prototype.$off = function(){};
}
又再 Vue.prototype 上定义了三个方法, 分别是 $on
、$once
和$off
。
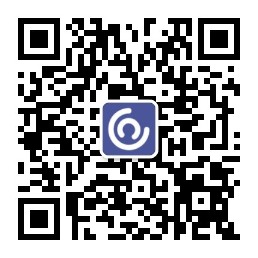
接下来执行 lifecycleMixin 方法, 看lifecycleMixin方法的来源文件:
export function lifecycleMixin (Vue: Class<Component>) {
Vue.prototype._update = function() {}
Vue.prototype.$forceUpdate = function() {}
Vue.prototype.$destroy = function(){}
在 lifecycleMixin 方法中又向 Vue的原型对象 prototype上添加了三个方法。分别是 _update
、$$forceUpdate
和$$destroy
。
$forceUpdate: 迫使 Vue 实例重新渲染。注意它仅仅影响实例本身和插入插槽内容的子组件,而不是所有子组件。
最后一个执行 renderMixin方法。在renderMixin来源文件可以到。
首先执行了一个 installRenderHelpers(Vue.prototype),这个方法的主要作用也是向 Vue.prototype上添加方法, 看它源文件是:
// 这个函数主要在Vue.prototype上面添加一些方法
export function installRenderHelpers (target: any) {
target._o = markOnce
target._n = toNumber
target._s = toString
target._l = renderList
target._t = renderSlot
target._q = looseEqual
target._i = looseIndexOf
target._m = renderStatic
target._f = resolveFilter
target._k = checkKeyCodes
target._b = bindObjectProps
target._v = createTextVNode
target._e = createEmptyVNode
target._u = resolveScopedSlots
target._g = bindObjectListeners
}
紧接着又向 Vue.prototype对象上添加了 $nextTick和_render方法。
export function renderMixin (Vue: Class<Component>) {
// install runtime convenience helpers
installRenderHelpers(Vue.prototype)
Vue.prototype.$nextTick = function (fn: Function) {
return nextTick(fn, this)
}
Vue.prototype._render = function (): VNode {}
执行完 renderMixin这个方法, Vue构造函数的出生文件也运行完了。也就是指在 npm run dev命令构建时运行。这里的每一个方法 *Mixin的作用就是包装 Vue.prototype, 对其挂载一些属性和方法。最后 export default Vue
将其导出这个构造函数。此时 Vue.prototype上添加的属性和方法有这些。
然后在哪里用到了呢。在 src/core/index.js中导入了Vue的出生文件。看Vue源码学习三 ———— Vue构造函数包装