首先打开idea新建一个springboot项目:file-new project
然后点击next
分别选中web ->web TemplateEngine-> freemarker SQL->jpa
然后next ->finish
pom文件如下`<?xml version="1.0" encoding="UTF-8"?>
4.0.0
<groupId>com.example</groupId>
<artifactId>springboot</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>springboot</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.4.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!--<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
<version>2.0.3.RELEASE</version>
</dependency>-->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</dependency>
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
<version>2.0.4.RELEASE</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.46</version>
</dependency>
<dependency>
<groupId>org.elasticsearch.client</groupId>
<artifactId>transport</artifactId>
<version>5.5.2</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.46</version>
</dependency>
<dependency>
<groupId>org.codehaus.jackson</groupId>
<artifactId>jackson-core-asl</artifactId>
<version>1.9.13</version>
</dependency>
<dependency>
<groupId>org.codehaus.jackson</groupId>
<artifactId>jackson-mapper-asl</artifactId>
<version>1.9.13</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
<version>2.0.3.RELEASE</version>
</dependency>
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>2.9.0</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
` springboot目录结构如下 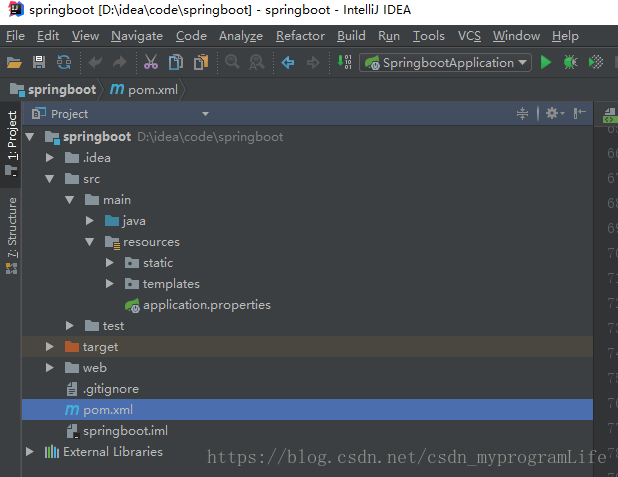 application.properties配置文件如下:
#基本配置
server.port=8080
server.servlet.context-path=/springBoot
#spring.mvc.view.prefix=classpath:/templates/
#spring.mvc.view.suffix=.jsp
#spring.freemarker.prefix=classpath:/templates/
spring.freemarker.suffix=.html
spring.freemarker.request-context-attribute=rc
spring.freemarker.expose-request-attributes=true
spring.freemarker.settings.auto_import=common/include.html as common
#MySQL
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/mytest?characterEncoding=utf8
spring.datasource.username=root
spring.datasource.password=root
#jpa
spring.jpa.show-sql=true
spring.jpa.hibernate.ddl-auto=update
jpa.hibernate.dialect = org.hibernate.dialect.MySQL5Dialect
jpa如下:src->main->java->包名
@Repository
public interface StudengRepository extends JpaRepository<Student,Integer> {
Student findStudentByAge(Integer age);
}
service :
public interface StudentService {
List<Student>getList();
void add(Student student);
Student findByAge(Integer age);
void delete(Student student);
List<Student> findAll();
}
service实现类:
@Service
public class StudentServiceImpl implements StudentService {
@Autowired
private StudengRepository studengRepository;
@Override
public List<Student> getList() {
return studengRepository.findAll();
}
@Override
public void add(Student student) {
Student student1=new Student();
student1.setAge(26);
student1.setName("li");
student1.setScore(100);
student1.setSex("女");
studengRepository.save(student1);
}
@Override
public Student findByAge(Integer age) {
return studengRepository.findStudentByAge(age);
}
@Override
public void delete(Student student) {
studengRepository.delete(student);
}
@Override
public List<Student> findAll() {
return studengRepository.findAll();
}
}
controller如下:
@Controller
public class StudentController {
@Autowired
private StudentService studentService;
/* @GetMapping(value = "/getList")
@ResponseBody
public List<Student> getList(){
List<Student> students=studentService.getList();
return students;
}*/
@RequestMapping(value = "/list")
public ModelAndView show(Model model, ModelAndView modelAndView) {
modelAndView.setViewName("student_list");
List<Student> students = studentService.findAll();
model.addAttribute("student", students);
return modelAndView;
}
@GetMapping(value = "/delete/{id}")
@ResponseBody
public void delete(Student student){
studentService.delete(student);
}
freemarker如下:list页面如下
<h1 class="page-header" style="text-align: center">
<small>学生管理</small>
</h1>
<link href="${rc.contextPath}/assets/plugins/jquery-ui/themes/base/minified/jquery-ui.min.css" rel="stylesheet"/>
<link href="${rc.contextPath}/assets/plugins/bootstrap/css/bootstrap.min.css" rel="stylesheet"/>
<link href="${rc.contextPath}/assets/plugins/font-awesome/css/font-awesome.min.css" rel="stylesheet"/>
<link href="${rc.contextPath}/assets/css/animate.min.css" rel="stylesheet"/>
<link href="${rc.contextPath}/assets/css/style.min.css" rel="stylesheet"/>
<link href="${rc.contextPath}/css/style.css" rel="stylesheet"/>
<link href="${rc.contextPath}/assets/css/style-responsive.min.css" rel="stylesheet"/>
<link href="${rc.contextPath}/assets/css/theme/default.css" rel="stylesheet" id="theme"/>
<!-- ================== END BASE CSS STYLE ================== -->
<link href="${rc.contextPath}/assets/plugins/datetimepicker/css/jquery.datetimepicker.css" rel="stylesheet"/>
<!-- ================== BEGIN PAGE LEVEL CSS STYLE ================== -->
<link href="${rc.contextPath}/assets/plugins/jquery-jvectormap/jquery-jvectormap-1.2.2.css" rel="stylesheet"/>
<link href="${rc.contextPath}/assets/plugins/bootstrap-calendar/css/bootstrap_calendar.css" rel="stylesheet"/>
<link href="${rc.contextPath}/assets/plugins/gritter/css/jquery.gritter.css" rel="stylesheet"/>
<link href="${rc.contextPath}/assets/plugins/morris/morris.css" rel="stylesheet"/>
<!-- ================== END PAGE LEVEL CSS STYLE ================== -->
<!-- ================== BEGIN BASE JS ================== -->
<script src="${rc.contextPath}/assets/plugins/pace/pace.min.js"></script>
<!-- ================== END BASE JS ================== -->
<link href="${rc.contextPath}/assets/plugins/datatables/css/data-table.css" rel="stylesheet"/>
<script src="${rc.contextPath}/assets/plugins/charts/highcharts.js"></script>
<link href="${rc.contextPath}/assets/plugins/bootstrap-wizard/css/bwizard.min.css" rel="stylesheet"/>
<div class="panel panel-inverse" data-sortable-id="table-basic-4">
<div class="panel-heading">
<h4 class="panel-title">学生列表</h4>
</div>
<div class="panel-body">
<table class="table table-striped" width="100%" border="1" cellpadding="0" cellspacing="0" style="text-align:center;">
<thead>
<tr >
<th>学生编号</th>
<th>学生名称</th>
<th>学生年龄</th>
<th>学生性别</th>
<th>学生成绩</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<#list student as ct>
<tr >
<td>${ct.id}</td>
<td>${ct.name}</td>
<td>${ct.age}</td>
<td>${ct.sex}</td>
<td>${ct.score}</td>
<td>
<a href="detail/${ct.id}" class="btn btn-success m-r-5"><i class="fa fa-search-plus"></i>详情</a>
<a href="toadd"><i class="fa fa-search-plus"></i>添加</a>
<a href="toedit/${ct.id}"><i class="fa fa-search-plus"></i>修改</a>
<a href="javascript:void(0);" onclick=deletestudent(${ct.id});>删除</a>
<a href="javascript:void(0);" onclick=editStudent('${ct.id}','${ct.name}','${ct.sex}','${ct.age}','${ct.score}');><i class="fa fa-search-plus"></i>修改</a>
<button type="button" class="btn btn-primary m-r-5" onclick="exportStudent()" >导出</button>
<button type="button" class="btn btn-primary m-r-5" onclick="importStudent()" >导入</button>
</td>
</tr>
</#list>
</table>
</div>
</div>
<#--
<script src="http://localhost:8081/demo/js/jquery-3.0.0.min.js"></script>
<script src="${rc.contextPath}/layer/layer.js"></script>
<script src="http://localhost:8081/demo/js/index.js"></script>-->
<#--
<script src="http://localhost:8081/demo/js/bootstrap-modal.js"></script>-->
<script src="${rc.contextPath}/assets/plugins/jquery/jquery-migrate-1.1.0.min.js"></script>
<script src="${rc.contextPath}/assets/plugins/jquery-ui/ui/minified/jquery-ui.min.js"></script>
<script src="${rc.contextPath}/assets/plugins/bootstrap/js/bootstrap.min.js"></script>
<script src="${rc.contextPath}/assets/plugins/slimscroll/jquery.slimscroll.min.js"></script>
<script src="${rc.contextPath}/assets/plugins/datetimepicker/js/jquery.datetimepicker.full.min.js"></script>
<script src="${rc.contextPath}/assets/plugins/morris/raphael.min.js"></script>
<script src="${rc.contextPath}/assets/plugins/morris/morris.js"></script>
<script src="${rc.contextPath}/assets/plugins/jquery-jvectormap/jquery-jvectormap-1.2.2.min.js"></script>
<script src="${rc.contextPath}/assets/plugins/jquery-jvectormap/jquery-jvectormap-world-merc-en.js"></script>
<script src="${rc.contextPath}/assets/plugins/bootstrap-calendar/js/bootstrap_calendar.min.js"></script>
<script src="${rc.contextPath}/assets/plugins/gritter/js/jquery.gritter.js"></script>
<script src="${rc.contextPath}/assets/plugins/bootstrap-wizard/js/bwizard.js"></script>
<script src="${rc.contextPath}/assets/js/apps.min.js"></script>
<script src="${rc.contextPath}/js/custom/ajaxfileupload.js" type="text/javascript" charset="UTF-8"></script>
<script src="${rc.contextPath}/js/custom/custom.js" type="text/javascript" charset="UTF-8"></script>
<script src="${rc.contextPath}/js/custom/picUpload.js" type="text/javascript" charset="UTF-8"></script>
<script src="${rc.contextPath}/js/custom/yabeiAjax.js" type="text/javascript" charset="UTF-8"></script>
<script src="${rc.contextPath}/js/custom/qiniuUpload.js" type="text/javascript" charset="UTF-8"></script>
<script src="${rc.contextPath}/assets/plugins/bootstrap-wizard/js/bwizard.js"></script>
<script>
function deletestudent(id) {
layer.confirm("确认要删除吗?", {
btn: ['确定', '关闭'] //按钮
}, function () {
$.ajax({
url:'delete/'+id
,type:"GET"
,success:function(data){
if(data==true){
alert("success");
window.location.href="/list";
}
}
})
}, function () {
$.close();
});
}
}
最后运行项目:http://localhost:8080/springBoot/list
整合完毕