小弟这学期的JSP课程上完了,课程作业是学生信息管理系统,在开发过程遇到了许多问题,记录下来,作为自己学习的总结,也可以给大家作为参考。如果有错误的地方,多多包涵。
github地址如下:
https://github.com/XiaoZhong233/StudentManagerSystem-JSP-
一、新建JAVA项目
File->New->DynamicWebProject
然后就是配置服务器,我用的是Tomcat,配置很简单,到官网下载好之后在项目新建中添加进去就可以了
二、引入Mysql的jar包
因为要用到数据库和服务端交互,因此要引入mysql的jar包,然后在项目中利用JDBC技术操作数据库就可以了
mysql的jar包在官网可以找到,我也上传到了百度网盘,分享给大家
https://pan.baidu.com/s/1YfucuLovNKBa4JMci1gfCg
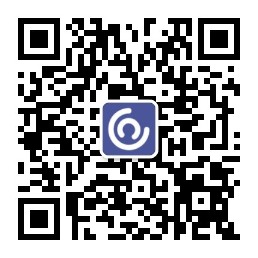
然后在Java Resources中新建一个lib文件夹,把mysql的jar包粘贴到这里面;
右键,如上图一样选择Add to Build Path就可以了,为了保险起见,可以在WebContent的lib文件中也这样引入一个sql的jar包。
三、建立数据库连接工具
把项目构建好了,引入了需要用的jar包,我们就可以开始进行开发了。我们与服务端交互,首先就要有个工具,来打开与数据库的连接。话不多说,直接上代码
package javabean;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class ConnDB {
private static String URL = "jdbc:mysql://localhost:3306/"; //数据库连接 "student"是数据库名
private static String USER = "root";//数据库账号
private static String PASSWORD = "root";//数据库密码
private Connection conn = null;
/**
* 无参构造函数默认的数据库为student
*/
public ConnDB() {
try {
URL="jdbc:mysql://localhost:3306/student?characterEncoding=utf-8";
Class.forName("com.mysql.jdbc.Driver");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
public ConnDB(String databaseName) {
try {
Class.forName("com.mysql.jdbc.Driver");
URL=URL+databaseName+"?characterEncoding=utf-8";
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
//获取数据库连接
public Connection getConnction() {
try {
conn = DriverManager.getConnection(URL, USER, PASSWORD);
} catch (SQLException e) {
e.printStackTrace();
}
return conn;
}
//关闭数据库连接
public void closeCon(Connection con) throws Exception{
if(con!=null){
con.close();
}
}
/**
* 根据传入的SQL语句返回一个结果集
*
* @param sql
* @return
* @throws Exception
*/
public ResultSet select(String sql) throws Exception {
Statement stmt = null;
ResultSet rs = null;
try {
stmt = conn.createStatement();
rs = stmt.executeQuery(sql);
return rs;
} catch (SQLException sqle) {
throw new SQLException("select data exception: "
+ sqle.getMessage());
} catch (Exception e) {
throw new Exception("System e exception: " + e.getMessage());
}
}
/**
* 根据传入的SQL语句向数据库增加一条记录
*
* @param sql
* @throws Exception
*/
public void insert(String sql) throws Exception {
PreparedStatement ps = null;
try {
ps = conn.prepareStatement(sql);
ps.executeUpdate();
} catch (SQLException sqle) {
throw new Exception("insert data exception: " + sqle.getMessage());
} finally {
try {
if (ps != null) {
ps.close();
}
} catch (Exception e) {
throw new Exception("ps close exception: " + e.getMessage());
}
}
try {
if (conn != null) {
conn.close();
}
} catch (Exception e) {
throw new Exception("connection close exception: " + e.getMessage());
}
}
/**
* 根据传入的SQL语句更新数据库记录
*
* @param sql
* @throws Exception
*/
public void update(String sql) throws Exception {
PreparedStatement ps = null;
try {
ps = conn.prepareStatement(sql);
ps.executeUpdate();
} catch (SQLException sqle) {
throw new Exception("update exception: " + sqle.getMessage());
} finally {
try {
if (ps != null) {
ps.close();
}
} catch (Exception e) {
throw new Exception("ps close exception: " + e.getMessage());
}
}
try {
if (conn != null) {
conn.close();
}
} catch (Exception e) {
throw new Exception("connection close exception: " + e.getMessage());
}
}
/**
* 根据传入的SQL语句删除一条数据库记录
*
* @param sql
* @throws Exception
*/
public void delete(String sql) throws Exception {
PreparedStatement ps = null;
try {
ps = conn.prepareStatement(sql);
ps.executeUpdate();
} catch (SQLException sqle) {
throw new Exception("delete data exception: " + sqle.getMessage());
} finally {
try {
if (ps != null) {
ps.close();
}
} catch (Exception e) {
throw new Exception("ps close exception: " + e.getMessage());
}
}
try {
if (conn != null) {
conn.close();
}
} catch (Exception e) {
throw new Exception("connection close exception: " + e.getMessage());
}
}
public static void main(String[] args) {
ConnDB connDB=new ConnDB();
try {
Connection connection=connDB.getConnction();
System.out.println("连接成功");
Statement stmt=connection.createStatement();
ResultSet rs=stmt.executeQuery("select * from studentdata");
while(rs.next()){
System.out.println(rs.getString(1));
}
connDB.closeCon(connection);
} catch (Exception e) {
// TODO: handle exception
}
}
}
这里数据库连接的URL为jdbc:mysql://localhost:3306/ 其中localhost:3306为用户名和端口,用':'分隔,用navicat连接到sql后就可以查看(navicat是一个很好用的数据库可视化工具,大家可以去官网下载一个来用)
在navicat中可以看到我们的主机名为localhost,端口号为3306,OK,这就是我们要在jdbc:mysql://后面加的东西。大家可以看到我写了两个构造函数,一个无参,默认连接到student这个库,另一个需要一个String指定数据库名。
拿默认连接来说,也就是student这个库,完整URL为:jdbc:mysql://localhost:3306/student?characterEncoding=utf-8 这里需要注意的是:如果我用jdbc:mysql://localhost:3306/student也是可以连接的上数据库的,但是在JDBC写入数据库的时候,会出现写入的值如果是中文,则会在数据库中乱码,所以我们可以在jdbc:mysql://localhost:3306/student加上?characterEncoding=utf-8指定我们写入时候的编码。
大家在写完这个工具的时候可以在main中测试下,是否连接成功
这样,我们的数据库连接工具就写好了。
四、接下来就是jsp网页了
我先把实现的成果图贴出来
注册
注册成功
然后数据库中就会多出注册的信息(这里我新建了一个user表用来记录用户名和密码)
登陆
密码错误或用户未进行注册时会提醒,并且重新返回登录界面
登录成功后,直接进入学生信息管理系统界面,这里可以看到所有学生的信息(各位同学看到不要打我)
在这个界面,可以对学生信息进行修改,删除,查询操作
添加学生
添加成功后:
系统会倒数,然后自动跳转至信息页面
删除学生
在删除前,系统会弹窗和用户再一次确认是否删除信息,以防误删
删除成功后
可以看到,刚才新增的记录已经被删除了
修改学生
点击修改按钮,即可进入修改页面,修改页面会自动填充好要修改学生的信息,用户只需要少许的改动即可修改信息
e.g.把姓名修改成“陈思成已被修改”
更改后的学生信息
查找学生
查询功能分为按学号查找,按姓名查找,按SQL语句查找
界面做的很简单,只是用来练习,没必要花大精力在界面上
l 按学号查找
l 按姓名查找
l 按SQL语句查找
例如,要查找地信2班的学生的学院,专业,名字信息,SQL语句如下图所示
查询结果如下图所示
系统自动对未加入查询的属性列赋null值
好了,这就是我们要实现的功能,下面就来看代码吧,新建若干个jsp文件
项目代码结构:
每个页面的功能,我已经标注出来了,名字也能看出来哈哈。。
接下来又是贴代码
Login_deal.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>学生管理系统登录页</title>
<script language="javascript">
function check(){
var username=document.getElementById("username");
var password=document.getElementById("password");
if(username.value==""||password.value==""){
alert("用户名或密码不可为空!");
return false;
}
return true;
}
</script>
<script language="javascript">
function regist(){
//跳转至注册页
window.location='register.jsp';
}
</script>
</head>
<body>
<h1 align='center'>学生信息管理系统</h1>
<form action="login_deal.jsp">
<div>
<table align='center'>
<tr>
<td>用户名:</td>
<td colspan='2'><input id="username" name="username" type="text" style="width: 90%; "></td>
</tr>
<tr>
<td>密码:</td>
<td colspan='2'><input id="password" name="password" type="password" style="width: 90%; "></td>
</tr>
<tr>
<td align='center' style="height: 1; "><input type="reset" value="重置"></td>
<td align='center' style="height: 1; "><input type="button" value="注册" onClick="regist()"></td>
<td align='center' style="width: 1; height: 61px"><input type="submit" value="提交" onClick="return check()"></td>
</tr>
</table>
</div>
</form>
</body>
</html>
要注意的是,要把开头三个charset都改成UTF-8,这样才能在JSP中使用中文
这里用了一个脚本来验证用户名密码是否非空。
Register.jsp(注册页面)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>注册管理员</title>
</head>
<body>
<h1 align ="center"> 管理员注册页面</h1>
<form action="registerDone.jsp">
<table align="center">
<tr>
<td>用户名:</td>
<td colspan='2'><input id="username" name="username" type="text" style="width: 90%; "></td>
</tr>
<tr>
<td>密码:</td>
<td colspan='2'><input id="password" name="password" type="password" style="width: 90%; "></td>
</tr>
<tr>
<td align='center' style="height: 1; "><input type="reset" value="重置"></td>
<td align='center' style="height: 1; "><input type="submit" value="注册"></td>
</tr>
</table>
</form>
</body>
</html>
Register_done.jsp(注册处理页面)
<%@page import="javabean.ConnDB"%>
<%@page import="java.sql.*"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<jsp:useBean id="user" class="javabean.User"/>
<jsp:setProperty property="*" name="user"/>
<%request.setCharacterEncoding("UTF-8"); %>
<!--一定要加这个,不然传入的请求参数会乱码 -->
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>注册结果</title>
</head>
<body>
<%
try
{
ConnDB db=new ConnDB();
Connection connection=db.getConnction();
String sql="insert into user values("+user.getUsername()+","+user.getPassword()+")";
db.insert(sql);
out.print("<script>alert('注册成功'); window.location='login.jsp' </script>");
}
catch(Exception e){
e.printStackTrace();
out.print("<script>alert('注册失败!请重新注册'); window.location='register.jsp' </script>");
}
%>
</body>
</html>
Table.css(css文件,用于控制表格布局)
@charset "UTF-8";
body{
padding:0px;
margin:0px;
font-size:12px;
}
body a{
color:#03515d;
text-decoration:none;
}
body button{
color:#03515d;
}
body span{
color:#03515d;
}
.center_bottom input{
color:#03515d;
font-size:12px;
height:20px;
width:40px;
padding:2px;
vertical-align:middle;
text-align:center;
margin-bottom:6px;
}
/**************************布局部分********************/
.table_div{
width:1000px;
padding:10px;
margin:0 auto;
}
.div_clear{
clear:both;
}
.left_top{
background:url("./tab/images/leftbottom.gif");
height:30px;
width:12px;
float:left;
}
.left_center{
background:url("./tab/images/rfcenter.gif");
height:530px;
width:12px;
float:left;
}
.left_bottom{
background:url("./tab/images/leftbottom.gif");
height:35px;
width:12px;
float:left;
}
.center_top{
background:url("./tab/images/center.gif") repeat-x;
height:30px;
line-height:30px;
width:900px;
float:left;
}
.center_center{
height:400px;
width:900px;
float:left;
}
.center_bottom{
background:url("./tab/images/center.gif") repeat-x;
height:35px;
width:900px;
float:left;
line-height:35px;
}
.right_top{
background:url("./tab/images/leftbottom.gif");
height:30px;
width:15px;
float:left;
}
.right_center{
background:url("./tab/images/rfcenter.gif");
height:530px;
width:15px;
float:left;
}
.right_bottom{
background:url("./tab/images/leftbottom.gif");
height:35px;
width:15px;
float:left;
}
/**************************************表格内容***********************************/
.table_content{
margin:5px;
border:1px solid #B5D6E6;
width:890px;
height:520px;
overflow-x:hidden;
overflow-y:auto;
}
.table_content table{
width:100%;
border:0;
border-collapse:collapse;
font-size:12px;
}
.table_content table tr:hover{
background-color:#C1EBFF;
}
.table_content table th{
border-collapse:collapse;
height:22px;
background:url("./tab/images/bg.gif");
border-right:1px solid #B5D6E6;
border-bottom:1px solid #B5D6E6;
}
.table_content table td{
height:22px;
word-wrap:break-word;
max-width:200px;
text-align:center;
vertical-align:middle;
border-right:1px solid #B5D6E6;
border-bottom:1px solid #B5D6E6;
}
studentInfo.jsp(学生信息管理页)
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.Connection"%>
<%@page import="javabean.ConnDB"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%request.setCharacterEncoding("UTF-8"); %>
<!-- 一定要加这个,不然传入的请求参数会乱码 -->
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>学生信息查询结果</title>
<link type="text/css" rel="stylesheet" href="tablecss.css"/>
</head>
<body>
<%
ConnDB connDB=new ConnDB();
Connection connection=connDB.getConnction();
String sql="select * from studentdata";
ResultSet rs=connDB.select(sql);
%>
<div align="center">
<font size="4"><strong> 学生信息:</strong><br>
下面的表格就是查询结果:
</font><br>
</div>
<div class="table_div">
<!-- 导航栏部分 -->
<div class="div_clear">
<div class="left_top"></div>
<div class="center_top">
<div style="float:left">
<img src="./tab/images/tb.gif" width="16px" height="16px" style="vertical-align:middle"/>
<span style="font-weight:bold">你当前的位置</span>:[学生信息管理系统]-[学生信息表]
</div>
<div style="float:right;padding-right:50px">
<img width='16' height='16' src="./tab/images/query.png" style="vertical-align:middle"/>
<a href="query.jsp"><strong>查找学生记录</strong></a>
</div>
<div style="float:right;padding-right:50px">
<img width='16' height='16' src="./tab/images/add.gif" style="vertical-align:middle"/>
<a href="Insert.jsp"><strong>新增学生记录</strong></a>
</div>
</div>
<div class="right_top"></div>
</div>
<!-- 导航栏部分结束 -->
<!-- 表格部分 -->
<div class="div_clear">
<div class="left_center"></div>
<!-- 表格内容块 -->
<div class="center_center">
<div class="table_content">
<table cellspacing="0px" cellpadding="0px">
<!-- 表头 -->
<thead>
<tr>
<th width="16%">学院名称</th>
<th width="16%">专 业</th>
<th width="16%">班 级</th>
<th width="16%">学 号</th>
<th width="16%">姓 名</th>
<th width="20%" style="border-right:none">操作</th>
</tr>
</thead>
<!-- 表体 -->
<tbody>
<%
int count=0;
while(rs.next()){
out.print("<tr>");
out.print("<td>" + rs.getString(1).toString()+ "</td>");
out.print("<td>" + rs.getString(2).toString() + "</td>");
out.print("<td>" + rs.getString(3).toString() + "</td>");
out.print("<td>" + rs.getString(4).toString() + "</td>");
out.print("<td>" + rs.getString(5).toString() + "</td>");
out.print("<td width='20%' style='border-right:none'><img width='16' height='16' src=\"./tab/images/delete.gif\" ><a href=Delete.jsp?id="+rs.getString(6) + " onclick=\"return window.confirm('是否确定删除?')\" >删除</a>");
out.print("<img width='16' height='16' src=\"./tab/images/edit.gif\" > <a href=Update.jsp?id="+rs.getString(6) + " onclick=\"return window.confirm('是否确定编辑?')\" >编辑</a></td>");
out.print("</tr>");
count++;
}
%>
<!-- 表尾 -->
<tfoot>
</tfoot>
</table>
</div>
</div>
<div class="right_center"></div>
</div>
<!-- 底部框 -->
<div class="div_clear">
<div class="left_bottom"></div>
<div class="center_bottom">
<span> 共有<%=count %>条记录,当前第 1/1 页</span>
<div style="float:right;padding-right:30px">
<input type="button" value="首页"/>
<input type="button" value="上页"/>
<input type="button" value="下页"/>
<input type="button" value="尾页"/>
<span>跳转到</span>
<input type="text" size="1"/>
<input type="button" value="跳转"/>
</div>
</div>
<div class="right_bottom"></div>
</div>
</div>
<%
if (rs != null) {
rs.close();
}
%>
</body>
这里就从数据库读取数据,然后输出成表格
Insert.jsp(增加学生记录页)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<script>
function check(form){
if (form.academy.value == "") {
alert("学院名称不能为空");
return false;
}
if (form.major.value == "") {
alert("专业不能为空");
return false;
}
if (form.classs.value == "") {
alert("班级不能为空");
return false;
}
if (form.name.value == "") {
alert("姓名不能为空");
return false;
}
//验证数字的正则表达式
if (form.sno.value == "") {
alert("学号不能为空");
return false;
}
else
{
var reg=/^\d+$/;
var number=form.sno.value;
if(number.match(reg)){
}
else
{
alert("学号必须为纯数字");
return false;
}
}
return true;
}
</script>
<title>添加学生信息记录</title>
</head>
<body>
<h1 align="center">添加学生信息记录</h1>
<br>
<br>
<form action="insertDeal.jsp" method="post" onsubmit="return check(this)">
<table align="center" border="" cellpadding="10" bordercolor="#89cff0" >
<tr>
<td>学院名称:</td>
<td><input type="text" name="academy" /></td>
</tr>
<tr>
<td>专 业:</td>
<td><input type="text" name="major" /></td>
</tr>
<tr>
<td>班 级:</td>
<td><input type="text" name="classs" /></td>
</tr>
<tr>
<td>学 号:</td>
<td><input type="text" name="sno"/></td>
</tr>
<tr>
<td>姓 名:</td>
<td><input type="text" name="name"/></td>
</tr>
<tr>
<td align='center' style="height: 1; "><input type="reset" value="重置"></td>
<td colspan="2" align="right"><input type="submit" value="增 加"></td>
</tr>
</table>
</form>
</body>
</html>
InsertDeal.jsp(添加学生信息处理页)
<%@page import="javabean.ConnDB"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%request.setCharacterEncoding("UTF-8"); %>
<!-- 一定要加这个,不然传入的请求参数会乱码 -->
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<script type="text/javascript">
function countDown(secs,surl){
var jumpTo = document.getElementById('jumpTo');
jumpTo.innerHTML="<strong>"+secs+"</strong>";
if(--secs>0){
setTimeout("countDown("+secs+",'"+surl+"')",1000);
}
else{
location.href=surl;
-ma
}
}
</script>
<title>学生信息添加页</title>
</head>
<body>
<%
String academy=request.getParameter("academy");
String major=request.getParameter("major");
String classs=request.getParameter("classs");
String sno=request.getParameter("sno");
String name=request.getParameter("name");
try{
ConnDB db=new ConnDB();
db.getConnction();
//String sql="insert into studentdata values('"+academy+"','"+major+"','"+classs+"','"+sno+"','"+name+"')";
String sql=String.format("insert into studentdata (academy,major,class,sno,name)"+
"values('%s','%s','%s','%s','%s')",academy,major,classs,sno,name);
System.out.println(sql);
db.insert(sql);
out.print(" <div center='align' width='100%'><font size='5'><strong> 成功添加学生信息"+
"<br><a href='studentInfo.jsp'> <span id='jumpTo'>3</span>秒后系统会自动跳转,也可点击本处直接跳查看当前数据库数据</a> "+
"</strong><br> </font></div>");
String jsscript="<script type='text/javascript'> countDown(3,'studentInfo.jsp'); </script> ";
out.print(jsscript);
}
catch(Exception e){
e.printStackTrace();
out.print(" <div center='align' width='100%'><font size='5' color='red'><strong> 添加失败"+
"<br><a href='Insert.jsp'> <span id='jumpTo'>3</span>秒后系统会自动跳转,也可点击本处直接跳转重新添加</a> "+
"</strong><br> </font></div>");
String jsscript="<script type='text/javascript'> countDown(3,'Insert.jsp'); </script> ";
out.print(jsscript);
}
%>
</body>
</html>
Update.jsp(信息更新页)
<%@page import="java.sql.*"%>
<%@page import="javabean.ConnDB"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<link type="text/css" rel="stylesheet" href="tablecss.css"/>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>更新学生信息</title>
<script type="text/javascript">
function check(form) {
if (form.academy.value == "") {
alert("学院名称不能为空");
return false;
}
if (form.major.value == "") {
alert("专业不能为空");
return false;
}
if (form.classs.value == "") {
alert("班级不能为空");
return false;
}
if (form.name.value == "") {
alert("姓名不能为空");
return false;
}
if (form.sno.value == "") {
alert("学号不能为空");
return false;
}
else
{
var reg=/^\d+$/;
var number=form.sno.value;
if(number.match(reg)){
}
else
{
alert("学号必须为纯数字");
return false;
}
}
return true;
}
</script>
</head>
<body>
<%
ConnDB db=new ConnDB();
db.getConnction();
String id=request.getParameter("id");
String sql="select * from studentdata where id='"+id+ "'";
ResultSet rs=db.select(sql);
rs.next();
%>
<h1 align="center">修改学生信息页</h1>
<form action="updateDeal.jsp" method="post" onsubmit=" return check(this)" >
<div>
<table align="center" border="" cellpadding="10" bordercolor="#89cff0" >
<tr><td>学院名称:</td>
<td><input type="text" name="academy" value=<%="'"+rs.getString(1)+"'"%>/></td>
</tr>
<tr><td>专 业:</td>
<td><input type="text" name="major" value=<%="'"+rs.getString(2)+"'"%>/></td>
</tr>
<tr><td>班 级:</td>
<td><input type="text" name="classs" value=<%="'"+rs.getString(3)+"'"%>/></td>
</tr>
<tr><td>学 号:</td>
<td><input type="text" name="sno" value=<%="'"+rs.getString(4)+"'"%>/></td>
</tr>
<tr><td>姓 名:</td>
<td><input type="text" name="name" value=<%="'"+rs.getString(5)+"'"%>/></td>
</tr>
<tr><td colspan="2" align="right"><input type="submit" value="修 改"></td></tr>
</table>
<input type="hidden" name="id" value=<%="'"+rs.getString(6)+"'"%>/>
<%
if(rs!=null){
rs.close();
}
%>
</div>
</form>
</body>
</html>
UpdateDeal.jsp(信息更新处理页)
<%@page import="javabean.ConnDB"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%request.setCharacterEncoding("UTF-8"); %>
<!-- 一定要加这个,不然传入的请求参数会乱码 -->
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>更新学生信息</title>
<script>
function countDown(secs,surl){
var jumpTo = document.getElementById('jumpTo');
jumpTo.innerHTML="<strong>"+secs+"</strong>";
if(--secs>0){
setTimeout("countDown("+secs+",'"+surl+"')",1000);
}
else{
location.href=surl;
-ma
}
}
</script>
</head>
<body>
<%
String academy=request.getParameter("academy");
String major=request.getParameter("major");
String classs=request.getParameter("classs");
String sno=request.getParameter("sno");
String name=request.getParameter("name");
int id=Integer.parseInt(request.getParameter("id"));
System.out.println(id);
System.out.println(academy);
System.out.println(major);
System.out.println(classs);
System.out.println(sno);
System.out.println(name);
ConnDB db=new ConnDB();
db.getConnction();
String sql="update studentdata set academy='"+academy+"', major='"+major+"',class='"+classs+"',sno='"+sno+"',name='"+name+"' where id="+id;
try{
db.update(sql);
out.print(" <div center='align' width='100%'><font size='5'><strong> 成功更新学生信息"+
"<br><a href='studentInfo.jsp'> <span id='jumpTo'>3</span>秒后系统会自动跳转,也可点击本处直接跳查看当前数据库数据</a> "+
"</strong><br> </font></div>");
String jsscript="<script type='text/javascript'> countDown(3,'studentInfo.jsp'); </script> ";
out.print(jsscript);
}
catch(Exception e){
e.printStackTrace();
out.print("<script>alert(更新失败!)</script>");
out.print(" <div center='align' width='100%'><font size='5' color='red'><strong> 更新失败"+
"<br><a href='studentInfo.jsp'> <span id='jumpTo'>3</span>秒后系统会自动跳转,也可点击本处直接跳转</a> "+
"</strong><br> </font></div>");
String jsscript="<script type='text/javascript'> countDown(3,'studentInfo.jsp'); </script> ";
out.print(jsscript);
}
%>
<font size="2"><strong> 成功修改数据!
<a href="studentInfo.jsp">查看当前数据库数据</a>
</strong><br> </font>
</body>
</html>
Delete.jsp(删除信息页面)
<%@page import="javabean.ConnDB"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%request.setCharacterEncoding("UTF-8"); %>
<!-- 一定要加这个,不然传入的请求参数会乱码 -->
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>学生信息删除页面</title>
<script>
function countDown(secs,surl){
var jumpTo = document.getElementById('jumpTo');
jumpTo.innerHTML="<strong>"+secs+"</strong>";
if(--secs>0){
setTimeout("countDown("+secs+",'"+surl+"')",1000);
}
else{
location.href=surl;
-ma
}
}
</script>
</head>
<body>
<%
try
{
ConnDB db=new ConnDB();
db.getConnction();
String id=request.getParameter("id");
System.out.println(id);
String sql_delete="delete from studentdata where id= '" +id+"'";
db.delete(sql_delete);
out.print(" <div center='align' width='100%'><font size='5'><strong> 成功删除学生信息"+
"<br><a href='studentInfo.jsp'> <span id='jumpTo'>3</span>秒后系统会自动跳转,也可点击本处直接跳查看当前数据库数据</a> "+
"</strong><br> </font></div>");
String jsscript="<script type='text/javascript'> countDown(3,'studentInfo.jsp'); </script> ";
out.print(jsscript);
}
catch(Exception e){
e.printStackTrace();
out.print("<script>alert(删除失败!)</script>");
out.print(" <div center='align' width='100%'><font size='5' color='red'><strong> 添加失败"+
"<br><a href='studentInfo.jsp'> <span id='jumpTo'>3</span>秒后系统会自动跳转,也可点击本处直接跳转重新删除</a> "+
"</strong><br> </font></div>");
String jsscript="<script type='text/javascript'> countDown(3,'studentInfo.jsp'); </script> ";
out.print(jsscript);
}
%>
</body>
</html>
Query.jsp(查询页面)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>选择查询方式</title>
<script language='javascript'>
function byxuehao(){
window.location='queryByXueHao.jsp';
}
</script>
<script language='javascript'>
function byxingming(){
window.location='queryByXingMing.jsp';
}
</script>
<script language='javascript'>
function bysql(){
window.location='queryBySQL.jsp';
}
</script>
</head>
<body>
<table align="center" cellpadding="5" cellspacing="5" >
<tr>
<td>
<input type="button" value="按照学号查找" onClick="byxuehao()" style="width: 165px; ">
</td>
</tr>
<tr>
<td>
<input type="button" value="按照姓名查找" onClick="byxingming()" style="width: 165px; ">
</td>
</tr>
<tr>
<td>
<input type="button" value="按SQL语句查找" onClick="bysql()" style="width: 165px; ">
</td>
</tr>
</table>
</body>
</html>
queryByXueHao.jsp(按学号查询页面)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<script type="text/javascript">
function check(form) {
if (form.xuehao.value == "") {
alert("学号不能为空");
return false;
}
else
{
var reg=/^\d+$/;
var number=form.xuehao.value;
if(number.match(reg)){
}
else
{
alert("学号必须为纯数字");
return false;
}
}
return true;
}
</script>
<title>学生信息查询</title>
</head>
<body>
<h1 align="center">
请输入学号,进行查询
</h1>
<form action="queryByXueHaoDone.jsp" onsubmit=" return check(this)">
<table align="center" cellpadding="5" cellspacing="5">
<tr>
<td>学号:</td>
<td colspan='2'><input id="xuehao" name="xuehao" type="text" style="width: 90%; "></td>
</tr>
<tr style="width: 312px; ">
<td align='center' style="height: 1; "></td>
<td align='center' style="height: 1; width: 182px"><input type="reset" value="重置">
<input type="submit" value="查询"></td>
</tr>
</table>
</form>
</body>
</html>
QueryByXingMing.jsp(按姓名查询)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>学生信息查询</title>
</head>
<body>
<h1 align="center">
请输入姓名,进行查询
</h1>
<form action="queryByXingMingDone.jsp" >
<table align="center" cellpadding="5" cellspacing="5">
<tr>
<td>姓名:</td>
<td colspan='2'><input id="name" name="name" type="text" style="width: 90%; "></td>
</tr>
<tr style="width: 312px; ">
<td align='center' style="height: 1; "></td>
<td align='center' style="height: 1; width: 182px"><input type="reset" value="重置">
<input type="submit" value="查询"></td>
</tr>
</table>
</form>
</body>
</html>
QueryByXingMingDone.jsp(姓名查询界面)
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.Connection"%>
<%@page import="javabean.ConnDB"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%request.setCharacterEncoding("UTF-8"); %>
<!-- 一定要加这个,不然传入的请求参数会乱码 -->
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<script>
function countDown(secs,surl){
var jumpTo = document.getElementById('jumpTo');
jumpTo.innerHTML="<strong>"+secs+"</strong>";
if(--secs>0){
setTimeout("countDown("+secs+",'"+surl+"')",1000);
}
else{
location.href=surl;
-ma
}
}
</script>
<title>学生信息查询结果</title>
<link type="text/css" rel="stylesheet" href="tablecss.css"/>
</head>
<body>
<%
String name=request.getParameter("name");
ConnDB connDB=new ConnDB();
Connection connection=connDB.getConnction();
String sql="select * from studentdata where name='"+name+"'";
ResultSet rs=null;
try{
rs=connDB.select(sql);
}catch(Exception e){
e.printStackTrace();
out.print("<script>alert(\"查询失败\")</script>");
out.print("window.location='query.jsp'");
}
%>
<div align="center">
<font size="4"><strong> 学生信息:</strong><br>
下面的表格就是查询结果:
</font><br>
</div>
<div class="table_div">
<!-- 导航栏部分 -->
<div class="div_clear">
<div class="left_top"></div>
<div class="center_top">
<div style="float:left">
<img src="./tab/images/tb.gif" width="16px" height="16px" style="vertical-align:middle"/>
<span style="font-weight:bold">你当前的位置</span>:<a href='studentInfo.jsp'>[学生信息管理系统]</a>-<a href='query.jsp'>[学生查询]</a>-[按姓名语句查询]
</div>
<div style="float:right;padding-right:50px">
<img width='16' height='16' src="./tab/images/query.png" style="vertical-align:middle"/>
<a href="query.jsp"><strong>查找学生记录</strong></a>
</div>
<div style="float:right;padding-right:50px">
<img width='16' height='16' src="./tab/images/add.gif" style="vertical-align:middle"/>
<a href="Insert.jsp"><strong>新增学生记录</strong></a>
</div>
</div>
<div class="right_top"></div>
</div>
<!-- 导航栏部分结束 -->
<!-- 表格部分 -->
<div class="div_clear">
<div class="left_center"></div>
<!-- 表格内容块 -->
<div class="center_center">
<div class="table_content">
<table cellspacing="0px" cellpadding="0px">
<!-- 表头 -->
<thead>
<tr>
<th width="16%">学院名称</th>
<th width="16%">专 业</th>
<th width="16%">班 级</th>
<th width="16%">学 号</th>
<th width="16%">姓 名</th>
<th width="20%" style="border-right:none">操作</th>
</tr>
</thead>
<!-- 表体 -->
<tbody>
<%
int count=0;
if(!rs.next()){
out.print("<tr>");
out.print("<td colspan=6>");
out.print(" <div center='align' width='100%'><font size='5' color='red'><strong> 查询失败:未查询到该学生记录"+
"<br><a href='query.jsp'> <span id='jumpTo'>8</span>秒后系统会自动跳转,也可点击本处直接跳转重新查询</a> "+
"</strong><br> </font></div>");
String jsscript="<script type='text/javascript'> countDown(8,'query.jsp'); </script> ";
out.print(jsscript);
out.print("</td>");
out.print("</tr>");
}
else{
rs.beforeFirst();
while(rs.next()){
out.print("<tr>");
out.print("<td>" + rs.getString(1).toString()+ "</td>");
out.print("<td>" + rs.getString(2).toString() + "</td>");
out.print("<td>" + rs.getString(3).toString() + "</td>");
out.print("<td>" + rs.getString(4).toString() + "</td>");
out.print("<td>" + rs.getString(5).toString() + "</td>");
out.print("<td width='20%' style='border-right:none'><img width='16' height='16' src=\"./tab/images/delete.gif\" ><a href=Delete.jsp?id="+rs.getString(6) + " onclick=\"return window.confirm('是否确定删除?')\" >删除</a>");
out.print("<img width='16' height='16' src=\"./tab/images/edit.gif\" > <a href=Update.jsp?id="+rs.getString(6) + " onclick=\"return window.confirm('是否确定编辑?')\" >编辑</a></td>");
out.print("</tr>");
count++;
}
}
%>
<!-- 表尾 -->
<tfoot>
</tfoot>
</table>
</div>
</div>
<div class="right_center"></div>
</div>
<!-- 底部框 -->
<div class="div_clear">
<div class="left_bottom"></div>
<div class="center_bottom">
<span> 共有<%=count %>条记录,当前第 1/1 页</span>
<div style="float:right;padding-right:30px">
<input type="button" value="首页"/>
<input type="button" value="上页"/>
<input type="button" value="下页"/>
<input type="button" value="尾页"/>
<span>跳转到</span>
<input type="text" size="1"/>
<input type="button" value="跳转"/>
</div>
</div>
<div class="right_bottom"></div>
</div>
</div>
<%
if (rs != null) {
rs.close();
}
%>
</body>
</html>
QueryBySQL.jsp(按照SQL语句进行查询)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>学生信息查询</title>
<style type='text/css'>
.main{
text-align: center; /*让div内部文字居中*/
background-color: #fff;
opacity:1;
border-radius: 20px;
width: 50%;
height: 370px;
margin: auto;
position: relative;
top: 0;
left: 0;
right: 0;
bottom: 0;
border: 2px solid black;
}
</style>
<style type='text/css'>
.leftDiv{
text-align: left; /*让div内部文字居中*/
width: 100%;
height: 100%;
left:0;
opacity:1;
top:27%;
position: absolute;
float:left;
}
</style>
<script language='javascript'>
function check(form){
var text = document.getElementById('sql').value.length;
if(text==0){
alert("SQL语句不可为空");
return false;
}
return true;
}
</script>
</head>
<body>
<h1 align='center'>使用SQL查询语句进行查找</h1>
<div class='main' >
<br>
<div>
<form action="queryBySQLDone.jsp" onsubmit=" return check(this)" >
<table align="left" cellpadding="5" cellspacing="5">
<tr>
<td colspan='2'>SQL语句: </td>
<td colspan='6'><textarea name="sql" id='sql' cols="28" rows="5" wrap="virtual"></textarea></td>
<td align='center' style="height: 1; "></td>
<td align='center' style="height: 1; width: 182px"><input type="submit" value="查询">
<input type="reset" value="重置"></td>
</tr>
</table>
</form>
</div>
<br>
<div class='leftDiv' >
<h5 align=center>以下是注意事项</h5>
<p>只支持简单查询,例如select [属性] from [表] where [约束]。不支持连接查询</p>
<font size='5' >
<ul>
<li >学生信息表名称---><Strong>studentdata</Strong></li>
<li>属性列:academy--->学院名</li>
<li>属性列:major--->专业名</li>
<li>属性列:class--->班级</li>
<li>属性列:sno--->学号</li>
<li>属性列:name--->姓名</li>
</ul>
</font>
</div>
</div>
<br>
<br>
</body>
</html>
QueryBySQLDone.jsp(按SQL语句查询结果)
<%@page import="java.util.*"%>
<%@page import="java.sql.ResultSetMetaData"%>
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.Connection"%>
<%@page import="javabean.ConnDB"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%request.setCharacterEncoding("UTF-8"); %>
<!-- 一定要加这个,不然传入的请求参数会乱码 -->
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<script>
function countDown(secs,surl){
var jumpTo = document.getElementById('jumpTo');
jumpTo.innerHTML="<strong>"+secs+"</strong>";
if(--secs>0){
setTimeout("countDown("+secs+",'"+surl+"')",1000);
}
else{
location.href=surl;
-ma
}
}
</script>
<title>学生信息查询结果</title>
<link type="text/css" rel="stylesheet" href="tablecss.css"/>
</head>
<body>
<%
String sql=request.getParameter("sql");
ConnDB connDB=new ConnDB();
Connection connection=connDB.getConnction();
ResultSet rs=null;
try{
rs=connDB.select(sql);
}catch(Exception e){
e.printStackTrace();
out.print("<script>alert(\"查询失败\")</script>");
out.print("window.location='query.jsp'");
}
%>
<div align="center">
<font size="4"><strong> 学生信息:</strong><br>
下面的表格就是查询结果:
</font><br>
</div>
<div class="table_div">
<!-- 导航栏部分 -->
<div class="div_clear">
<div class="left_top"></div>
<div class="center_top">
<div style="float:left">
<img src="./tab/images/tb.gif" width="16px" height="16px" style="vertical-align:middle"/>
<span style="font-weight:bold">你当前的位置</span>:<a href='studentInfo.jsp'>[学生信息管理系统]</a>-<a href='query.jsp'>[学生查询]</a>-[按SQL语句查询]
</div>
<div style="float:right;padding-right:50px">
<img width='16' height='16' src="./tab/images/query.png" style="vertical-align:middle"/>
<a href="query.jsp"><strong>查找学生记录</strong></a>
</div>
<div style="float:right;padding-right:50px">
<img width='16' height='16' src="./tab/images/add.gif" style="vertical-align:middle"/>
<a href="Insert.jsp"><strong>新增学生记录</strong></a>
</div>
</div>
<div class="right_top"></div>
</div>
<!-- 导航栏部分结束 -->
<!-- 表格部分 -->
<div class="div_clear">
<div class="left_center"></div>
<!-- 表格内容块 -->
<div class="center_center">
<div class="table_content">
<table cellspacing="0px" cellpadding="0px">
<!-- 表头 -->
<thead>
<tr>
<th width="16%">学院名称</th>
<th width="16%">专 业</th>
<th width="16%">班 级</th>
<th width="16%">学 号</th>
<th width="16%">姓 名</th>
<th width="20%" style="border-right:none">操作</th>
</tr>
</thead>
<!-- 表体 -->
<tbody>
<%
int count=0;
if(!rs.next()){
out.print("<tr>");
out.print("<td colspan=6>");
out.print(" <div center='align' width='100%'><font size='5' color='red'><strong> 查询失败:未查询到该学生记录"+
"<br><a href='query.jsp'> <span id='jumpTo'>8</span>秒后系统会自动跳转,也可点击本处直接跳转重新查询</a> "+
"</strong><br> </font></div>");
String jsscript="<script type='text/javascript'> countDown(8,'query.jsp'); </script> ";
out.print(jsscript);
out.print("</td>");
out.print("</tr>");
}
else{
rs.beforeFirst();
//获取列数
ResultSetMetaData resultSetMetaData=rs.getMetaData();
int numColumn=resultSetMetaData.getColumnCount();
int i;
List<String> name=new ArrayList<String>();
String columnName;
//定义开关,true的位置说明是有数据的
boolean[] switchs=new boolean[]{false,false,false,false,false,false};
for(i=1;i<=numColumn;i++){
columnName=resultSetMetaData.getColumnName(i);
System.out.println(columnName);
switch(columnName){
case "academy":
switchs[0]=true;
break;
case "major":
switchs[1]=true;
break;
case "class":
switchs[2]=true;
break;
case "sno":
switchs[3]=true;
break;
case "name":
switchs[4]=true;
break;
case "id":
break;
default:
break;
}
}
//定义rs的列数
int rsColumn;
while(rs.next()){
//i确定位置,rsColumn确定数据在rs中的位置
i=1;
rsColumn=0;
out.print("<tr>");
for(;i<=6;i++){
if(switchs[i-1]){
if(rsColumn<=numColumn)
{
rsColumn++;
}
out.print("<td>" + rs.getString(rsColumn).toString()+ "</td>");
}
else{
if(i!=6){
out.print("<td>" +"null"+ "</td>");
}
else{
out.print("<td>" +"暂不支持操作"+ "</td>");
}
}
}
out.print("</tr>");
count++;
}
}
%>
<!-- 表尾 -->
<tfoot>
</tfoot>
</table>
</div>
</div>
<div class="right_center"></div>
</div>
<!-- 底部框 -->
<div class="div_clear">
<div class="left_bottom"></div>
<div class="center_bottom">
<span> 共有<%=count %>条记录,当前第 1/1 页</span>
<div style="float:right;padding-right:30px">
<input type="button" value="首页"/>
<input type="button" value="上页"/>
<input type="button" value="下页"/>
<input type="button" value="尾页"/>
<span>跳转到</span>
<input type="text" size="1"/>
<input type="button" value="跳转"/>
</div>
</div>
<div class="right_bottom"></div>
</div>
</div>
<%
if (rs != null) {
rs.close();
}
%>
</body>
</html>