(1)python DB API包含的内容:

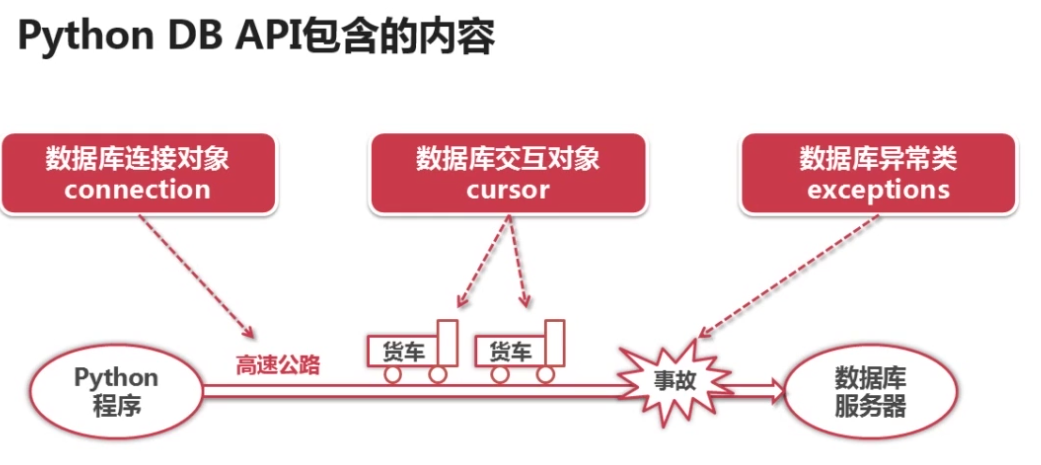

(2)使用python DB API访问数据库的流程:
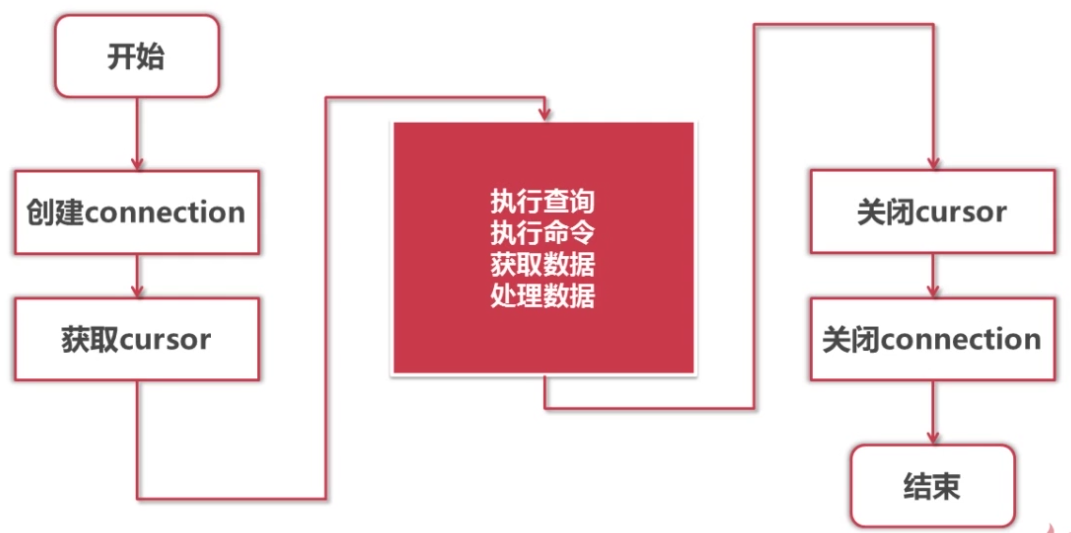

(3)python DB API的主要方法:
- connect连接类:
- conn = pymsql.connect(host="localhost", port=3306, user="root", passwd="root", db="goods", charset="utf8"):创建一个mysql连接。
- cursor = conn.cursor():创建游标,用于python和mysql之间通信,即执行sql语句。
- conn.rollback(): 回滚,使得cursor指代的sql语句执行失败后,数据库保持执行前的状态不变。
- conn.commit():确认,使得sql语句执行后,数据库产生相应变化。
- conn.close():关闭连接。
- cursor游标类:
- cursor.execute(sql):实参为sql语句的字符串,用于在MySQL中执行sql语句。
- cursor.fetchone():返回查询结果的下一行,形式为元组,即:((1 line),(2 line),(3 line) )。
- cursor.fetchsize(size):返回查询结果的下size行,形式为元组。
- cursor.fetchall():返回查询结果的剩余行,形式为元组。
- cursor.rowcount:最近一次execute中影响数据库的行数。
- cursor.close():关闭游标。
(4)python3.6操作数据库---简单增删改查:
import pymysql if __name__ == '__main__': #创建MYSQL连接 conn = pymysql.Connect(host = '127.0.0.1',port = 3306,user = 'root',passwd = 'root',db = 'databasetest',charset = 'utf8') #创建游标:用于 Python 和 Mysql 之间的通信,即:用于执行 SQL 语句。 cursor = conn.cursor() try: #增加数据库 sql = "INSERT INTO userdata VALUES (1,'lbg'),(2,'zgj'),(3,'ldt')" cursor.execute(sql) #删除数据库 sql2 = "DELETE FROM userdata WHERE id=3" cursor.execute(sql2) #更新数据库 sql3 = "UPDATE userdata SET username='wjz' WHERE id=2" cursor.execute(sql3) #查询数据库 sql4 = "SELECT * FROM userdata" num = cursor.execute(sql4) print("the number of the userdata: %d" % num) #fetchall返回一个元组,其中包含所有查询的结果。 for row in cursor.fetchall(): print(" ID: "+str(row[0])+" name: "+row[1]) except Exception as reason: #SQL事物回滚:将事务中对数据库的所有已经完成的操作全部撤销,回滚到事务开始时的状态 conn.rollback() print('事务处理失败', reason) else: #SQL事物确认:只有事物确认之后,数据库操作才会生效 conn.commit() print('事务处理成功',cursor.rowcount) finally: #关闭连接和游标 cursor.close() conn.close()
(5)python3.6操作数据库---银行转账:
import pymysql import sys class TransferMoney: def __init__(self,conn): self.conn = conn def transfer(self,id_out,id_in,money): try: self.HasIdAccount(id_out) self.HasIdAccount(id_in) self.OutIdHasEnoughMoney(id_out,money) self.SubMoney(id_out,money) self.AddMoney(id_in,money) conn.commit() except Exception as e: self.conn.rollback() raise e def HasIdAccount(self,userid): cursor = self.conn.cursor() try: sql = "select * from goods_change where id = %s" % userid cursor.execute(sql) res = cursor.fetchall() if len(res) != 1: raise Exception("id %s is not exist" % userid) print("Has id " + str(userid)) finally: cursor.close() def OutIdHasEnoughMoney(self,userid,change_money): cursor = self.conn.cursor() try: sql = "select money from goods_change where id = %s" % userid cursor.execute(sql) if int(cursor.fetchone()[0]) < int(change_money): raise Exception("id %s has not enough money" % userid) print("id " + str(userid) + " has enough money") finally: cursor.close() def SubMoney(self,userid,change_money): cursor = self.conn.cursor() try: sql = "update goods_change set money=money-%s where id=%s" % (change_money,userid) cursor.execute(sql) if cursor.rowcount != 1: raise Exception("id %s is not exist" % userid) print("id " + str(userid) + " sub " + str(change_money) + " RMB") finally: cursor.close() def AddMoney(self,userid,change_money): cursor = self.conn.cursor() try: sql = "update goods_change set money=money+%s where id=%s" % (change_money,userid) cursor.execute(sql) if cursor.rowcount != 1: raise Exception("id %s is not exist" % userid) print("id " + str(userid) + " add " + str(change_money) + " RMB") finally: cursor.close() if __name__ == '__main__': conn = pymysql.Connect(host='127.0.0.1',port=3306,user='root',passwd='root',db='goods',charset='utf8') id_out = sys.argv[1] id_in = sys.argv[2] money = sys.argv[3] trans = TransferMoney(conn) try: trans.transfer(id_out,id_in,money) except Exception as reason: print('事务处理失败:', reason) finally: conn.close()