#include "stdlib.h"
#include "math.h"
#include "stdio.h"
#include "conio.h"
#include "windows.h"
#include "time.h"
#include "graphics.h"
#define Width 640
#define Hight 480
void gotoxy(int x,int y) //光标移动到(x,y)位置
{
HANDLE handle = GetStdHandle(STD_OUTPUT_HANDLE);
COORD pos;
pos.X = x;
pos.Y = y;
SetConsoleCursorPosition(handle,pos);
}
//定义食物
struct Food {
int Foodx;
int Foody;
}Food;
//点
typedef struct Point
{
int x;
int y;
}Point;
//小蛇最长身长
#define bodyLen 100
typedef struct Snake{
Point body[bodyLen];
int tail;
int head;
}Snake;
Snake snake;
int newx,newy;
//初始化
void init()
{
snake.tail=2;
snake.head=6;
for (int i=7;i<=11;i++)
{
snake.body[i-5].x=i;
snake.body[i-5].y=12;
}
newx=11;
newy=12;
initgraph(Width,Hight);
srand((unsigned)time(NULL));
Food.Foodx=rand()%64;
Food.Foody=rand()%48;
}
//判断是否空
bool IfEmpty(Snake *q)
{
if(snake.head==snake.tail)
return true;
else
return false;
}
//判断是否满
bool IfFull(Snake q)
{
if(snake.tail==(snake.head+1)%bodyLen)
return true;
else
return false;
}
//定义方向键
int direction;//aleft,dright,wup,sdown
void UpdateWithInput()
{
while(kbhit())
{
char ch=getch();
switch(ch)
{
case 'a':
case 'A':
direction=0;//left
break;
case 'd':
case 'D':
direction=1;//right
break;
case 'w':
case 'W':
direction=2;
break;
case 's':
case 'S':
direction=3;
break;
}
}
}
//入队列
void InsertQuery(Snake &snake,Point point)
{
if(!IfFull(snake))
{
snake.head=(snake.head+1)%bodyLen;
int ipos=snake.head;
snake.body[ipos].x=point.x;
snake.body[ipos].y=point.y;
}
else
{
printf("达到上限,游戏结束");
exit(0);
}
}
//出队列
void DeleteQuery(Snake &snake)
{
snake.tail=(snake.tail+1)%bodyLen;
}
//碰撞检测
void UpdateWithoutInput()
{
if (direction==0)
{
newx--;
if (newx<=3)
{
direction=3;
}
}
if (direction==1)
{
newx++;
if (newx*10>Width-30)
{
direction=2;
}
}
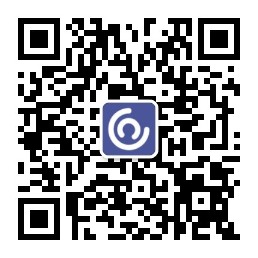
if (direction==2)
{
newy--;
if (newy<=3)
{
direction=0;
}
}
if (direction==3)
{
newy++;
if (newy*10>Hight-30)
{
direction=1;
}
}
double d=sqrt((10*(newx-Food.Foodx))*(10*(newx-Food.Foodx))+(10*(newy-Food.Foody))*(10*(newy-Food.Foody)));
if(d<10)
{
Point p;
p.x=Food.Foodx;
p.y=Food.Foody;
InsertQuery(snake,p);
Food.Foodx=rand()%(Width/10);
Food.Foody=rand()%(Hight/10);
}
}
//
void show()
{
int tail,head;
tail=snake.tail;
head=snake.head;
//system("cls");
setcolor(BLUE);
setfillcolor(WHITE);
fillrectangle(0,0,Width,Hight);
for (int position=tail;position<head;position++)
{
fillcircle(snake.body[position].x*10,snake.body[position].y*10,5);
}
setcolor(YELLOW);
setfillcolor(RED);
fillcircle(snake.body[head].x*10,snake.body[head].y*10,5);
setfillcolor(GREEN);
fillcircle(Food.Foodx*10,Food.Foody*10,5);
Point point;
point.x=newx;
point.y=newy;
InsertQuery(snake,point);
DeleteQuery(snake);
Sleep(300);
}
void main()
{
init();
while (1)
{
show();
UpdateWithInput();
UpdateWithoutInput();
}
//system("pause");
}