目录:
Java NIO 学习笔记(一)----概述,Channel/Buffer
Java NIO 学习笔记(二)----聚集和分散,通道到通道
Java NIO 学习笔记(三)----Selector
Java NIO 学习笔记(四)----文件通道和网络通道
Java NIO 学习笔记(五)----路径、文件和管道 Path/Files/Pipe
Java NIO 学习笔记(六)----异步文件通道 AsynchronousFileChannel
AsynchronousFileChannel 异步文件通道
在 Java 7 中,AsynchronousFileChannel 已添加到 Java NIO 中,它可以异步读取数据并将数据写入文件。先说明,异步和阻塞/非阻塞没有关系,下面简单介绍一下相关概念:
- 阻塞是进程发起任务请求然后一直等到任务完成,再把任务结果返回。
- 非阻塞是发起任务请求之后就马上返回去做别的事,然后再时不时主动查看任务请求是否被完成。(轮询)
- 同步是如果完成任务时,遇到一个耗时操作,需要等操作返回,才能继续执行剩下的操作。
- 异步是如果完成任务时,遇到一个耗时操作,不等完成,而是去做其他事情,也不主动查看是否完成,而是由耗时操作完成的通知再回来继续执行,常见的例子就是 Ajax 。
一般异步都是和非阻塞组合使用的。
创建 AsynchronousFileChannel
通过其静态方法 open() 创建 AsynchronousFileChannel ,下面是一个示例:
Path path = Paths.get("D:\\test\\input.txt");
AsynchronousFileChannel fileChannel = AsynchronousFileChannel.open(path, StandardOpenOption.READ);
// 作为对比,普通的 FileChannel 是这样打开的:
RandomAccessFile aFile = new RandomAccessFile("D:\\test\\input.txt", "rw");
FileChannel inChannel = aFile.getChannel();
open() 方法的第一个参数是指向 AsynchronousFileChannel 要关联的文件的 Path 实例。第二个参数是可选的,可以是一个或多个打开选项,这些选项告诉 AsynchronousFileChannel 在底层文件上执行什么操作。在这个例子中,我们使用了 StandardOpenOption.READ,这意味着该文件将被打开以供阅读。
读取数据
可以通过两种方式从 AsynchronousFileChannel 读取数据,都是调用 read() 方法之一完成的。
Future<Integer> read(ByteBuffer dst, long position);
<A> void read(ByteBuffer dst,
long position,
A attachment,
CompletionHandler<Integer,? super A> handler);
通过 Future 读取数据
Future 是 Java 多线程方面的内容,后面我会再继续学习多线程的知识,这里先稍微了解,带过。
首先这个 Futrue 类有什么用?我们知道多线程编程的时候,一般使用 Runable ,重写 run() 方法,然后调用线程对象的 start() 方法,重点就在 run() 方法的返回值是 void ,那如果我们需要线程执行完成后返回结果怎么办,Future 就可以解决这个问题。
从 AsynchronousFileChannel 读取数据的第一种方法是调用 read() 方法,该方法返回 Future :
Future<Integer> operation = fileChannel.read(buffer, 0);
此版本的 read() 方法将 ByteBuffer 作为第一个参数。 从 AsynchronousFileChannel 读取的数据被读入此 ByteBuffer ,第二个参数指定文件中要开始读取的字节位置。
即使读取操作尚未完成,read() 方法也会立即返回,可以通过调用 read() 方法返回的 Future 实例的 isDone() 方法来检查读取操作何时完成。这是一个示例:
Path path = Paths.get("D:\\test\\input.txt");
AsynchronousFileChannel channel = AsynchronousFileChannel.open(path, StandardOpenOption.READ);
ByteBuffer buffer = ByteBuffer.allocate(1024);
long position = 0;
Future<Integer> operation = channel.read(buffer, position);
while (!operation.isDone()) ;
buffer.flip();
System.out.println(new String(buffer.array()));
此示例创建一个 AsynchronousFileChannel ,然后创建一个 ByteBuffer ,它作为参数传递给 read() 方法,并且位置为 0 ,在调用 read() 之后,循环调用 Future 的 isDone() 方法直到返回 true。 当然,这不是一个非常有效的 CPU 使用,但是这里需要等待读取操作完成。读取操作完成后,将数据读入 ByteBuffer 并输出。
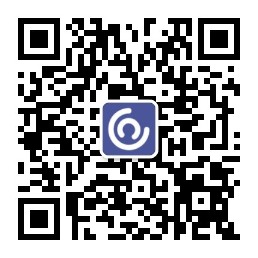
通过 CompletionHandler 读取数据
从 AsynchronousFileChannel 读取数据的第二种方法是调用以 CompletionHandler 作为参数的 read() 方法版本,其第二个参数 position 可指定从什么位置开始读取。 以下是调用此 read() 方法的示例:
Path path = Paths.get("D:\\test\\input.txt");
AsynchronousFileChannel channel = AsynchronousFileChannel.open(path);
ByteBuffer buffer = ByteBuffer.allocate(1024);
channel.read(buffer, 0, buffer, new CompletionHandler<Integer, ByteBuffer>() {
@Override
public void completed(Integer result, ByteBuffer attachment) {
System.out.println("result: " + result);
attachment.flip();
System.out.println(new String(attachment.array()));
attachment.clear();
}
@Override
public void failed(Throwable exc, ByteBuffer attachment) {
System.out.println("failed");
}
});
一旦读取操作完成,将调用 CompletionHandler 的 completed() 方法,completed() 方法的第一个参数是 Integer 类型,表示读取了多少字节,attachment 对应 read() 方法的第三个参数。 在这个例子中也是 ByteBuffer,数据也被读入其中。 可以自由选择要附加的对象。如果读取操作失败,则将调用 CompletionHandler 的 failed() 方法。
写入数据
和读取数据类似,也可以通过 AsynchronousFileChannel 对象的 2 种方法写入数据:
Future<Integer> write(ByteBuffer src, long position);
<A> void write(ByteBuffer src,
long position,
A attachment,
CompletionHandler<Integer,? super A> handler);
具体的操作请参考读取数据,这里不展开了。