版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/hsx1612727380/article/details/84679327
Title:Longest Continuous Increasing Subsequence 674
Difficulty:Easy
原题leetcode地址:https://leetcode.com/problems/longest-continuous-increasing-subsequence/
1. 后面的数大每次加加,否则为1,每次都比较下大小
时间复杂度:O(n),一次一层for循环,需要遍历整个数组。
空间复杂度:O(1),没有申请额外的空间。
/**
* 后面的数大每次加加,否则为1,每次都比较下大小
* @param nums
* @return
*/
public static int findLengthOfLCIS(int[] nums) {
if (nums == null || nums.length <= 0) {
return 0;
}
if (nums.length == 1) {
return 1;
}
int count = 1;
int max = Integer.MIN_VALUE;
for (int i = 0; i < nums.length - 1; i++) {
if (nums[i + 1] > nums[i]) {
count++;
}
else {
count = 1;
}
if (count > max) {
max = count;
}
}
return max;
}
2. 动态规划
时间复杂度:O(n),一次一层for循环,需要遍历整个数组。
空间复杂度:O(n),申请与nums长度一样的大的数组长度n。
/**
* 动态规划
* @param nums
* @return
*/
public static int findLengthOfLCIS1(int[] nums) {
if (nums == null || nums.length <= 0) {
return 0;
}
if (nums.length == 1) {
return 1;
}
int tmp[] = new int[nums.length];
tmp[0] = 1;
for (int i = 1; i < nums.length; i++) {
tmp[i] = 1;
if (nums[i] > nums[i - 1]) {
tmp[i] = tmp[i - 1] + 1;
}
}
int max = Integer.MIN_VALUE;
for (int i = 0; i < tmp.length; i++) {
if (tmp[i] > max) {
max = tmp[i];
}
}
return max;
}
扫描二维码关注公众号,回复:
4323268 查看本文章
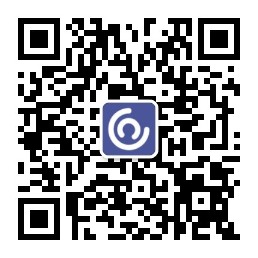