版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/u012142460/article/details/82384197
linux内核调度三种策略:
1,SCHED_OTHER 分时调度策略,
2,SCHED_FIFO实时调度策略,先到先服务
3,SCHED_RR实时调度策略,时间片轮转
分时进程则通过nice和counter值决定权值,nice越小,counter越大,被调度的概率越大,也就是曾经使用了cpu最少的进程将会得到优先调度。所以分时调度与优先级是无关的,在这种调度下,优先级是无法修改的,默认情况下创建的线程采用的分时调度策略。要修改线程优先级需要首先修改内核调度策略。
SCHED_FIFO和SCHED_RR属于实时调度策略,在RTOS中基本用的也都是这两种策略。SCHED_RR是时间片轮转,同一优先级的线程分配相同的时间片进行执行,时间片到了之后,将当前线程放到队尾,执行下一个线程。
SCHED_FIFO 情况下,一旦占有了CPU后,知道线程执行完或者主动释放CPU,才会结束对CPU的占有。
函数的使用
获取线程可支持的最高和最低优先级,SCHED_FIFO和SCHED_RR是1-99,数值越大优先级越高。SCHED_OTHER不支持优先级。
int sched_get_priority_max(int policy);
int sched_get_priority_min(int policy);
系统创建线程时,默认的线程是SCHED_OTHER。所以如果我们要改变线程的调度策略的话,可以通过下面的这个函数实现。
扫描二维码关注公众号,回复:
4317692 查看本文章
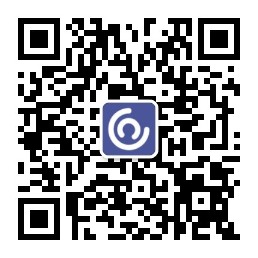
int pthread_attr_setschedpolicy(pthread_attr_t *attr, int policy);
policy参数就是我们的调度策略。
获取和设置优先级,一般是获取当前优先级,再去设置一下优先级。
int pthread_attr_setschedparam(pthread_attr_t *attr, const struct sched_param *param);
int pthread_attr_getschedparam(const pthread_attr_t *attr, struct sched_param *param);
sched_param结构体如下,只有一个元素,就是优先级
struct sched_param
{
int __sched_priority;
};
修改优先级代码
#include <stdio.h>
#include <pthread.h>
#include <sched.h>
#include <assert.h>
static void show_thread_priority(pthread_attr_t *attr,int policy)
{
int priority = sched_get_priority_max(policy);
assert(priority!=-1);
printf("max_priority=%d\n",priority);
priority= sched_get_priority_min(policy);
assert(priority!=-1);
printf("min_priority=%d\n",priority);
}
static int get_thread_policy(pthread_attr_t *attr)
{
int policy;
int rs = pthread_attr_getschedpolicy(attr,&policy);
//assert(rs==0);
switch(policy)
{
case SCHED_FIFO:
printf("policy= SCHED_FIFO\n");
break;
case SCHED_RR:
printf("policy= SCHED_RR");
break;
case SCHED_OTHER:
printf("policy=SCHED_OTHER\n");
break;
default:
printf("policy=UNKNOWN\n");
break;
}
return policy;
}
static void set_thread_policy(pthread_attr_t *attr,int policy)
{
int rs = pthread_attr_setschedpolicy(attr,policy);
assert(rs==0);
get_thread_policy(attr);
}
int main()
{
pthread_t pid;
pthread_attr_t attr;
struct sched_param param1;
int new_pri = 20;
int i,ret;
pthread_attr_init(&attr);
int policy = get_thread_policy(&attr);
printf("show current configuration of priority\n");
show_thread_priority(&attr,policy);
printf("show SCHED_FIFO of priority\n");
show_thread_priority(&attr,SCHED_FIFO);
printf("show SCHED_FIFO of priority\n");
show_thread_priority(&attr,SCHED_RR);
set_thread_policy(&attr, SCHED_FIFO);
pthread_attr_getschedparam(&attr,¶m1);
printf("ori pri:%d\n",param1.sched_priority);
param1.sched_priority = new_pri;
pthread_attr_setschedparam(&attr,¶m1);
pthread_attr_getschedparam(&attr,¶m1);
printf("cur pri:%d\n",param1.sched_priority);
return 0 ;
}
执行结果