版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/yerenyuan_pku/article/details/84206834
高级for循环
高级for循环的作用是用于遍历Collection集合或数组。其格式为:
for(数据类型(一般是泛型类型) 变量名 : 被遍历的集合(Collection)或者数组) {
}
遍历Collection集合
之前我们使用迭代器是这样遍历的。
package cn.liayun.foreach;
import java.util.ArrayList;
import java.util.Iterator;
public class ForeachDemo {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<String>();
list.add("abc1");
list.add("abc2");
list.add("abc3");
for (Iterator<String> it = list.iterator(); it.hasNext();) {
String str = it.next();
System.out.println(str);
}
}
}
现在我们可使用高级For循环了,主要就是为了简化书写。
package cn.liayun.foreach;
import java.util.ArrayList;
import java.util.Iterator;
public class ForeachDemo {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<String>();
list.add("abc1");
list.add("abc2");
list.add("abc3");
/*
for (Iterator<String> it = list.iterator(); it.hasNext();) {
String str = it.next();
System.out.println(str);
}
*/
for (String s : list) {
System.out.println(s);
}
}
}
小结
高级for循环对集合进行遍历,只能获取集合中的元素,但是不能对集合进行操作。迭代器除了遍历,还可以进行remove()集合中元素的动作,如果使用ListIterator,还可以在遍历过程中对集合进行增删改查的操作。
遍历数组
对于数组,我们可使用传统for循环遍历。
package cn.liayun.foreach;
public class ForeachDemo {
public static void main(String[] args) {
int[] arr = {3, 5, 1};
for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
}
}
现在可使用高级for循环进行遍历了。
package cn.liayun.foreach;
public class ForeachDemo {
public static void main(String[] args) {
int[] arr = {3, 5, 1};
/*
for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
*/
for (int i : arr) {
System.out.println(i);
}
}
}
小结
传统for和高级for有什么区别呢? 高级for有一个局限性,必须有被遍历的目标,而该目标只能是Collection或数组。建议在遍历数组的时候还是希望使用传统for,因为传统for可以定义角标。
遍历Map集合
对于Map集合,我们也可以使用高级for循环进行遍历。
第一种遍历方式
package cn.liayun.foreach;
import java.util.HashMap;
public class ForeachDemo {
public static void main(String[] args) {
HashMap<Integer, String> hm = new HashMap<Integer, String>();
hm.put(1, "a");
hm.put(2, "b");
hm.put(3, "c");
for(Integer key : hm.keySet()) {
String value = hm.get(key);
System.out.println(key + "::" + value);
}
}
}
第二种遍历方式
package cn.liayun.foreach;
import java.util.HashMap;
import java.util.Map;
public class ForeachDemo {
public static void main(String[] args) {
HashMap<Integer, String> hm = new HashMap<Integer, String>();
hm.put(1, "a");
hm.put(2, "b");
hm.put(3, "c");
/*
for(Integer key : hm.keySet()) {
String value = hm.get(key);
System.out.println(key + "::" + value);
}
*/
for(Map.Entry<Integer, String> me : hm.entrySet()) {
Integer key = me.getKey();
String value = me.getValue();
System.out.println(key + "------" + value);
}
}
}
可变参数
可变参数其实就是一种数组参数的简写形式,不用每一次都手动的建立数组对象,只要将要操作的元素作为参数传递即可,隐式地将这些参数封装成了数组。
package cn.liayun.param;
public class ParamDemo {
public static void main(String[] args) {
int sum = add(3, 4, 10, 6, 7, 43, 8);
int sum2 = add(99, 11, 33, 22);
}
/*
* 可变参数需要注意,只能定义在参数列表的最后。
*/
public static int add(int... arr) {
int sum = 0;
for (int i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
}
注意:方法的可变参数在使用时,可变参数一定要定义在参数列表的最后面。
静态导入
package cn.liayun.staticimport;
import java.util.*;
import static java.util.Collections.*;//导入的是Collections类中的所有静态成员
import static java.lang.System.*;//导入了System类中所有静态成员
public class StaticImportDemo {
public static void main(String[] args) {
List<String> list = new ArrayList<String>();
list.add("a");
list.add("c");
sort(list);
// System.out.println(max(list));
out.println("hello");
}
}
注意:当类名重名时,需要指定具体的包名;当方法重名时,需要指定具备所属的对象或类。
扫描二维码关注公众号,回复:
4283216 查看本文章
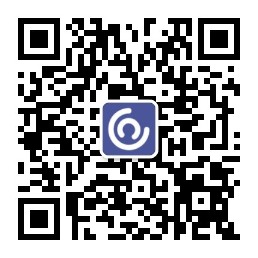