版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/xiaoguaihai/article/details/84277854
文章目录
1. 题目描述:
Given an array nums
of n integers and an integer target
, are there elements a, b, c, and d in nums
such that a + b + c + d = target
? Find all unique quadruplets in the array which gives the sum of target
.
Note:
The solution set must not contain duplicate quadruplets.
Example:
Given array nums = [1, 0, -1, 0, -2, 2], and target = 0.
A solution set is:
[
[-1, 0, 0, 1],
[-2, -1, 1, 2],
[-2, 0, 0, 2]
]
2. 思路分析:
题目的意思是找到数组中所有4个和为给定数(target)的数,并且不能重复。
该题可以先转化成Three Sum的思路,再转化成Two Sum的思路去解决。
先固定两个数,然后从数组中剩下的数中查找另外2个数,则转化成了Two Sum问题:先排序数组,使两个指针分别指向首尾的两个数,如果这4个数和等于target,则找到,如果小于target则右移左指针,如果大于target则左移右指针。
关键是题目要求去重,所以每次移动指针的时候要判断一下是否和上一个数相同,如果相同则继续移动。
扫描二维码关注公众号,回复:
4210288 查看本文章
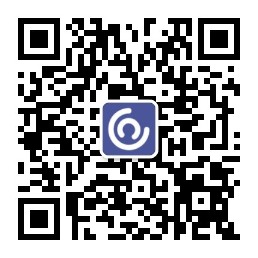
3. Java代码:
源代码
:见我GiHub主页
代码:
public static List<List<Integer>> fourSum(int[] nums, int target) {
List<List<Integer>> result = new ArrayList<>();
Arrays.sort(nums);
for (int i = 0; i < nums.length - 3; i++) {
// 用于去重
if (i != 0 && nums[i] == nums[i - 1]) {
continue;
}
for(int j = i + 1; j < nums.length - 2; j++) {
// 用于去重
if (j != i + 1 && nums[j] == nums[j - 1]) {
continue;
}
int left = j + 1;
int right = nums.length - 1;
while (left < right) {
int fourSum = nums[i] + nums[j] + nums[left] + nums[right];
if (fourSum == target) {
result.add(Arrays.asList(nums[i], nums[j], nums[left], nums[right]));
left++;
right--;
// 用于去重
while (left < right && nums[left] == nums[left - 1]) {
left++;
}
while (left < right && nums[right] == nums[right + 1]) {
right--;
}
} else if (fourSum < target) {
left++;
} else {
right--;
}
}
}
}
return result;
}