版权声明: https://blog.csdn.net/qq_24313635/article/details/84285503
配置中心之实践
我们再前几篇的基础上,继续学习SpringCloud框架,搭建ConfigServer和ConfigClient两个项目,项目结构
①ConfigServer项目
pom.xml
<?xml version="1.0"?>
<project
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"
xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>com.yj</groupId>
<artifactId>SpringCloud</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<artifactId>ConfigServer</artifactId>
<name>ConfigServer</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-config-server</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-eureka</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-bus-amqp</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
</dependencies>
<build>
<finalName>${artifactId}</finalName>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<skip>true</skip>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<configuration>
<archive>
<manifest>
<mainClass>com.yj.config.ConfigServerApplication</mainClass>
<addClasspath>true</addClasspath>
<classpathPrefix>lib</classpathPrefix>
</manifest>
<manifestEntries>
<Class-Path>./</Class-Path>
</manifestEntries>
</archive>
<excludes>
<exclude>config/**</exclude>
<exclude>**.xml</exclude>
<exclude>**.properties</exclude>
</excludes>
</configuration>
</plugin>
<plugin>
<artifactId>maven-assembly-plugin</artifactId>
<configuration>
<appendAssemblyId>false</appendAssemblyId>
<descriptors>
<descriptor>src/main/build/package.xml</descriptor>
</descriptors>
</configuration>
<executions>
<execution>
<id>make-assembly</id>
<phase>package</phase>
<goals>
<goal>single</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
<testResources>
<testResource>
<directory>src/main/resources</directory>
</testResource>
</testResources>
</build>
</project>
ConfigServerApplication
package com.yj.config;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.config.server.EnableConfigServer;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
@SpringBootApplication
@EnableConfigServer
@EnableEurekaClient
public class ConfigServerApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigServerApplication.class, args);
}
}
application.properties
spring.application.name=config-server
eureka.client.serviceUrl.defaultZone=http://admin:123456@peer1:8001/eureka,http://admin:123456@peer2:8002/eureka
eureka.instance.prefer-ip-address=true
eureka.instance.instance-id=${spring.cloud.client.ipAddress}:${server.port}
eureka.instance.hostname=${spring.cloud.client.ipAddress}
#配置文件在本地
spring.profiles.active=native
#配置文件的目录
#spring.cloud.config.server.native.search-locations=file:D:/config-repo
spring.cloud.config.server.native.search-locations=file:///opt/springcloud/config-repo
#security.basic.enabled=false
security.user.name=admin
security.user.password=123456
encrypt.key=myEncryptKey
#management.security.enabled=false
spring.rabbitmq.host=192.168.37.140
spring.rabbitmq.port=5672
spring.rabbitmq.username=guest
spring.rabbitmq.password=guest
#encrypt.key-store.location=classpath:/config-server.jks
#encrypt.key-store.alias=mytestkey
#encrypt.key-store.password=yangjie
#encrypt.key-store.secret=yangjie
startAll.sh
#! /bin/bash
moduleName="ConfigServer"
java -jar ./$moduleName.jar --server.port=8010 &
java -jar ./$moduleName.jar --server.port=8011 &
②ConfigClient项目
pom.xml
<?xml version="1.0"?>
<project
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"
xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>com.yj</groupId>
<artifactId>SpringCloud</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<artifactId>ConfigClient</artifactId>
<name>ConfigClient</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-eureka</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-bus-amqp</artifactId>
</dependency>
</dependencies>
<build>
<finalName>${artifactId}</finalName>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<skip>true</skip>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<configuration>
<archive>
<manifest>
<mainClass>com.yj.config.ConfigClientApplication</mainClass>
<addClasspath>true</addClasspath>
<classpathPrefix>lib</classpathPrefix>
</manifest>
<manifestEntries>
<Class-Path>./</Class-Path>
</manifestEntries>
</archive>
<excludes>
<exclude>config/**</exclude>
<exclude>**.xml</exclude>
<exclude>**.properties</exclude>
</excludes>
</configuration>
</plugin>
<plugin>
<artifactId>maven-assembly-plugin</artifactId>
<configuration>
<appendAssemblyId>false</appendAssemblyId>
<descriptors>
<descriptor>src/main/build/package.xml</descriptor>
</descriptors>
</configuration>
<executions>
<execution>
<id>make-assembly</id>
<phase>package</phase>
<goals>
<goal>single</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
<testResources>
<testResource>
<directory>src/main/resources</directory>
</testResource>
</testResources>
</build>
</project>
ConfigClientController
package com.yj.config.controller;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.cloud.context.config.annotation.RefreshScope;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RefreshScope
public class ConfigClientController {
@Value("${config.testStr}")
private String testStr;
@RequestMapping("/hiConfig")
public String hiConfig(){
return testStr;
}
}
ConfigClientApplication
扫描二维码关注公众号,回复:
4155483 查看本文章
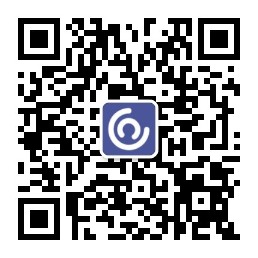
package com.yj.config;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
@SpringBootApplication
@EnableEurekaClient
public class ConfigClientApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigClientApplication.class, args);
}
}
bootstrap.properties
server.port=8012
spring.application.name=config-client
eureka.client.serviceUrl.defaultZone=http://admin:123456@peer1:8001/eureka,http://admin:123456@peer2:8002/eureka
eureka.instance.prefer-ip-address=true
eureka.instance.instance-id=${spring.cloud.client.ipAddress}:${server.port}
eureka.instance.hostname=${spring.cloud.client.ipAddress}
spring.cloud.config.discovery.enabled=true
spring.cloud.config.discovery.service-id=config-server
spring.cloud.config.label=config-client
spring.cloud.config.profile=dev
spring.cloud.config.username=admin
spring.cloud.config.password=123456
spring.rabbitmq.host=192.168.37.140
spring.rabbitmq.port=5672
spring.rabbitmq.username=guest
spring.rabbitmq.password=guest
部署,在服务器/opt/springcloud/config-repo/config-client路径下放一个config-client-dev.properties的配置文件
config.testStr={cipher}9fdd3c1aee0d95551f7829ba9d7aa02dc096930b33e178c14dacf3502c9d79b2
加密串来源于http://admin:[email protected]:8010/encrypt/ 端点的加密结果。
启动端口为8010,8011的server实例,8012,8013的client实例
部署成功,
①请求http://192.168.37.140:8012/hiConfig,显示hiConfig
②修改配置文件加密串240552d25edcbac3f43f4a0262cc718e5a862cb54db548956f2dbf1e40be8415,然后请求
http://admin:[email protected]:8010/bus/refresh刷新接口,等待刷新成功后,重复请求步骤1,显示hiYj