面试题一:
package com.yan.interview;
import java.util.Arrays;
public class Exam4 {
public static void main(String[] args) {
int i = 1;
String str = "hello";
Integer num = 200;
int[] arr = {1,2,3,4,5};
MyData my = new MyData();
change(i,str,num,arr,my);
System.out.println("i = " + i);
System.out.println("str = " + str);
System.out.println("num = " + num);
System.out.println("arr = " + Arrays.toString(arr));
System.out.println("my.a = " + my.a);
}
public static void change(int j, String s, Integer n, int[] a,MyData m){
j += 1;
s += "world";
n += 1;
a[0] += 1;
m.a += 1;
}
}
class MyData{
int a = 10;
}
运行结果:
i = 1
str = hello
num = 200
arr = [2, 2, 3, 4, 5]
my.a = 11
方法的参数传递机制:
- 形参是基本数据类型 传递数据值
- 实参是引用数据类型 传递地址值
- 特殊的类型:String、包装类等对象不可变性
面试题二:
package com.yan.interview;
public class Exam5 {
static int s;//成员变量,类变量
int i;//成员变量,实例变量
int j;//成员变量,实例变量
{
int i = 1;//非静态代码块中的局部变量 i
i++;
j++;
s++;
}
public void test(int j){//形参,局部变量,j
j++;
i++;
s++;
}
public static void main(String[] args) {//形参,局部变量,args
Exam5 obj1 = new Exam5();//局部变量,obj1
Exam5 obj2 = new Exam5();//局部变量,obj1
obj1.test(10);
obj1.test(20);
obj2.test(30);
System.out.println(obj1.i + "," + obj1.j + "," + obj1.s);
System.out.println(obj2.i + "," + obj2.j + "," + obj2.s);
}
}
运行结果
2,1,5
1,1,5
考点
- 就近原则
- 变量的分类
- 成员变量:类变量、实例变量
- 局部变量
- 非静态代码块的执行:每次创建实例对象都会执行
- 方法的调用规则:调用一次执行一次
当局部变量与xx变量重名时,如何区分:
- 局部变量与实例变量重名: 在实例变量前面加“this.”
- 局部变量与类变量重名: 在类变量前面加“类名.”
扫描二维码关注公众号,回复:
4154272 查看本文章
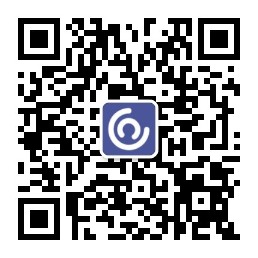