一、HTML DOM
1.Document对象
1.1属性
title
描述:获取/设置标题栏信息
语法: document.title = value 或 var 变量名称 = document.title
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<script>
function changeTitle(){
document.title = '网页标题';
}
</script>
</head>
<body>
<input type="button" value="单击我,设置标题栏信息" onclick="changeTitle()">
</body>
</html>
head
描述:获取文档的head对象
语法:Element document.head
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>异步加载JS文档</title>
<script>
function loadJS(){
var scriptEle = document.createElement('script');
scriptEle.src = 'scripts/common.js';
document.head.appendChild(scriptEle);
}
</script>
</head>
<body>
<input type="button" value="单击我,加载common.js文件" onclick="loadJS()">
</body>
</html>
body
描述:获取文档的body对象
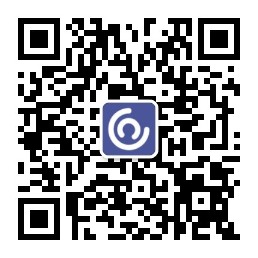
语法:Element document.body
images
描述:返回文档中所有的图像组成的集合(数组)
语法:NodeList document.images
forms
描述:返回文档中所有的表单组成的集合(数组)
语法:NodeList document.forms
links
描述:返回文档中所有的标记组成的集合(数组)
语法:NodeList document.links
anchors
描述:返回文档中所有的标记组成的集合(数组)
语法:NodeList document.anchors
1.2方法
querySelectorAll()
描述:返回由使用指定的CSS选择器的对象组成的集合(数组)
语法:NodeList document.querySelectorAll(selector)
说明:该方法支持所有CSS3选择器
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>querySelectorAll()方法</title>
<script>
function getObjectNum(){
//返回文档中所有使用t1 CSS类的对象所形成的数组
var eles = document.querySelectorAll('.t1');
window.alert(eles.length);
}
</script>
</head>
<body>
<p class="t1">段落</p>
<div class="t1">DIV</div>
<h2 class="t1">标题</h2>
<h3 class="t1">标题</h3>
<div class="t1">DIV</div>
<input type="button" value="获取使用.t1类的对象的数量" onclick="getObjectNum()">
</body>
</html>
querySelector()
描述:返回由使用指定的CSS选择器的对象的第一个元素
语法:Element document.querySelector(selector)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>querySelectorAll()方法</title>
<script>
function changeContent(){
var pEle = document.querySelector('.t1');
pEle.innerHTML = '中华人民共和国';
}
</script>
</head>
<body>
<p class="t1">段落</p>
<div class="t1">DIV</div>
<h2 class="t1">标题</h2>
<h3 class="t1">标题</h3>
<div class="t1">DIV</div>
<input type="button" value="设置使用.t1类的第一对象的内容" onclick="changeContent()">
</body>
</html>
2.Element对象
2.1属性
tagName
描述:获取元素的标记名称
语法:string Element.tagName
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<script>
function getTagName(){
var ele = document.getElementById('p');
window.alert(ele.tagName);
}
</script>
</head>
<body>
<div id="p">中华人民共和国</div>
<input type="button" value="单击我,获取id为p的元素的名称" onclick="getTagName()">
</body>
</html>
2.2方法
querySelector()
描述:返回由使用指定的CSS选择器的对象的第一个元素
语法:Element Element.querySelector(selector)
querySelectorAll()
描述:返回由使用指定的CSS选择器的对象组成的集合(数组)
语法:NodeList Element.querySelectorAll(selector)
二、BOM
1.BOM(Browser Object Model),浏览器对象模型,提供与浏览器相关的API.
window对象是BOM的顶级对象,代表浏览器窗口或iframe或frame.
2.属性
document
描述:返回HTMLDocument对象
history
描述:返回History对象
screen
描述:返回Screen对象
navigator
描述:返回Navigator对象
location
描述:返回Location对象
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>location对象</title>
<script>
function redirect(){
var selEle = document.getElementById('friendLink');
var url = selEle.value;
//设置浏览器地址栏中的地址为获取到的信息
location.href = url;
}
</script>
</head>
<body>
友情链接:
<select id="friendLink" onchange="redirect()">
<option value="">请选择</option>
<option value="http://www.sina.com.cn">新浪</option>
<option value="http://www.163.com">网易</option>
<option value="http://www.taobao.com">淘宝</option>
</select>
</body>
</html>
Math
描述:返回Math对象
3.方法
alert()方法
描述:弹出警示对话框(只有一个确定按钮)
语法:window.alert(string)
confirm()方法
描述:弹出询问对话框(有确定和取消两个按钮)
语法:bool window.confirm(string)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<script>
function removeItem(){
if(window.confirm('确认要删除吗?\n删除后将无法恢复')) {
window.alert('新闻删除成功');
}
}
</script>
</head>
<body>
<table width="100%" cellpadding="10" cellspacing="0" border="1">
<tr>
<td>关于HTML的问题</td>
<td>张三</td>
<td><a href="#" onclick="removeItem()">删除</a></td>
</tr>
<tr>
<td>关于CSS的问题</td>
<td>李四</td>
<td>删除</td>
</tr>
<tr>
<td>关于JavaScript的问题</td>
<td>王五</td>
<td>删除</td>
</tr>
</table>
</body>
</html>
setInterval()
描述:每间隔指定的时间执行相关的代码(重复执行)
语法:int window.setInterval(function,milliseconds)
clearInterval()
描述:清除由setInterval()设置的timeId
语法:window.clearInterval(int timeId)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<h1 align="center">用户注册许可协议</h1>
<p align="center">...</p>
<p align="center">...</p>
<p align="center">...</p>
<p align="center">
<input type="button" value="我同意(5)" disabled id="agree" onclick="nextStep()">
</p>
</body>
<script>
function nextStep(){
location.href = 'http://www.baidu.com';
}
var i = 4;
var timeId = window.setInterval(function(){
document.getElementById('agree').value = '我同意(' + i + ')';
if(i==0){
window.clearInterval(timeId);
document.getElementById('agree').value = '我同意';
document.getElementById('agree').disabled = false;
}
i--;
},1000);
</script>
</html>
setTimeout()
描述:间隔指定的时间后执行相关的代码(执行一次)
语法:int window.setTimeout(function,milliseconds)
clearTimeout()
描述:清除由setTimeout()设置的timeId
语法:window.clearTimeout(int timeId)
parseInt()
描述:转换成整数
语法:int parseInt(value)
parseFloat()
描述:转换成浮点数
语法:int parseFloat(value)
isNaN
描述:检测值是否为NaN(Not a Number)
语法:bool isNaN(value)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<script>
console.log(parseInt(3.7999)); //3
console.log(parseInt('379'));//合法的数字字符串开头
console.log(parseInt('3e7'));//合法的数字字符串开头
console.log(parseInt('e37'));//非法的数字字符串开头
console.log(parseFloat(3.7999)); //3
console.log(parseFloat('379'));//合法的数字字符串开头
console.log(parseFloat('3e7'));//合法的数字字符串开头
console.log(parseFloat('e37'));//非法的数字字符串开头
console.log(isNaN(5));
console.log(isNaN(NaN));
</script>
</head>
<body>
</body>
</html>
4.Location对象
href属性
描述:获取/设置地址栏中的地址信息
语法:
location.href = string
var 变量名称 = location.href
search属性
描述:获取地址栏"?"以后所有的参数
语法:string location.search
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Location</title>
<script>
function changeUrl(){
//名称=值&名称=值
location.href = '09_search.html?id=1&action=remove';
}
function getParameter(){
var str = location.search;
window.alert(str);
}
</script>
</head>
<body>
<p><input type="button" value="(1).单击我,改变地址栏的表现" onclick="changeUrl()"></p>
<p><input type="button" value="(2).获取地址栏的参数" onclick="getParameter()"></p>
</body>
</html>
reload()
描述:重新加载文档
语法:location.reload(void)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Location</title>
</head>
<body>
<h1 id="random"></h1>
<input type="button" value="单击我,刷新页面" onclick="refresh()">
</body>
<script>
document.getElementById('random').innerHTML = Math.random();
function refresh(){
//刷新页面
location.reload();
}
</script>
</html>
5.Screen对象
width属性
描述:获取显示分辨率宽度
语法:number screen.width
height
描述:获取显示分辨率高度
语法:number screen.height
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<script>
window.alert('分辨率:' + screen.width + 'X' + screen.height);
</script>
</head>
<body>
</body>
</html>
6.History对象
back()
描述:后退一步
语法:history.back(void)
forward()
描述:前进一步
语法:history.forward(void)
go()
描述:前进/后退
语法:history.go(number)
说明:如果为负数,则后退;否则前进;
history.back() 等价于 history.go(-1)
history.forward() 等价于 history.go(1)
7.Navigator对象
userAgent属性(简称UA)
描述:返回代理器信息
语法:string navigator.userAgent
三、JSON
1.JSON基础
JSON(JavaScript Object Notation),是一种轻量级的数据交换格式;
JSON的官网 http://www.json.org
2.JSON支持的数据格式
数组:[value,…]
对象:{property:value,…}