版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/qq78442761/article/details/84096889
关联容器map
key + value 的值
关联容器 = 有序容器(红黑树) + 无序容器(散列表) + hash_map
有序容器中:
map的键值是不允许重复的
multimap的键值是允许重复的
set是一个集合,键值=实值,就是只包含一个值,既是键值也是实值,不允许重复
multiset运行重复
map
底层就是红黑树(平衡排序二叉树)
键值保存,高效访问
插入效率低于链表,因为涉及排序
下面是一段插入代码,菜鸡一般是这么写的:
#include <map>
#include <iostream>
using namespace std;
void MapConstruct(){
map<int, char> mp;
mp.insert(pair<int, char>(1, 'a'));
return;
}
int main(){
MapConstruct();
getchar();
return 0;
}
局部变量结构如下:
‘
进价一点点的写法如下代码(稍微有工作经验的人一般是这么写的)
#include <map>
#include <iostream>
using namespace std;
void MapConstruct(){
typedef pair<int, char> in_pair;
map<int, char> mp;
mp.insert(in_pair(1, 'a'));
//mp.insert(pair<int, char>(1, 'a'));
return;
}
int main(){
MapConstruct();
getchar();
return 0;
}
局部变量结构如下:
扫描二维码关注公众号,回复:
4091132 查看本文章
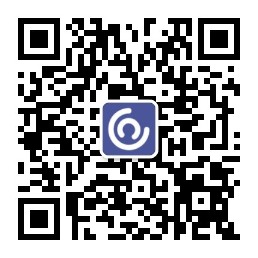
遍历一下:
#include <map>
#include <iostream>
#include <algorithm>
using namespace std;
typedef pair<int, char> in_pair;
void fun(in_pair pr){
cout << pr.first << "\t" << pr.second << endl;
}
void MapConstruct(){
map<int, char> mp;
mp.insert(in_pair(1, 'a'));
mp.insert(in_pair(2, 'b'));
mp.insert(in_pair(3, 'c'));
mp.insert(in_pair(4, 'd'));
for_each(mp.begin(), mp.end(), fun);
return;
}
int main(){
MapConstruct();
getchar();
return 0;
}
运行截图如下:
然后,大佬的写法(标准写法,一般是这种写法)
不得不说,大佬和小菜的最大区别就是大佬想得比较周到:
#include <map>
#include <iostream>
#include <algorithm>
using namespace std;
typedef pair<int, char> in_pair;
typedef pair<map<int, char>::iterator, bool> in_pair_bool;
void judgeOk(in_pair_bool pr){
if(pr.second){
cout << "插入成功!" << endl;
}
else{
cout << "插入失败!" << endl;
}
}
void fun(in_pair pr){
cout << pr.first << "\t" << pr.second << endl;
}
void MapConstruct(){
map<int, char> mp;
pair<map<int, char>::iterator, bool> pr;
pr = mp.insert(in_pair(1, 'a'));
judgeOk(pr);
pr = mp.insert(in_pair(2, 'b'));
judgeOk(pr);
pr = mp.insert(in_pair(3, 'c'));
judgeOk(pr);
pr = mp.insert(in_pair(4, 'd'));
judgeOk(pr);
pr = mp.insert(in_pair(1, 'e'));
judgeOk(pr);
for_each(mp.begin(), mp.end(), fun);
return;
}
int main(){
MapConstruct();
getchar();
return 0;
}
运行截图如下: