API
网关可以用来统一处理用户的所有请求,会根据不同请求路径将请求路由到不同的微服务中去
集成Zuul
创建项目
以之前的micro-weather-eureka-client-zuul
为蓝本,创建msa-weather-eureka-client-zuul
项目
修改源码
修改application.properties
配置文件:
#应用名称
spring.application.name=msa-weather-eureka-client-zuul
#注册服务器的URL
eureka.client.service-url.defaultZone=http://localhost:8761/eureka/
#zuul本身的配置
#设置需要路由的URL,此处设置hi资源的路径
zuul.routes.city.path=/city/**
#访问city应用时将请求转发到某应用的应用名称
zuul.routes.city.service-id=msa-weather-city-eureka
#zuul本身的配置
#设置需要路由的URL,此处设置weather资源的路径
zuul.routes.data.path=/data/**
#访问city应用时将请求转发到某应用的应用名称
zuul.routes.data.service-id=msa-weather-data-eureka
天气预报微服务重构
创建项目
以之前的msa-weather-report-eureka-feign
为蓝本,创建msa-weather-report-eureka-feign-gateway
项目
在com.study.spring.cloud.weather.service
包下新建DataClient
接口:
package com.study.spring.cloud.weather.service;
import com.study.spring.cloud.weather.vo.City;
import com.study.spring.cloud.weather.vo.WeatherResponse;
import org.springframework.cloud.netflix.feign.FeignClient;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import java.util.List;
@FeignClient("msa-weather-eureka-client-zuul")
public interface DataClient {
//获取城市列表
@GetMapping("/city/cities")
List<City> listCity() throws Exception;
//根据城市id查询天气数据
@GetMapping("/data/weather/cityId/{cityId}")
WeatherResponse getDataByCityId(@PathVariable("cityId") String cityId);
}
删除com.study.spring.cloud.weather.service
包下的CityClient
和WeatherDataClient
两个接口
修改com.study.spring.cloud.weather.controller
包下的WeatherReportController
类:
package com.study.spring.cloud.weather.controller;
import com.study.spring.cloud.weather.service.DataClient;
import com.study.spring.cloud.weather.service.WeatherReportService;
import com.study.spring.cloud.weather.vo.City;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.servlet.ModelAndView;
import java.util.List;
@RestController
@RequestMapping("/report")
public class WeatherReportController {
//在应用中添加日志
private final static Logger logger=LoggerFactory.getLogger(WeatherReportController.class);
@Autowired
private WeatherReportService weatherReportService;
@Autowired
private DataClient dataClient;
@GetMapping("/cityId/{cityId}")
//@PathVariable:标识从路径中获取参数
public ModelAndView getReportByCityId(@PathVariable("cityId") String cityId,Model model) throws Exception {
//获取城市列表
List<City> cityList=null;
try {
//由城市数据API微服务来提供数据
cityList = dataClient.listCity();
} catch (Exception e) {
logger.error("Exception!",e);
}
model.addAttribute("title", "天气预报");
model.addAttribute("cityId", cityId);
model.addAttribute("cityList", cityList);
model.addAttribute("report", weatherReportService.getDataByCityId(cityId));
return new ModelAndView("weather/report","reportModel",model);
}
}
修改com.study.spring.cloud.weather.service
包下的WeatherReportServiceImpl
类:
package com.study.spring.cloud.weather.service;
import com.study.spring.cloud.weather.vo.Weather;
import com.study.spring.cloud.weather.vo.WeatherResponse;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class WeatherReportServiceImpl implements WeatherReportService {
@Autowired
private DataClient dataClient;
@Override
public Weather getDataByCityId(String cityId) {
//由天气数据API微服务来提供数据
WeatherResponse resp=dataClient.getDataByCityId(cityId);
Weather data=resp.getData();
return data;
}
}
修改application.properties
配置文件:
#热部署静态文件
spring.thymeleaf.cache=false
#应用名称
spring.application.name=msa-weather-report-eureka-feign-gateway
#注册服务器的URL
eureka.client.service-url.defaultZone=http://localhost:8761/eureka/
#请求服务时的超时时间
feign.client.config.feignName.connect-timeout=5000
#读数据时的超时时间
feign.client.config.feignName.read-timeout=5000
运行
先通过IDE
运行micro-weather-eureka-server
运行Redis
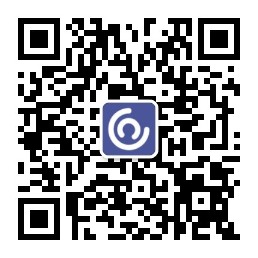
通过命令行在8081
和8082
端口运行msa-weather-collection-eureka-feign
通过命令行在8083
和8084
端口运行msa-weather-data-eureka
通过命令行在8085
和8086
端口运行msa-weather-city-eureka
通过命令行在8087
和8088
端口运行msa-weather-report-eureka-feign-gateway
通过命令行在8089
端口运行msa-weather-eureka-client-zuul
访问http://localhost:8761
页面,可以看到Eureka
的管理页面:
访问http://localhost:8088/report/cityId/101020100
页面:
在页面中切换选中城市:
如果访问页面时报错“Doesn't hava data
”,可能是由于启动msa-weather-collection-eureka-feign
时还未启动msa-weather-city-eureka
,无法获取城市数据,可以将8081
端口的运行先停掉,再重启,就可以了