一.Vue的全局组件注册
Vue.component("组件名称",{})
<div id="app01"> <global-component></global-component> </div> <script src="./static/vue.min.js"></script> <script> Vue.component('global-component',{ template:`<div> <h3>{{ msg }}</h3> </div>`, data(){ return{ msg:'hello vue', } } }); new Vue({ el:'#app01', // template:'' })
二.局部组件注册
const app = new Vue({
el: "#app",
components: {
组件的名称: 组件的配置信息
}
})
let Header={ template:`<div id="d1"> <h1>{{ greeting }}</h1> </div>`, data(){ return {greeting:'hello world'} } }; let app={ template:`<div><app-header></app-header></div>`, components:{'app-header':Header} };
三.子组件的注册,及父子组件通讯
-- 在父组件中注册components
## 注意写组件标签
## 每个组件的template只识别一个作用域块
let son={ template:`<div><span>{{ fData }}</span></div>`, props:['fData'] }; let father={ template:`<div><my-son :fData="fatherdata"></my-son></div>`, data(){return{fatherdata:'alex'}}, components:{'my-son':son} }; new Vue({ el:'#app01', template:`<father></father>`, components:{father} })
四 子父组件通讯
let son={ template:`<div><button @click="sonclick">改变字体大小</button></div>`, methods:{ sonclick:function () { this.$emit('changesize',0.1) } } }; let father={ template:`<div> <span :style="{ fontSize:postFontSize + 'em' }">我是你爸爸</span> <my-son @changesize ="fatherclick"></my-son> </div>`, data(){return{postFontSize:1}}, components:{'my-son':son}, methods:{ fatherclick:function (value) { console.log(value); this.postFontSize += value; } } }
子组件通过$emit提交事件,父组件中的子组件绑定这个事件,监听这个事件用来触发自身的方法,会自动接收子组件的传值
五.非父子组件之间的通讯
扫描二维码关注公众号,回复:
4087587 查看本文章
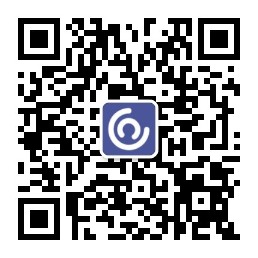
声明中间调度器
-- 其中一个组件向中间调度器提交事件
-- 另一个组件监听中间调度器的事件
-- 注意this的问题
<script> let hanfei = new Vue(); let maweihua = { template: `<div> <h1>这是马伟华</h1> <button @click="my_click">点我向康抻说话</button> </div>`, methods: { my_click: function () { console.log("马") // 向康抻说话 // 向中间调度器提交事件 hanfei.$emit("maweihua_say", "晚上等我一起吃饭~~~") } } }; let kangchen = { template: `<div><h1>这是康抻</h1>{{say}}</div>`, data(){ return { say: "" } }, mounted(){ // 监听中间调度器中的方法 let that = this; hanfei.$on("maweihua_say", function (data) { console.log(data) that.say = data }) } }; const app = new Vue({ el: "#app", components: { maweihua: maweihua, kangchen: kangchen } }) </script>
六.插槽\命名插槽
Vue.component('global-component',{ template:`<div class="box"><slot></slot></div>`, });
Vue.component('global-name',{ template:` <div class="box"> <slot name="lightcourses"></slot> <slot name="degreecourses"></slot> <slot name="home"></slot> </div>` });
七.mix
let mixs = { methods:{ show:function (name) { console.log(name+'comming'); }, hide:function (name) { console.log(name+'goout') } } }; let alex={ template:'#t1', mixins:[mixs] }; let wusir={ template:'#t2', mixins:[mixs] };