public class ValueAnimator
extends Animator 继承Animator
java.lang.Object | ||
↳ | android.animation.Animator | |
↳ | android.animation.ValueAnimator |
这个类为正在运行的动画提供了简单的计时引擎,正在运行的动画计算动画的值并且将它设置在目标对象。
所有动画都使用一个计时脉冲,它运行在自定义的handler去确保属性的改变放生在UI线程。
默认情况下,ValueAnimator 使用非线性时间插入器,通过AccelerateDecelerateInterpolator类,此类加速进入动画并且减速退出动画,这个行为通过调用setInterpolator(TimeInterpolator)来改变。
Animators can be created from either code or resource files. Here is an example of a ValueAnimator resource file:
动画可以通过代码或者资源文件创建。一下是一个ValueAnimator 资源文件的例子。
<animator xmlns:android="http://schemas.android.com/apk/res/android" android:duration="1000" android:valueFrom="1" android:valueTo="0" android:valueType="floatType" android:repeatCount="1" android:repeatMode="reverse"/>从API23开始,同样可以用PropertyValuesHolder
和Keyframe 资源标签去创建多步动画。注意你可以定义明确的小数值(从0到1)为每一个
keyframe 去决定何时动画应该到达那个值。当然,你可以停止小数而keyframes 会被均匀地分配在整个过程。
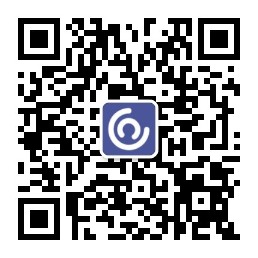
<animator xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="1000"
android:repeatCount="1"
android:repeatMode="reverse">
<propertyValuesHolder>
<keyframe android:fraction="0" android:value="1"/>
<keyframe android:fraction=".2" android:value=".4"/>
<keyframe android:fraction="1" android:value="0"/>
</propertyValuesHolder>
</animator>
Summary 总结
Nested classes 嵌套的类 |
|
---|---|
interface |
ValueAnimator.AnimatorUpdateListener Implementors of this interface can add themselves as update listeners to an 实现这个接口可以将它们自己添加为对ValueAnimator实例更新监听,去接收在每一动画帧的回调,这是发生在当前ValueAnimator帧的值被计算之后。 |
Constants |
|
---|---|
int |
INFINITE This value used used with the 这个值用在 |
int |
RESTART When the animation reaches the end and 当动画结束并且 |
int |
REVERSE When the animation reaches the end and 当动画结束并且 |
Inherited constants 继承类 |
---|
Public constructors 公共构造器 |
|
---|---|
ValueAnimator() Creates a new ValueAnimator object. 创建一个新ValueAnimator 对象 |
Public methods |
|
---|---|
void |
addUpdateListener(ValueAnimator.AnimatorUpdateListener listener) Adds a listener to the set of listeners that are sent update events through the life of an animation. 添加一个监听器到监听器的集合,这个集合在整个动画生命周期被发送更新时间 |
static boolean |
areAnimatorsEnabled() Returns whether animators are currently enabled, system-wide. 返回是否动画当前可操作的 |
void |
cancel() Cancels the animation. 取消动画 |
ValueAnimator |
clone() Creates and returns a copy of this object. 创建并返回这个对象的副本 |
void |
end() Ends the animation. 结束动画 |
float |
getAnimatedFraction() Returns the current animation fraction, which is the elapsed/interpolated fraction used in the most recent frame update on the animation. |
Object |
getAnimatedValue() The most recent value calculated by this
|
Object |
getAnimatedValue(String propertyName) The most recent value calculated by this 获取propertyName 对应的 |
long |
getCurrentPlayTime() Gets the current position of the animation in time, which is equal to the current time minus the time that the animation started. 及时获取当前动画的位置,相当于当前时间减去开始时间 |
long |
getDuration() Gets the length of the animation. 获取动画的时长 |
static long |
getFrameDelay() The amount of time, in milliseconds, between each frame of the animation. 动画的帧与帧之间时间的总量,毫秒 |
TimeInterpolator |
getInterpolator() Returns the timing interpolator that this ValueAnimator uses. 返回ValueAnimator使用的时间差之器 |
int |
getRepeatCount() Defines how many times the animation should repeat. 返回动画应该重复的次数 |
int |
getRepeatMode() Defines what this animation should do when it reaches the end. 返回动画结束时动画应该做什么 |
long |
getStartDelay() The amount of time, in milliseconds, to delay starting the animation after |
long |
getTotalDuration() Gets the total duration of the animation, accounting for animation sequences, start delay, and repeating. 获取动画持续的时间总量,包括顺序,开始延时和重复 |
PropertyValuesHolder[] |
getValues() Returns the values that this ValueAnimator animates between. 返回ValueAnimator 所执行在值。 |
boolean |
isRunning() Returns whether this Animator is currently running (having been started and gone past any initial startDelay period and not yet ended). 返回动画是否在执行。 |
boolean |
isStarted() Returns whether this Animator has been started and not yet ended. 返回是否动画开始并且还没结束 |
static ValueAnimator |
ofArgb(int... values) Constructs and returns a ValueAnimator that animates between color values. 构造并返回一个执行在颜色之间的ValueAnimator |
static ValueAnimator |
ofFloat(float... values) Constructs and returns a ValueAnimator that animates between float values. 构造并返回一个执行在floate值之间的ValueAnimator |
static ValueAnimator |
ofInt(int... values) Constructs and returns a ValueAnimator that animates between int values. 构造并返回一个执行在int值之间的ValueAnimator |
static ValueAnimator |
ofObject(TypeEvaluator evaluator, Object... values) Constructs and returns a ValueAnimator that animates between Object values. 构造并返回一个执行在Object 值之间的ValueAnimator |
static ValueAnimator |
ofPropertyValuesHolder(PropertyValuesHolder... values) Constructs and returns a ValueAnimator that animates between the values specified in the PropertyValuesHolder objects. 构造并返回一个执行在定义在PropertyValuesHolder值之间的ValueAnimator |
void |
pause() Pauses a running animation. 暂停动画 |
void |
removeAllUpdateListeners() Removes all listeners from the set listening to frame updates for this animation. 清除所有更新监听 |
void |
removeUpdateListener(ValueAnimator.AnimatorUpdateListener listener) Removes a listener from the set listening to frame updates for this animation. 清除集合中的指定监听 |
void |
resume() Resumes a paused animation, causing the animator to pick up where it left off when it was paused. 使暂停的动画获取焦点 |
void |
reverse() Plays the ValueAnimator in reverse. 反向执行动画 |
void |
setCurrentFraction(float fraction) Sets the position of the animation to the specified fraction. 设置动画的位置到指定的小数值 |
void |
setCurrentPlayTime(long playTime) Sets the position of the animation to the specified point in time. 设置动画的位置到指定的时间点 |
ValueAnimator |
setDuration(long duration) Sets the length of the animation. 设置动画执行的时长 |
void |
setEvaluator(TypeEvaluator value) The type evaluator to be used when calculating the animated values of this animation. 类型估值器用在计算动画执行值时 |
void |
setFloatValues(float... values) Sets float values that will be animated between. 设置动画执行的值 |
static void |
setFrameDelay(long frameDelay) The amount of time, in milliseconds, between each frame of the animation. 帧与帧之间的时长 |
void |
setIntValues(int... values) Sets int values that will be animated between. 设置动画执行的值 |
void |
setInterpolator(TimeInterpolator value) The time interpolator used in calculating the elapsed fraction of this animation. 设置时间差值器 |
void |
setObjectValues(Object... values) Sets the values to animate between for this animation. |
void |
setRepeatCount(int value) Sets how many times the animation should be repeated. 设置重复次数 |
void |
setRepeatMode(int value) Defines what this animation should do when it reaches the end. 设置动画结束时的动作 |
void |
setStartDelay(long startDelay) The amount of time, in milliseconds, to delay starting the animation after 设置开始延时 |
void |
setValues(PropertyValuesHolder... values) Sets the values, per property, being animated between. |
void |
start() Starts this animation. |
String |
toString() Returns a string representation of the object. |
Inherited methods |
|
---|---|