JQ03
1、val方法
val方法用于设置和获取表单元素的值,如input/textarea
1)设置与获取:
.val(“需要设置的字符串”);
.val();//获取字符串
2)focus();//方法,获取焦点时触发focus事件
.focus(fuction(){
});
.blur();//失去焦点
只要把js中的相关函数名中的on去掉即可
2、html与text方法
html方法相当于innerHTML。text方法相当于innerText
使用text()时不必考虑兼容性问题
html方法会识别html标签,而text方法会直接把标签内容作为字符串,不识别。
获取时text会直接忽略指定标签内的标签,html则会把标签内容作为文本获取过来
3、width和height方法
js三大家族offset/scroll/client
.width();//获取元素的宽度,返回一个数字
.width(num);//设置元素的宽度
height的用法完全类似
//获取页面可视区域的宽高
resize()事件:
.resize(function(){…});
$(window).resize(function(){
});
width()方法获取的仅是元素width属性的值
而innerWidth()获取的是元素padding+width的值
outerWidth()默认获取的是元素padding+width+border的值
若传参数true,则会将margin值也加上
4、.scroll()事件
.scroll(function(){…});
.scrollTop()方法
返回或设置匹配元素的滚动条的垂直位置
.scrollLeft()
返回或设置匹配元素的滚动条的水平位置
案例:固定导航栏
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title></title>
<style>
* {
margin: 0;
padding: 0
}
img {
vertical-align: top;
}
.main {
margin: 10px auto 0;
width: 1000px;
}
.fixed {
position: fixed;
top: 0;
left: 0;
}
</style>
<script src="../jquery-1.12.4.js"></script>
<script>
$(function () {
$(window).scroll(function () {
//判断卷去的高度超过topPart的高度
//1. 让navBar有固定定位
//2. 让mainPart有一个marginTop
if($(window).scrollTop() >= $(".top").height() ){
$(".nav").addClass("fixed");
$(".main").css("marginTop", $(".nav").height() + 10);
}else {
$(".nav").removeClass("fixed");
$(".main").css("marginTop", 10);
}
});
});
</script>
</head>
<body>
<div class="top" id="topPart">
<img src="images/top.png" alt=""/>
</div>
<div class="nav" id="navBar">
<img src="images/nav.png" alt=""/>
</div>
<div class="main" id="mainPart">
<img src="images/main.png" alt=""/>
</div>
</body>
</html>
案例:返回顶部的火箭
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
body {
height: 8000px;
}
a {
color: #FFF;
}
.actGotop {
position: fixed;
bottom: 50px;
right: 50px;
width: 150px;
height: 195px;
display: none;
z-index: 100;
}
.actGotop a, .actGotop a:link {
width: 150px;
height: 195px;
display: inline-block;
background: url(images/gotop.png) no-repeat;
outline: none;
}
.actGotop a:hover {
width: 150px;
height: 195px;
background: url(images/gotop.gif) no-repeat;
outline: none;
}
</style>
</head>
<body>
<!-- 返回顶部小火箭 -->
<div class="actGotop"><a href="javascript:;" title="Top"></a></div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
//当页面超出去1000px的时候,让小火箭显示出来,如果小于1000,就让小火箭隐藏
$(window).scroll(function () {
if($(window).scrollTop() >= 1000 ){
$(".actGotop").stop().fadeIn(1000);
}else {
$(".actGotop").stop().fadeOut(1000);
}
});
function getScroll(){
return {
left:window.pageYOffset || document.documentElement.scrollLeft || document.body.scrollLeft || 0,
top:window.pageYOffset || document.documentElement.scrollTop || document.body.scrollTop || 0
}
}
//在外面注册
$(".actGotop").click(function () {
//$("html,body").stop().animate({scrollTop:0},3000);
//scrollTop为0
//$(window).scrollTop(0);
})
});
</script>
</body>
</html>
4、offset方法和position方法
用于获取元素的位置。
.offset();//返回object对象,含元素相对于document的left和top属性值
.position();//获取元素相对于有定位的父元素的位置
5、JQ事件
1)事件发展历程
bind方式注册事件(了解)
委托事件的注册:
委托事件可以解决新注册的元素没有之前既有相同元素的事件的问题,这是由于在页面加载完成时事件已全部注册完毕而动态元素还未创建的问题。主要借助事件冒泡原理。调用元素必须是触发元素的祖先元素
.delegate(selector,types,data,fn);
其中types为事件类型,data可缺省,fn为触发事件时调用的函数。注意,事件不是由调用它的元素触发,而是由参数第一项所选择的元素触发
2)on注册事件
有JQ统一的事件处理方法
注册简单事件(表示给调用元素绑定事件,仅能由自己触发,不支持动态绑定):
$(selector).on(“事件类型”,function(){});
注册委托事件:
$(selector).on(“事件类型”,selector,function(){});
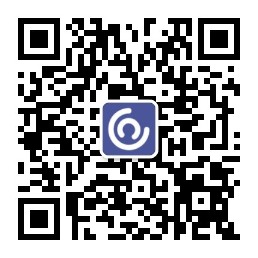
6、事件的执行顺序
点击p元素,执行输出:呵呵哒-嘿嘿嘿-呜呜呜
即先执行p现有的事件,再执行委托事件,最后才是父级冒泡事件
对于被绑定了委托事件的div来说,先要处理完自己身上被委托的事件,才能去执行自己的简单事件
表格删除案例:
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title></title>
<style>
* {
padding: 0;
margin: 0;
}
.wrap {
width: 410px;
margin: 100px auto 0;
}
table {
border-collapse: collapse;
border-spacing: 0;
border: 1px solid #c0c0c0;
}
th,
td {
border: 1px solid #d0d0d0;
color: #404060;
padding: 10px;
}
th {
background-color: #09c;
font: bold 16px "΢ÈíÑźÚ";
color: #fff;
}
td {
font: 14px "΢ÈíÑźÚ";
}
td a.get {
text-decoration: none;
}
a.del:hover {
text-decoration: underline;
}
tbody tr {
background-color: #f0f0f0;
}
tbody tr:hover {
cursor: pointer;
background-color: #fafafa;
}
.btnAdd {
width: 110px;
height: 30px;
font-size: 20px;
font-weight: bold;
}
.form-item {
height: 100%;
position: relative;
padding-left: 100px;
padding-right: 20px;
margin-bottom: 34px;
line-height: 36px;
}
.form-item > .lb {
position: absolute;
left: 0;
top: 0;
display: block;
width: 100px;
text-align: right;
}
.form-item > .txt {
width: 300px;
height: 32px;
}
.mask {
position: absolute;
top: 0px;
left: 0px;
width: 100%;
height: 100%;
background: #000;
opacity: 0.15;
display: none;
}
.form-add {
position: fixed;
top: 30%;
left: 50%;
margin-left: -197px;
padding-bottom: 20px;
background: #fff;
display: none;
}
.form-add-title {
background-color: #f7f7f7;
border-width: 1px 1px 0 1px;
border-bottom: 0;
margin-bottom: 15px;
position: relative;
}
.form-add-title span {
width: auto;
height: 18px;
font-size: 16px;
font-family: ËÎÌå;
font-weight: bold;
color: rgb(102, 102, 102);
text-indent: 12px;
padding: 8px 0px 10px;
margin-right: 10px;
display: block;
overflow: hidden;
text-align: left;
}
.form-add-title div {
width: 16px;
height: 20px;
position: absolute;
right: 10px;
top: 6px;
font-size: 30px;
line-height: 16px;
cursor: pointer;
}
.form-submit {
text-align: center;
}
.form-submit input {
width: 170px;
height: 32px;
}
</style>
</head>
<body>
<div class="wrap">
<input type="button" value="清空内容" id="btn">
<input type="button" value="添加" id="btnAdd">
<table>
<thead>
<tr>
<th>课程名称</th>
<th>所属学院</th>
<th>操作</th>
</tr>
</thead>
<tbody id="j_tb">
<tr>
<!-- <td><input type="checkbox"/></td> -->
<td>JavaScript</td>
<td>传智播客-前端与移动开发学院</td>
<td><a href="javascrip:;" class="get">DELETE</a></td>
</tr>
<tr>
<!-- <td><input type="checkbox"/></td> -->
<td>css</td>
<td>传智播客-前端与移动开发学院</td>
<td><a href="javascrip:;" class="get">DELETE</a></td>
</tr>
<tr>
<!-- <td><input type="checkbox"/></td> -->
<td>html</td>
<td>传智播客-前端与移动开发学院</td>
<td><a href="javascrip:;" class="get">DELETE</a></td>
</tr>
<tr>
<td>jQuery</td>
<td>传智播客-前端与移动开发学院</td>
<td><a href="javascrip:;" class="get">DELETE</a></td>
</tr>
</tbody>
</table>
</div>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
//1. 找到清空按钮,注册点击事件,清空tbody
$("#btn").on("click", function () {
$("#j_tb").empty();
});
//2. 找到delete,注册点击事件
// $(".get").on("click", function () {
// $(this).parent().parent().remove();
// });
$("#j_tb").on("click", ".get", function () {
$(this).parent().parent().remove();
});
//3. 找到添加按钮,注册点击事件
$("#btnAdd").on("click", function () {
$('<tr> <td>jQuery111</td> <td>传智播客-前端与移动开发学院111</td> <td><a href="javascrip:;" class="get">DELETE</a></td> </tr>').appendTo("#j_tb");
});
});
</script>
</body>
</html>
7、JQ事件对象
打印的结果都是0,因为事件只在点击的时候执行
为了保留money的初值。利用on方法中的data函数进行暂存,但不常用。
阻止冒泡和默认行为:
$(selector).on(“事件类型”,function(e)){
e.preventDefault();//阻止默认行为
e.stopPropagation();//阻止冒泡
}
//钢琴案例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
* {
margin: 0;
padding: 0;
list-style: none;
}
.nav {
width: 900px;
height: 60px;
background-color: black;
margin: 0 auto;
}
.nav li {
width: 100px;
height: 60px;
/*border: 1px solid yellow;*/
float: left;
position: relative;
overflow: hidden;
}
.nav a {
position: absolute;
width: 100%;
height: 100%;
font-size: 24px;
color: blue;
text-align: center;
line-height: 60px;
text-decoration: none;
z-index: 2;
}
.nav span {
position: absolute;
width: 100%;
height: 100%;
background-color: yellow;
top: 60px;
}
</style>
<script src="../jquery-1.12.4.js"></script>
<script>
$(function () {
//给li注册鼠标进入事件,让li下面的span top:0 播放音乐
$(".nav li").mouseenter(function () {
$(this).children("span").stop().animate({top: 0});
//播放音乐
var idx = $(this).index();
$(".nav audio").get(idx).load();
$(".nav audio").get(idx).play();
}).mouseleave(function () {
$(this).children("span").stop().animate({top: 60});
});
//节流阀 :按下的时候,触发,如果没弹起,不让触发下一次
//1. 定义一个flag
var flag = true;
//按下1-9这几个数字键,能触发对应的mouseenter事件
$(document).on("keydown", function (e) {
if(flag) {
flag = false;
//获取到按下的键
var code = e.keyCode;
if(code >= 49 && code <= 57){
//触发对应的li的mouseenter事件
$(".nav li").eq(code - 49).mouseenter();
}
}
});
$(document).on("keyup", function (e) {
flag = true;
//获取到按下的键
var code = e.keyCode;
if(code >= 49 && code <= 57){
//触发对应的li的mouseenter事件
$(".nav li").eq(code - 49).mouseleave();
}
});
//弹起的时候,触发mouseleave事件
});
</script>
</head>
<body>
<div class="nav">
<ul>
<li>
<a href="javascript:void(0);">导航1</a>
<span></span>
</li>
<li><a href="javascript:void(0);">导航2</a><span></span></li>
<li><a href="javascript:void(0);">导航3</a><span></span></li>
<li><a href="javascript:void(0);">导航4</a><span></span></li>
<li><a href="javascript:void(0);">导航5</a><span></span></li>
<li><a href="javascript:void(0);">导航6</a><span></span></li>
<li><a href="javascript:void(0);">导航7</a><span></span></li>
<li><a href="javascript:void(0);">导航8</a><span></span></li>
<li><a href="javascript:void(0);">导航9</a><span></span></li>
</ul>
<div>
<audio src="mp3/1.ogg"></audio>
<audio src="mp3/2.ogg"></audio>
<audio src="mp3/3.ogg"></audio>
<audio src="mp3/4.ogg"></audio>
<audio src="mp3/5.ogg"></audio>
<audio src="mp3/6.ogg"></audio>
<audio src="mp3/7.ogg"></audio>
<audio src="mp3/8.ogg"></audio>
<audio src="mp3/9.ogg"></audio>
</div>
</div>
</body>
</html>
keydown事件如果按键不撤离会一直触发,使用节流阀解决:按下时触发,如果没弹起就不触发
节流阀的添加:
先定义一个变量flag=true;
按下时flag=false
弹起后flag=true
delay()方法:
delay(数字);延迟给定毫秒的延迟
8、链式编程
通常情况下,只有设置操作才能把链式编程延续下去。获取性操作不能链式。
//五角星评分案例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>五角星评分案例</title>
<style>
* {
padding: 0;
margin: 0;
}
.comment {
font-size: 40px;
color: #ff16cf;
}
.comment li {
float: left;
}
ul {
list-style: none;
}
</style>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
//1. 给li注册鼠标经过事件,让自己和前面所有的兄弟都变成实心
var wjx_k = "☆";
var wjx_s = "★";
$(".comment>li").on("mouseenter", function () {
$(this).text(wjx_s).prevAll().text(wjx_s);
$(this).nextAll().text(wjx_k);
});
//2. 给ul注册鼠标离开时间,让所有的li都变成空心
$(".comment").on("mouseleave", function () {
$(this).children().text(wjx_k);
//再做一件事件,找到current,让current和current前面的变成实心就行。
$("li.current").text(wjx_s).prevAll().text(wjx_s);
});
//3. 给li注册点击事件
$(".comment>li").on("click", function () {
$(this).addClass("current").siblings().removeClass("current");
});
});
</script>
</head>
<body>
<ul class="comment">
<li>☆</li>
<li>☆</li>
<li>☆</li>
<li>☆</li>
<li>☆</li>
</ul>
</body>
</html>
.prevAll();//之前所有兄弟元素
.nextAll();//之后所有兄弟元素
.end(“方法”);获取的方法在链式编程中使DOM对象变化时,将改变的dom对象还原回之前的那个对象,以便继续链式编程
each();方法
jq隐式迭代会对所有的dom对象设置相同的值,但如果我们需要给每一个对象设置不同的值,则需要自行进行迭代。或者使用each方法
这个方法将遍历jq对象集合,为每个匹配的元素执行一个函数
$(selector).each(function(index,element){});
参数1为当前元素在所有匹配元素中的索引号,参数2表示当前元素(dom对象)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>复制</title>
<style>
ul {
list-style: none;
}
li {
float: left;
width: 200px;
height: 200px;
background: pink;
text-align: center;
line-height: 200px;
margin: 0 20px 20px 0;
}
</style>
<script src="jquery-1.12.4.js"></script>
<script>
$(function () {
// for(var i = 0; i < $("li").length; i++) {
// $("li").eq(i).css("opacity", (i+1)/10);
// }
//each方法
$("li").each(function (index, element) {
$(element).css("opacity", (index+1)/10);
})
});
</script>
</head>
<body>
<ul id="ulList">
<li>什么都看不见</li>
<li>什么都看不见</li>
<li>什么都看不见</li>
<li>什么都看不见</li>
<li>什么都看不见</li>
<li>什么都看不见</li>
<li>什么都看不见</li>
<li>什么都看不见</li>
<li>什么都看不见</li>
<li>什么都看不见</li>
</ul>
</body>
</html>
8、美元符冲突的问题
在别的框架中,也有可能会使用到美元符。
解决方法:让jq框架放弃对$的控制权
$.noConflict();
$可被jQuery替换或者自定义变量替代美元符