版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/deeplan_1994/article/details/83146521
目录
1.std::count()
template <class InputIterator, class T>
typename iterator_traits<InputIterator>::difference_type
count (InputIterator first, InputIterator last, const T& val);
作用:计算范围内值T出现的次数。对迭代器范围内的元素每个值进行比较,时间复杂度是线性的。
参数:输入的迭代器范围[ first, last),待匹配的值val
输出:范围内[first,last)
比较等于val的元素数。
例子:
// count algorithm example
#include <iostream> // std::cout
#include <algorithm> // std::count
#include <vector> // std::vector
int main () {
// counting elements in array:
int myints[] = {10,20,30,30,20,10,10,20}; // 8 elements
int mycount = std::count (myints, myints+8, 10);
std::cout << "10 appears " << mycount << " times.\n";
// counting elements in container:
std::vector<int> myvector (myints, myints+8);
mycount = std::count (myvector.begin(), myvector.end(), 20);
std::cout << "20 appears " << mycount << " times.\n";
return 0;
}
2.std::find()
template <class InputIterator, class T>
InputIterator find (InputIterator first, InputIterator last, const T& val);
作用:在迭代器范围[first, last)找值val,如果找到,返回一个迭代器指向该值首次出现的位置。如果没有找到,返回 last迭代器。
扫描二维码关注公众号,回复:
4012200 查看本文章
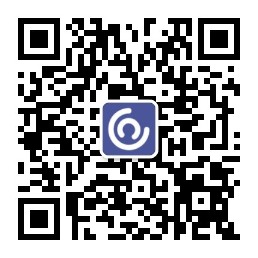
例子:
// find example
#include <iostream> // std::cout
#include <algorithm> // std::find
#include <vector> // std::vector
int main () {
// using std::find with array and pointer:
int myints[] = { 10, 20, 30, 40 };
int * p;
p = std::find (myints, myints+4, 30);
if (p != myints+4)
std::cout << "Element found in myints: " << *p << '\n';
else
std::cout << "Element not found in myints\n";
// using std::find with vector and iterator:
std::vector<int> myvector (myints,myints+4);
std::vector<int>::iterator it;
it = find (myvector.begin(), myvector.end(), 30);
if (it != myvector.end())
std::cout << "Element found in myvector: " << *it << '\n';
else
std::cout << "Element not found in myvector\n";
return 0;
}
3.std::for_each()
template <class InputIterator, class Function>
Function for_each (InputIterator first, InputIterator last, Function fn);
作用:
将功能应用于范围
将函数fn应用于范围中的每个元素[first,last)
。
参数:fn -- 一元函数接受范围内的元素作为参数。其返回值将被忽略。
例子:
#for_each example
#include<iostream>
#include<algorithm>
#include<vector>
void myfunction(int i) // function:
{
std::cout<<' '<<i;
}
struct myclass{ // function object type:
void operator()(int i){std::cout<<' '<<i;
}myobject;
int main()
{
std::vector<int> myvector;
myvecotr.push_back(10);
myvecotr.push_back(20);
myvecotr.push_back(30);
std::cout<<"myvector contains:";
for_each(myvector.begin(),myvector.end(),myfunction);
std::cout<<std::endl;
//or
std::cout<<"myvector contains:";
for_each(myvector.begin(),myvector.end(),myobject);
std::cout<<'\n';
retrun 0;
}
4.std::sort()
default (1)
template <class RandomAccessIterator>
void sort (RandomAccessIterator first, RandomAccessIterator last);
custom (2)
template <class RandomAccessIterator, class Compare>
void sort (RandomAccessIterator first, RandomAccessIterator last, Compare comp);
作用:排序范围内[ first, last) 的元素按升序排序。
参数:Compare 二进制函数,接受范围中的两个元素作为参数,并返回可转换为的值bool
。返回的值表示作为第一个参数传递的元素是否被认为是在它定义的特定严格弱顺序中的第二个参数之前。
该函数不得修改其任何参数。
这可以是函数指针或函数对象。
返回值:没有
例子:
// sort algorithm example
#include <iostream> // std::cout
#include <algorithm> // std::sort
#include <vector> // std::vector
bool myfunction (int i,int j) { return (i<j); }
struct myclass {
bool operator() (int i,int j) { return (i<j);}
} myobject;
int main () {
int myints[] = {32,71,12,45,26,80,53,33};
std::vector<int> myvector (myints, myints+8); // 32 71 12 45 26 80 53 33
// using default comparison (operator <):
std::sort (myvector.begin(), myvector.begin()+4); //(12 32 45 71)26 80 53 33
// using function as comp
std::sort (myvector.begin()+4, myvector.end(), myfunction); // 12 32 45 71(26 33 53 80)
// using object as comp
std::sort (myvector.begin(), myvector.end(), myobject); //(12 26 32 33 45 53 71 80)
// print out content:
std::cout << "myvector contains:";
for (std::vector<int>::iterator it=myvector.begin(); it!=myvector.end(); ++it)
std::cout << ' ' << *it;
std::cout << '\n';
return 0;
}