title: String源码解析一
date: 2018-11-07 15:00:00
tags: JAVA基础
一.类定义、属性与构造函数
类定义
public final class String
implements java.io.Serializable, Comparable<String>, CharSequence {
final说明类是常量,String类实现了Serializable, Comparable, CharSequence接口,Comparable接口有compareTo方法,而CharSequence接口有length、charAt、subSequence、toString方法,jdk1.8新增了chars和codePoints方法。
属性
/** The value is used for character storage. */
private final char value[];
/** Cache the hash code for the string */
private int hash; // Default to 0
/** use serialVersionUID from JDK 1.0.2 for interoperability */
private static final long serialVersionUID = -6849794470754667710L;
/**
* Class String is special cased within the Serialization Stream Protocol.
*
* A String instance is written into an ObjectOutputStream according to
* <a href="{@docRoot}/../platform/serialization/spec/output.html">
* Object Serialization Specification, Section 6.2, "Stream Elements"</a>
*/
private static final ObjectStreamField[] serialPersistentFields =
new ObjectStreamField[0];
value:不可变字符数组,用来存储String值
hash:用来缓存计算后的hash 值
常用构造函数
public String() {
this.value = "".value;
}
public String(String original) {
this.value = original.value;
this.hash = original.hash;
}
public String(char value[]) {
this.value = Arrays.copyOf(value, value.length);
}
public String(char value[], int offset, int count) {
if (offset < 0) {
throw new StringIndexOutOfBoundsException(offset);
}
if (count <= 0) {
if (count < 0) {
throw new StringIndexOutOfBoundsException(count);
}
if (offset <= value.length) {
this.value = "".value;
return;
}
}
// Note: offset or count might be near -1>>>1.
if (offset > value.length - count) {
throw new StringIndexOutOfBoundsException(offset + count);
}
this.value = Arrays.copyOfRange(value, offset, offset+count);
}
二.常用方法
应用的最多的方法,需要重点关注下!
length、isEmpty、charAt
//字符串的长度
public int length() {
return value.length;
}
//是否为空
public boolean isEmpty() {
return value.length == 0;
}
//返回某个索引下的字符
public char charAt(int index) {
if ((index < 0) || (index >= value.length)) {
throw new StringIndexOutOfBoundsException(index);
}
return value[index];
}
equals:判断两个String对象是否相等
两个String对象的规则如下:
1.首先判断两个对象的内存地址是否相同,若是,返回true
2.判断比较的对象是否是String类型
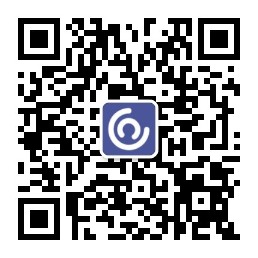
3.比较两个对象的长度是否相等
4.比较两个对象的每个字符是否相等
public boolean equals(Object anObject) {
//1.首先判断是否是对象本身,若是,返回true
if (this == anObject) {
return true;
}
//2.判断比较的对象是否是String类型
if (anObject instanceof String) {
String anotherString = (String)anObject;
int n = value.length;
//3.比较两个对象的长度是否相等
if (n == anotherString.value.length) {
char v1[] = value;
char v2[] = anotherString.value;
int i = 0;
//4.比较两个对象的每个字符是否相等
while (n-- != 0) {
if (v1[i] != v2[i])
return false;
i++;
}
return true;
}
}
return false;
}
compareTo:比较两个String对象的大小
public int compareTo(String anotherString) {
int len1 = value.length;
int len2 = anotherString.value.length;
//1.获取两个字符串对象的长度最小值
int lim = Math.min(len1, len2);
//2.将两个字符串转换成字符数组
char v1[] = value;
char v2[] = anotherString.value;
int k = 0;
//3.从两个字符数组的第一个字符开始比较,直到最小值lim为止
while (k < lim) {
char c1 = v1[k];
char c2 = v2[k];
if (c1 != c2) {
return c1 - c2;
}
k++;
}
//4.若之前的字符都相同,则比较两个字符串的长度,长度长的大
return len1 - len2;
}
startsWith:是否以某个指定的字符串开始
public boolean startsWith(String prefix, int toffset) {
char ta[] = value;
int to = toffset;
char pa[] = prefix.value;
int po = 0;
int pc = prefix.value.length;
// Note: toffset might be near -1>>>1.
//1.判定toffset是否符合规范,如果起始地址小于0或者(起始地址+所比较对象长度)大于自身对象长度,返回false
if ((toffset < 0) || (toffset > value.length - pc)) {
return false;
}
//2.对象从指定位置与比较对象依次比较是否相同
while (--pc >= 0) {
if (ta[to++] != pa[po++]) {
return false;
}
}
return true;
}
public boolean startsWith(String prefix) {
return startsWith(prefix, 0);
}
indexOf:返回第一次出现的指定字符串的索引位置
public int indexOf(String str) {
return indexOf(str, 0);
}
public int indexOf(String str, int fromIndex) {
return indexOf(value, 0, value.length,
str.value, 0, str.value.length, fromIndex);
}
static int indexOf(char[] source, int sourceOffset, int sourceCount,
char[] target, int targetOffset, int targetCount,
int fromIndex) {
//1.判断参数fromIndex的合法性
if (fromIndex >= sourceCount) {
return (targetCount == 0 ? sourceCount : -1);
}
if (fromIndex < 0) {
fromIndex = 0;
}
if (targetCount == 0) {
return fromIndex;
}
//2.获取目标字符串第一个字符和源字符串和目标字符串的长度差
char first = target[targetOffset];
int max = sourceOffset + (sourceCount - targetCount);
for (int i = sourceOffset + fromIndex; i <= max; i++) {
/* Look for first character. */
//3.寻找源字符串中匹配的第一个字符
if (source[i] != first) {
while (++i <= max && source[i] != first);
}
/* Found first character, now look at the rest of v2 */
//4.找到第一个字符后,依次匹配剩下的字符,并返回源字符串的索引位置
if (i <= max) {
int j = i + 1;
int end = j + targetCount - 1;
for (int k = targetOffset + 1; j < end && source[j]
== target[k]; j++, k++);
if (j == end) {
/* Found whole string. */
return i - sourceOffset;
}
}
}
//5.若不匹配,返回-1
return -1;
}
substring:获取指定索引开始的子字符串
public String substring(int beginIndex) {
if (beginIndex < 0) {
throw new StringIndexOutOfBoundsException(beginIndex);
}
int subLen = value.length - beginIndex;
if (subLen < 0) {
throw new StringIndexOutOfBoundsException(subLen);
}
return (beginIndex == 0) ? this : new String(value, beginIndex, subLen);
}
concat:将指定的字符串参数连接到字符串上
public String concat(String str) {
int otherLen = str.length();
//1.若拼接字符串为空,返回原字符串
if (otherLen == 0) {
return this;
}
int len = value.length;
//2.将指定字符串拼接在原字符串上,并返回新的字符串对象
char buf[] = Arrays.copyOf(value, len + otherLen);
str.getChars(buf, len);
return new String(buf, true);
}
replace:替换指定的字符
public String replace(char oldChar, char newChar) {
if (oldChar != newChar) {
int len = value.length;
int i = -1;
char[] val = value; /* avoid getfield opcode */
//1.获取源字符串中出现指定字符串的索引位置
while (++i < len) {
if (val[i] == oldChar) {
break;
}
}
if (i < len) {
char buf[] = new char[len];
//2.将指定源字符串中出现指定字符之前的字符串记录下来
for (int j = 0; j < i; j++) {
buf[j] = val[j];
}
while (i < len) {
//3.将后面的字符依次替换为新字符
char c = val[i];
buf[i] = (c == oldChar) ? newChar : c;
i++;
}
//4.返回新创建的字符串
return new String(buf, true);
}
}
return this;
}
//正则表达式匹配
public String replace(CharSequence target, CharSequence replacement) {
return Pattern.compile(target.toString(), Pattern.LITERAL).matcher(
this).replaceAll(Matcher.quoteReplacement(replacement.toString()));
}
public String replaceFirst(String regex, String replacement) {
return Pattern.compile(regex).matcher(this).replaceFirst(replacement);
}
public String replaceAll(String regex, String replacement) {
return Pattern.compile(regex).matcher(this).replaceAll(replacement);
}
split:根据所传递的regex字符串分割当前对象
public String[] split(String regex) {
return split(regex, 0);
}
public String[] split(String regex, int limit) {
/* fastpath if the regex is a
(1)one-char String and this character is not one of the
RegEx's meta characters ".$|()[{^?*+\\", or
(2)two-char String and the first char is the backslash and
the second is not the ascii digit or ascii letter.
*/
//1.字符串匹配的原则一,如果是一个字符的话不能是正则表达式的元字符
//2.字符串匹配的原则二,如果是两个字符的话第一个不能是反斜杠,第二个不能是数字或字母
//3.我们这里设定limit=0,也就是split(String regex)方法的使用
char ch = 0;
if (((regex.value.length == 1 &&
".$|()[{^?*+\\".indexOf(ch = regex.charAt(0)) == -1) ||
(regex.length() == 2 &&
regex.charAt(0) == '\\' &&
(((ch = regex.charAt(1))-'0')|('9'-ch)) < 0 &&
((ch-'a')|('z'-ch)) < 0 &&
((ch-'A')|('Z'-ch)) < 0)) &&
(ch < Character.MIN_HIGH_SURROGATE ||
ch > Character.MAX_LOW_SURROGATE))
{
int off = 0;
int next = 0;
boolean limited = limit > 0;
ArrayList<String> list = new ArrayList<>();
while ((next = indexOf(ch, off)) != -1) {
//4.字符串中依次匹配regex字符
if (!limited || list.size() < limit - 1) {
list.add(substring(off, next));
off = next + 1;
} else { // last one
//assert (list.size() == limit - 1);
list.add(substring(off, value.length));
off = value.length;
break;
}
}
//5.若一个都没匹配上,返回原字符串数组
// If no match was found, return this
if (off == 0)
return new String[]{this};
// Add remaining segment
//6.匹配剩下的字符串拼接到数组后
if (!limited || list.size() < limit)
list.add(substring(off, value.length));
// Construct result
int resultSize = list.size();
if (limit == 0) {
//7.确定匹配后实际的字符串数组长度
while (resultSize > 0 && list.get(resultSize - 1).length() == 0) {
resultSize--;
}
}
//8.返回最终匹配的字符串数组
String[] result = new String[resultSize];
return list.subList(0, resultSize).toArray(result);
}
//9.若不是上面的匹配情况,进行正则匹配
return Pattern.compile(regex).split(this, limit);
}
toLowerCase:将所有字符串转换成小写
使用给定的Locale的规则将此String中的所有字符转换为小写。
大小写映射基于Character类指定的Unicode标准版本,由于大小写映射并不总是1:1字符映射,因此生成的字符串可能与原始字符串的长度不同
public String toLowerCase() {
return toLowerCase(Locale.getDefault());
}
public String toLowerCase(Locale locale) {
if (locale == null) {
throw new NullPointerException();
}
int firstUpper;
final int len = value.length;
/* Now check if there are any characters that need to be changed. */
//1.扫描字符串是否存在大写的字符
scan: {
for (firstUpper = 0 ; firstUpper < len; ) {
char c = value[firstUpper];
if ((c >= Character.MIN_HIGH_SURROGATE)
&& (c <= Character.MAX_HIGH_SURROGATE)) {
int supplChar = codePointAt(firstUpper);
if (supplChar != Character.toLowerCase(supplChar)) {
break scan;
}
firstUpper += Character.charCount(supplChar);
} else {
if (c != Character.toLowerCase(c)) {
break scan;
}
firstUpper++;
}
}
return this;
}
char[] result = new char[len];
int resultOffset = 0; /* result may grow, so i+resultOffset
* is the write location in result */
/* Just copy the first few lowerCase characters. */
//2.拷贝前面的小写字符集
System.arraycopy(value, 0, result, 0, firstUpper);
String lang = locale.getLanguage();
boolean localeDependent =
(lang == "tr" || lang == "az" || lang == "lt");
char[] lowerCharArray;
int lowerChar;
int srcChar;
int srcCount;
//3.由于大写与小写的字符比例不一定是1:1,所以转换成小写时需要统计转换后的字符串长度再转换
for (int i = firstUpper; i < len; i += srcCount) {
srcChar = (int)value[i];
if ((char)srcChar >= Character.MIN_HIGH_SURROGATE
&& (char)srcChar <= Character.MAX_HIGH_SURROGATE) {
srcChar = codePointAt(i);
srcCount = Character.charCount(srcChar);
} else {
srcCount = 1;
}
if (localeDependent ||
srcChar == '\u03A3' || // GREEK CAPITAL LETTER SIGMA
srcChar == '\u0130') { // LATIN CAPITAL LETTER I WITH DOT ABOVE
lowerChar = ConditionalSpecialCasing.toLowerCaseEx(this, i, locale);
} else {
lowerChar = Character.toLowerCase(srcChar);
}
if ((lowerChar == Character.ERROR)
|| (lowerChar >= Character.MIN_SUPPLEMENTARY_CODE_POINT)) {
if (lowerChar == Character.ERROR) {
lowerCharArray =
ConditionalSpecialCasing.toLowerCaseCharArray(this, i, locale);
} else if (srcCount == 2) {
resultOffset += Character.toChars(lowerChar, result, i + resultOffset) - srcCount;
continue;
} else {
lowerCharArray = Character.toChars(lowerChar);
}
/* Grow result if needed */
int mapLen = lowerCharArray.length;
if (mapLen > srcCount) {
char[] result2 = new char[result.length + mapLen - srcCount];
System.arraycopy(result, 0, result2, 0, i + resultOffset);
result = result2;
}
for (int x = 0; x < mapLen; ++x) {
result[i + resultOffset + x] = lowerCharArray[x];
}
resultOffset += (mapLen - srcCount);
} else {
result[i + resultOffset] = (char)lowerChar;
}
}
return new String(result, 0, len + resultOffset);
}
toLowerCase:将所有字符串转换成大写,源码类似于toLowerCase
trim:过滤掉字符串首尾空格
public String trim() {
int len = value.length;
int st = 0;
char[] val = value; /* avoid getfield opcode */
//1.去掉起始空格
while ((st < len) && (val[st] <= ' ')) {
st++;
}
//2.去掉末尾空格
while ((st < len) && (val[len - 1] <= ' ')) {
len--;
}
return ((st > 0) || (len < value.length)) ? substring(st, len) : this;
}
valueOf:将其他类型的值转换为String类型
public static String valueOf(Object obj) {
return (obj == null) ? "null" : obj.toString();
}
public static String valueOf(char data[]) {
return new String(data);
}
public static String valueOf(boolean b) {
return b ? "true" : "false";
}
public static String valueOf(char c) {
char data[] = {c};
return new String(data, true);
}
public static String valueOf(int i) {
return Integer.toString(i);
}
public static String valueOf(long l) {
return Long.toString(l);
}
public static String valueOf(float f) {
return Float.toString(f);
}
public static String valueOf(double d) {
return Double.toString(d);
}