其实啊,我写这篇博客的时候还不知道C++的具体语法(emmmmmm以后肯定会),只是看到人家的程序里能够直接调用queue省时省力,而我只会一遍又一遍的写queue的子函数,太费劲。所以呢,出于偷懒
的目的,我总结一下偷懒
的常用途径。
队列的定义
- 队列是一种容器适配器,专门设计用于在FIFO上下文(先进先出)中操作,其中元素插入容器的一端并从另一端提取。 队列被实现为容器适配器,它是使用特定容器类的封装对象作为其基础容器的类,提供一组特定的成员函数来访问其元素。 元素被推入特定容器的“背部”并从其“前”弹出。
使用时必须表明的头文件
#include <iostream>
#include <queue>
#include <cstdio>
#include <cstdlib>
using namespace std;
常见用法(与c中循环队列进行对照,需要注意的是循环队列需要提前标注队列容量MaxSize)
queue<Type> Queue
—>定义一个queue变量typedef int Position;
typedef struct QNode *PtrToQNode;
struct QNode {
ElementType *Data;
Position Front, Rear;
int MaxSize;
};
typedef PtrToQNode Queue;
Queue CreateQueue(int MaxSize)
{
Queue Q = (Queue)malloc(sizeof(struct QNode));
Q->Data = (ElementTye *)malloc(MaxSize * sizeof(ElementType));
Q->Front = Q->Rear = 0;
Q->MaxSize = MaxSize;
}
Queue.empty()
—>判断队列是否为空,空返回1,非空返回0bool Isempty(Queue Q)
{
return (Q->Front == Q->Rear);
}
Queue.push()
—>入队,即在已有元素后面增加元素void AddQ(Queue Q,ElementType X)
{
Q->Rear = (Q->Rear + 1) % Q->MaxSize;
Q->Data[Q->Rear] = X;
}
Queue.size()
—>输出现有队列的长度
- 循环队列可以通过记录
cnt
来输出现有队列的长度,即每入队一次cnt++
;
Queue.front()
—>显示队列第一个元素,但不出队列,即为Q->Data[Front]
;
Queue.back()
—>显示最后一个元素。在循环队列中,即为Q->Data[Rear]
;
Queue.pop()
—>出队列,不显示出队列的元素void DeleteQ(Queue Q)
{
Q->Front = (Q->Front + 1) % Q->MaxSize;
}
对照示例程序
#include <iostream>
#include <queue>
#include <cstdio>
#include <cstdlib>
using namespace std;
int main()
{
queue <int> myQ;
cout<< "现在 queue 是否 empty? "<< myQ.empty() << endl;
for(int i =0; i<10 ; i++)
{
myQ.push(i);
}
for(int i=0; i<10; i++)
{
printf("myQ.size():%d\n",myQ.size());
cout << myQ.front()<<endl;
myQ.pop();
}
return 0;
}
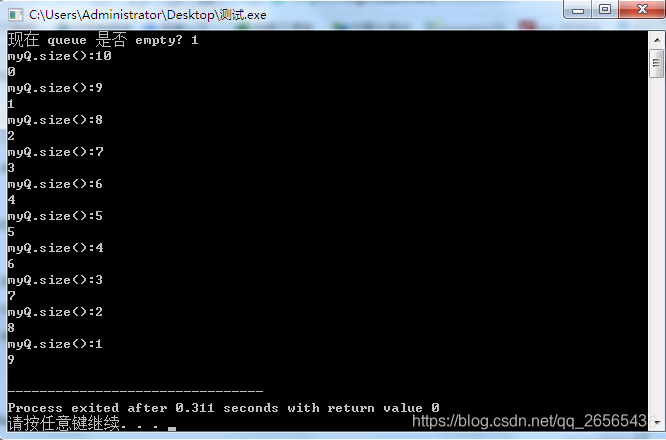
#include <stdio.h>
#include <stdlib.h>
#define ElementType int
typedef int Position;
typedef struct QNode *PtrToQNode;
struct QNode {
ElementType *Data;
Position front, rear;
int MaxSize;
};
typedef PtrToQNode Queue;
Queue CreateQ(int MaxSize)
{
Queue Q = (Queue)malloc(sizeof(struct QNode));
Q->Data = (ElementType *)malloc(MaxSize*sizeof(ElementType));
Q->front = Q->rear = 0;
Q->MaxSize = MaxSize;
}
void AddQ(Queue Q, ElementType V)
{
Q->rear = (Q->rear + 1)%Q->MaxSize ;
Q->Data[Q->rear ] = V;
}
ElementType DeleteQ(Queue Q)
{
Q->front = (Q->front +1)%Q->MaxSize;
return Q->Data[Q->front ];
}
int IsEmpty(Queue Q)
{
return (Q->rear ==Q->front );
}
int main()
{
Queue Q;
int MaxSize = 10;
int cnt = 0;
Q = CreateQ(MaxSize);
printf("现在 Q 是否 empty? %d\n", IsEmpty(Q) );
for (int i = 0; i < 10; i++){
AddQ(Q, i);
cnt++;
}
for (int i = 0; i < 10; i++){
printf("Q的长度:%d\n", cnt);
printf("%d\n", DeleteQ(Q));
cnt--;
}
return 0;
}
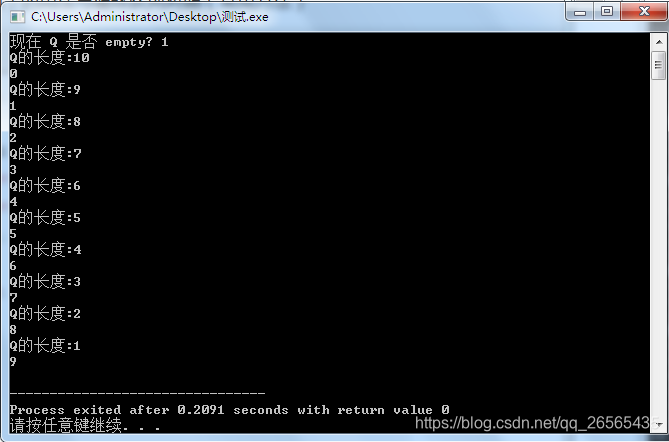