104. Maximum Depth of Binary Tree
求二叉树最大深度
3 / \ 9 20 / \ 15 7
return its depth = 3.
class Solution { public int maxDepth(TreeNode root) { if(root ==null) return 0; int h_l = maxDepth(root.left)+1; int h_r = maxDepth(root.right)+1; return Math.max(h_l,h_r); } }
559. Maximum Depth of N-ary Tree
一开始写成这样, 结果怎么都不对:
因为 这里 maxDepth(child) 根本不会 hit root ==null 的情况,因为如果没有叶子节点, children 直接为空, 不会进行递归。
class Solution { public int maxDepth(Node root) { if(root ==null) return 0; int max = Integer.MIN_VALUE; //不能写成 这样 for(Node child: root.children){ max = Math.max(max, maxDepth(child)); } return max+1; } }
为了便于理解,写成这样, 注意 helper里 不需要 if(root ==null) return 0 的判断。
class Solution { public int maxDepth(Node root) { if(root ==null) return 0; return helper(root); } int helper(Node node){ int max = 0; for(Node child: node.children){ max = Math.max(max, maxDepth(child)); } return max+1; } }
111. Minimum Depth of Binary Tree 求最小深度
一开始直接无脑写成这样,直接就WA掉了。
扫描二维码关注公众号,回复:
3983798 查看本文章
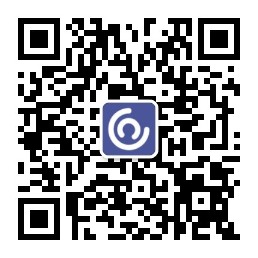
class Solution { public int minDepth(TreeNode root) { if(root ==null) return 0; return Math.min(minDepth(root.left), minDepth(root.right)) +1; } }
注意, 最小深度时,如果 某个节点没有某个子节点 不计算在内, 例如 1: 2, null , 最小深度为2,用过用上面的算法 返回为1了。
正确写法:
class Solution { public int minDepth(TreeNode root) { if(root ==null) return 0; int left = minDepth(root.left); int right = minDepth(root.right); return (left==0 || right==0)? left+right+1: Math.min(left,right)+1; } }
BFS 写法:
class Solution { public int minDepth(TreeNode root) { if(root == null) return 0; Queue<TreeNode> queue = new LinkedList<>(); queue.add(root); int level = 0; while(!queue.isEmpty()){ TreeNode node; int size = queue.size(); level++; for(int i = 0; i<size; i++){ node = queue.remove(); if(node.left==null && node.right==null) {return level;} //说明已经是最小 直接返回 if(node.left!=null) queue.add(node.left); if(node.right!=null) queue.add(node.right); } } return level; } }
110. Balanced Binary Tree
左右子树深度差不超过1的树称为 平衡二叉树,如果是空数的话也是平衡二叉树。
算法: DFS求左右子数深度,求解过程中判断深度差。
算法一: 递归过程中加一个flag, 只要有不满足条件的 flag = false,但这样的写法需要额外定义flag, code 写的不够漂亮。
class Solution { public boolean isBalanced(TreeNode root) { boolean[] flag = new boolean[1]; flag[0] = true; depth(root,flag); return flag[0]; } private int depth(TreeNode root, boolean[] flag){ if(root == null) return 0; int depth_left = 1+ depth(root.left,flag); int depth_right = 1+ depth(root.right,flag); //System.out.println(depth_left+ " "+depth_right); if(Math.abs(depth_left -depth_right) >1) { flag[0] = false; return -1; } return Math.max(depth_left,depth_right); } }
改进算法: 出现非对称的子数就返回-1, 并且后面都返回-1.
class Solution { public boolean isBalanced(TreeNode root) { return depth(root) >= 0; } private int depth(TreeNode root){ if(root == null) return 0; int depth_left = depth(root.left); int depth_right = depth(root.right); if(Math.abs(depth_left -depth_right) >1) { return -1; } return (depth_left== -1 || depth_right == -1) ? -1: Math.max(depth_left,depth_right)+1; } }