linkqueue.h
#ifndef __LINKQUEUE_H__
#define __LINKQUEUE_H__
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
typedef int datatype;
typedef struct linkqueue_node{
datatype data;
struct linkqueue_node *next;
}queuenode,*p_queuenode;
typedef struct node{
p_queuenode front,rear;
}linkqueue,*p_linkqueue;
extern bool create_linkqueue(p_linkqueue *H);
extern bool is_empty_linkqueue(p_linkqueue H);
extern bool in_linkqueue(p_linkqueue H,datatype x);
extern bool out_linkqueue(p_linkqueue H,datatype *x);
extern void show_linkqueue(p_linkqueue H);
#endif
linkqueue.c
#include "linkqueue.h"
bool create_linkqueue(p_linkqueue *H)
{
if(((*H) = (p_linkqueue)malloc(sizeof(linkqueue))) == NULL)
{
printf("malloc no memory!\n");
return false;
}
if(((*H)->front = (p_queuenode)malloc(sizeof(queuenode))) == NULL)
{
printf("malloc no memory!\n");
return false;
}
(*H)->front->next = NULL;
(*H)->rear = (*H)->front;
return true;
}
bool is_empty_linkqueue(p_linkqueue H)
{
return (H->rear == H->front);
}
bool in_linkqueue(p_linkqueue H,datatype x)
{
p_queuenode K;
if((K = (p_queuenode)malloc(sizeof(queuenode))) == NULL)
{
printf("malloc no memory!\n");
return false;
}
K->data = x;
K->next = H->rear->next;
H->rear->next = K;
H->rear = K;
return true;
}
bool out_linkqueue(p_linkqueue H,datatype *x)
{
p_queuenode r;
if(is_empty_linkqueue(H))
{
printf("queue is NULL!\n");
return false;
}
r = H->front;
H->front = r->next;
(*x) = H->front->data;
r->next = NULL;
free(r);
r = NULL;
return true;
}
void show_linkqueue(p_linkqueue H)
{
p_queuenode r;
if(is_empty_linkqueue(H))
{
printf("queue is NULL!\n");
return ;
}
r = H->front;
while(r != H->rear)
{
r = r->next;
printf("%d\t",r->data);
}
puts("");
}
main.c
#include "linkqueue.h"
int main(int argc, const char *argv[])
{
datatype x;
p_linkqueue H;
create_linkqueue(&H);
show_linkqueue(H);
while(1)
{
printf("Please input(number in <--->character out)[-1 exit:");
if(scanf("%d",&x))
{
if(x == -1)
{
break;
}
in_linkqueue(H,x);
show_linkqueue(H);
}
else
{
getchar();
out_linkqueue(H,&x);
printf("out:%d\n",x);
show_linkqueue(H);
}
}
show_linkqueue(H);
return 0;
}
运行结果
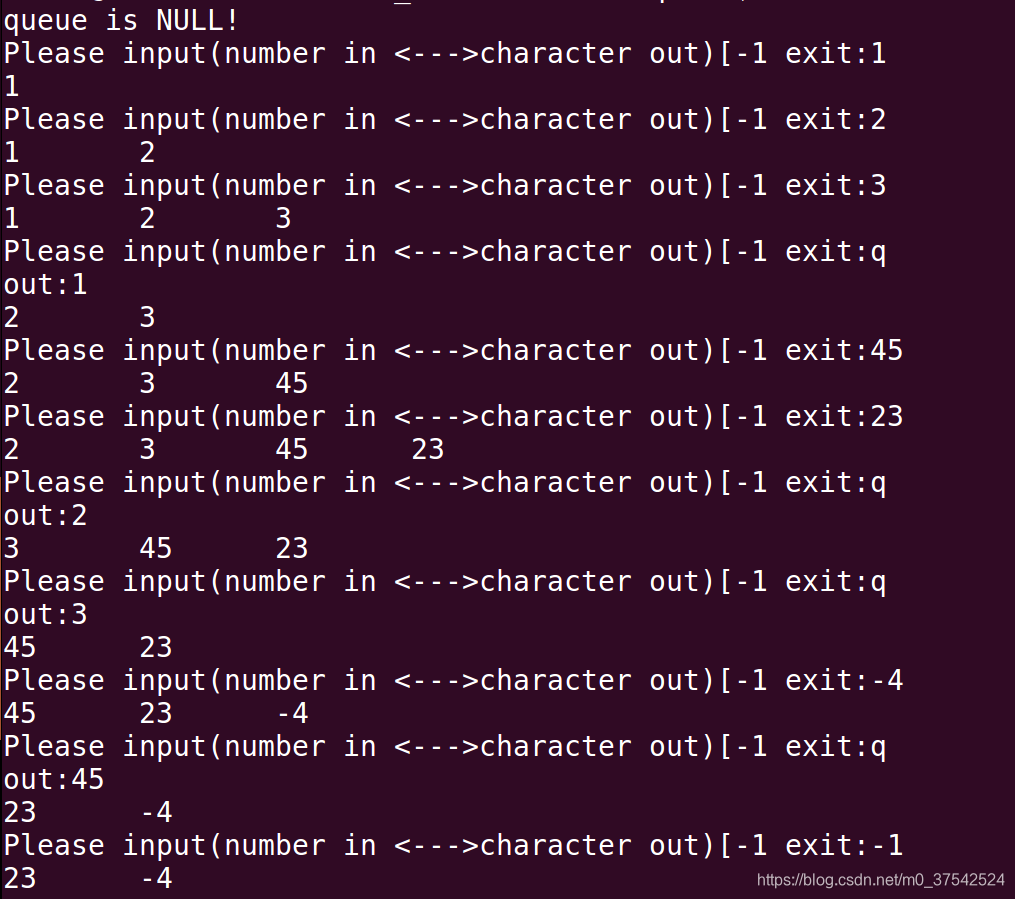