v-for
data为object,例如:
{
name:"1111",
age:12
}
,那么使用v-for(value in object),输出会是"1111",12,v-for(key in object),输出会是"name","age"
v-for可以循环渲染组件、数组和对象
组件:
首先新建a.vue文件,补上具体内容,
如果app.vue要用到它,所以在app.vue的<script>下一行写上:
import componentA from './a.vue'(此处文件是相对App.vue而言的)
然后export default {
components: {theA: componentA},data:......},
然后在App.vue的<template></template>放入上面的标签,引入<theA></theA>(本来规定必须是-写法,现在vue2.0驼峰写法也是可以了)
数组类型
直接设置data里面数组类型的值,比如:
list[2]=12或者list.length=100这样子是不会引起页面更新渲染的,
对于情况1,要这么写:
Vue.set(this.list,2,12);/Vue.$set(this.list,2,12);
或者 list.splice(2,1,12)
对于修改长度,要list.splice(100);
设置class:
方法一:
<p :class="classStr"></p>
data:{classStr:'red'}//为字符串 ---------<p class="red"></p>
data:{classStr:{'red':true,'blue':false}//为对象----------<p class="red"></p>
data:{classStr:['red','blue']}//为数组--------<p class="red blue"></p>
方法二:
<p :class="[classA,classB]"></p>
data:{classA:'red',classB:'blue'}
方法三:
:class=[classA,{'red':hasError}]
data:{classA:'hello',hasError:true}//<p class="hello red"></p>
方法四:
<p :style="linkCSS"></p>
data:{linkCss:{'color':'red','font-size':'12px'}}
v-if与v-show的区别:
v-if是直接增删dom,v-show是通过设置display属性
父子组件通信:
1.通过事件:
子组件:
<button @click="emitMyEvent">emit</button>
data () {
return {
hello: 'i am component a'
}
},
methods: {
emitMyEvent () {
this.$emit('my-event', this.hello)
}
}
父组件:(my-event格式不能为驼峰式)
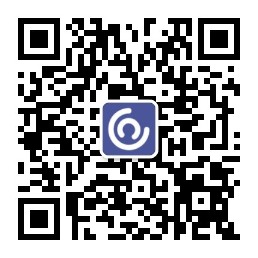
<componentA @my-event="deal"></componentA>
methods:{
deal (param) {
console.log(param)
}
}
2.父组件向子组件传值 :
父组件:(number格式不能为驼峰式)
<componentA :number="myval"></componentA>
data(){
myval:''
}
子组件:
<template>
{{number}}
</template>
props:['number']
props还可以写成:
props:{
'number':[Number,String]//指定number类型
}
3.slot
子组件:
<div>
<slot name="header">no header</slot>
<slot name="foooter">no footer</slot>
</div>
父组件:
<theA>
<p slot="header">xxx header</p>
<p slot="footer">xxx footer</p>
</theA>
这样子组件就会出现xxx header和xxx footer这样的显示
表单:
<input type="checkbox" v-model="myBox" value="apple1">
<input type="checkbox" v-model="myBox" value="apple2">
<input type="checkbox" v-model="myBox" value="apple3">
{{myBox}}
data(){myBox: []}
最终mybox的值会随着选择而变,例如选择apple1,apple2,那么myBox会变成:[ "apple1", "apple2" ]
<select v-model='sel'>
<option value="a">1</option>
<option value="b">2</option>
</select>
{{sel}}
data(){sel:''}
如果选了1,那么sel会变成a
vuex
main.js:
import Vue from 'vue'
import App from './App'
import Vuex from 'vuex'
Vue.use(Vuex) // 注册使用vuex
let store = new Vuex.Store({
state: {
totalPrice: 0
},
getters: {
getTotal (state) {
return state.totalPrice
}
},
mutation: {
increase (state, price) {
state.total += price
},
decrease (state, price) {
state.totalPrice -= price
}
},
actions: {
incment (context, price) {
API(id, function (price) {//此处意为从后台异步获取接口数据,mutation不能异步
context.commit('increase', price)//调用mutation的方法
})
}
}
})
/* eslint-disable no-new */
new Vue({
el: '#app',
router,
store,
render: h => h(App)
})
App.vue:
<template>
{{totalPrice}}
<theA></theA>
<theB></theB>
</div>
</template>
<script>
import componentA from './A.vue'
import componentB from './b.vue'
export default {
components: {theA: componentA, theB: componentB},
data () {
return {}
},
computed: {
totalPrice () {
return this.$store.getters.getTotal
}
}
}
</script>
<style>
</style>
A.vue:
<template>
<div>
<button @click="addOne">+1</button>
<button @click="delOne">+1</button>
</div>
</template>
<script>
export default{
data () {
return {
price: 5
}
},
methods: {
addOne () {
this.$store.increment('increase', this.price)//这里是dispatch了action--increment
},
delOne () {
this.$store.commit('decrease', this.price)
}
}
}
</script>
b.vue:
<template>
<div>
<button @click="addOne">+1</button>
<button @click="delOne">+1</button>
</div>
</template>
<script>
export default{
data () {
return {
price: 15
}
},
methods: {
addOne () {
this.$store.commit('increase', this.price)
},
delOne () {
this.$store.commit('decrease', this.price)
}
}
}
</script>
如此一来,达成的效果就是点击App.vue组件里A,b组件对应的按钮,App.vue组件里显示的totalPrice的值会随着点击而改变(+5或+15)