spring框架 - IOC
-
一、MVC概念
1. Model : 数据模型
MVC 2. View : 视图
3. Controller : 控制器
-
二、框架概念
页面 -> 发请求 -> 后台(C -> S -> D) -JDBC-> 数据库 DB
数据库访问 |
JDBC Mybatis Hibernate |
bean管理 |
spring框架 |
MVC框架 |
springMVC structs |
-
三、spring名词
IOC:控制反转(Inversion of Control)
具有依赖注入功能的,可以创建实例的容器。IOC容器负责实例化、定位、配置应用程序中的对象及建立这些对象间的依赖。通常new一个实例,控制权由程序员控制,而"控制反转"是指new实例工作不由程序员来做而是交给Spring容器来做。。在Spring中BeanFactory是IOC容器的实际代表者。
DI:依赖注入(dependency injection)
在容器创建对象后,处理对象的依赖关系。
AOP:面向切面
提供面向切面的编程,可以给某一层提供事务管理,例如在Service层添加事物控制 。
-
四、搭建 spring - maven 环境
1. 创建一个maven的普通工程 quickstart
2. 引用pom.xml依赖
<!-- spring 框架核心坐标添加 -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>4.3.9.RELEASE</version>
</dependency>
3. 创建一个bean, HelloService
package com.lwx.service;
/**
* By :刘文旭 ~ https://blog.csdn.net/qq_40414738
* Date:2018/10/29 12:02 .
*/
public class HelloService {
public void Hello(String lwx) {
System.out.println("Hello Spring!!!");
}
}
4. 创建一个spring01.xml配置文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!--
xmlns 即xml namespace xml 使用的命名空间
xmlns:xsi 即xml schema instance xml 遵守的具体规范
xsi:schemaLocation 本文档xml 遵守的规范官方指定
-->
<bean id="helloService" class="com.lwx.service.HelloService"></bean>
</beans>
5. 单元测试 junit @Test
package com.lwx.service;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class SpringTest {
@Test
public void test01(){
/**
* 1.加载配置文件
* 2.获取bean对象
* 3.执行bean方法
*/
ApplicationContext context = new ClassPathXmlApplicationContext("spring01.xml");
HelloService helloService = (HelloService) context.getBean("helloService");
helloService.Hello("lwx");
}
}
-
五、手写IOC容器
知识点:xml解析 xpath使用 dom4j 反射 集合
功能:
1. 读取xml配置文件
2. 通过配置信息创建类的实例
3. 存储实例以及取出实例
步骤:
1.xml 解析dom4j 坐标依赖
2.Bean 工厂接口定义
3.实现类
package com.lwx;
import org.dom4j.Document;
import org.dom4j.DocumentException;
import org.dom4j.Element;
import org.dom4j.io.SAXReader;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* By:刘文旭 ~ https://blog.csdn.net/qq_40414738
* Date:2018/10/29 15:02 .
*/
@SuppressWarnings("all")
public class LwxApplicationContext implements ApplicationContext {
/**
* 1.读取配置文件
* @param id
* @return
*/
private String path; //xml配置路径
private List<LwxBean> beanList = new ArrayList<>(); // 存放bean配置信息
private Map<String, Object> beanMap = new HashMap<>(); //存放bean实例以及反射
public LwxApplicationContext(String path) {
this.path = path;
// xml解析
parseXml(path);
// 创建bean实例
creatBean();
}
/**
* 2.创建bean实例
*/
private void creatBean() {
/**
* 循环beanList,生成bean
*/
if (beanList != null && beanList.size() > 0) {
for (LwxBean lwxBean : beanList) {
try {
beanMap.put(lwxBean.getId(), Class.forName(lwxBean.getClazz()).newInstance());
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
}
/**
* 1.解析xml
* @param path
*/
private void parseXml(String path) {
SAXReader reader = new SAXReader();
if (null != path && !"".equals(path)) {
try {
URL url = this.getClass().getClassLoader().getResource(path);
Document document = reader.read(url);
List<Element> list = document.selectNodes("//beans/bean");
for (Element element : list) {
System.out.println(element.attributeValue("id") + "-" + element.attributeValue("class"));
LwxBean lwxBean = new LwxBean();
lwxBean.setId(element.attributeValue("id"));
lwxBean.setClazz(element.attributeValue("class"));
beanList.add(lwxBean);
}
} catch (DocumentException e) {
e.printStackTrace();
}
} else {
System.out.println("文件不存在!");
}
}
@Override
public Object getBean(String id) {
return beanMap.get(id);
}
}
4.Bean 属性对象定义
理解: Spring 容器在启动的时候读取xml 配置信息,并对配置的bean 进行实例化(这里模拟的比较简单,仅用于帮助大家理解),同时通过上下文对象提供的getbean 方法拿到我们配置的bean 对象,从而实现外部容器自动化维护并创建bean 的效果。
-
六、多文件加载
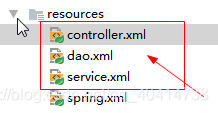
ApplicationContext context=
new ClassPathXmlApplicationContext("dao.xml","service.xml","controller.xml");
-
七、实例化bean的三种方式
1.构造器方式(常用)
通过空构造实例化,没有就会报错!!!
2.静态工厂
控制bean创建的初始化行为
相比于上一种方法,静态工厂可以在实例化bean时进行一些初始化操作。
|
3.实力工厂
整合多个框架bean依赖
|
|
|
|