版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/Houheshuai/article/details/50480634
我们创建三个任务与三个线程,让三个线程启动,同时执行三个任务。
任务类必须实现 Runable 接口,而 Runable 接口只包含一个 run 方法。需要实现
这个方法来告诉系统线程将如何运行。
创建任务:
TaskClass task = new TaskClass(...);
任务必须在线程中执行,创建线程:
Thread thread = new Thread(task);
然后调用 start 方法告诉 Java 虚拟机该线程准备运行,如下:
thread.start();
下面的程序创建三个任务和三个运行这些任务的线程:
任务1 打印字母 a 10次
扫描二维码关注公众号,回复:
3816396 查看本文章
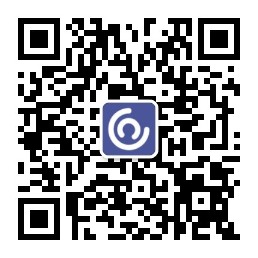
任务2 打印字母 b 10次
任务3 打印 1 ~ 10 的整数
运行这个程序,则三个线程共享CPU,在控制台上轮流打印字母和数字。
代码:
public class TaskDemo {
public static void main(String[] args) {
//创建三个任务
Runnable printA = new PrintChar('a', 10);
Runnable printB = new PrintChar('b', 10);
Runnable print100 = new PrintNum(10);
//创建三个线程
Thread thread1 = new Thread(printA);
Thread thread2 = new Thread(printB);
Thread thread3 = new Thread(print100);
//启动三个线程,将同时执行创建的三个任务
thread1.start();
thread2.start();
thread3.start();
}
}
//此任务将一个字符打印指定次数
class PrintChar implements Runnable {
private char charToPrint;
private int times;
//构造函数
public PrintChar(char charToPrint, int times) {
this.charToPrint = charToPrint;
this.times = times;
}
//重写接口Runable中的run方法,告诉系统此任务的内容
@Override
public void run() {
for (int i = 0; i < times; i++) {
System.out.print(charToPrint);
}
}
}
//此任务打印1~n
class PrintNum implements Runnable {
private int lastNum;
public PrintNum(int lastNum) {
this.lastNum = lastNum;
}
@Override
public void run() {
for (int i = 0; i < lastNum; i++) {
System.out.print(" " + i);
}
}
}
结果:
注意:每次运行的结果都不相同,因为我们并不知道三个任务的执行顺序。